How to Display SVG Images in Android Jetpack Compose
SVG, Scalable Vector Graphics, is a type of image format that uses vector graphics to define the shapes and colors of an image. Unlike raster images such as JPEG or PNG, SVG images can be resized without losing quality, making them a great choice for use in mobile apps.
In this blog post, let’s check how to show SVG images in Jetpack Compose.
Android doesn’t support SVG by default. Hence, we are using the Coil library for the SVG support. Add the following code to the dependency tag in build.gradle app module file.
implementation("io.coil-kt:coil-compose:2.2.2")
implementation("io.coil-kt:coil-svg:2.2.2")
Import a SVG image into drawable and then you can show svg images using coil as given below.
val imageLoader = ImageLoader.Builder(LocalContext.current)
.components {
add(SvgDecoder.Factory())
}
.build()
Image(
painter = rememberAsyncImagePainter(R.drawable.mushroom, imageLoader),
contentDescription = null,
modifier = Modifier.fillMaxSize()
)
You will get the following output.
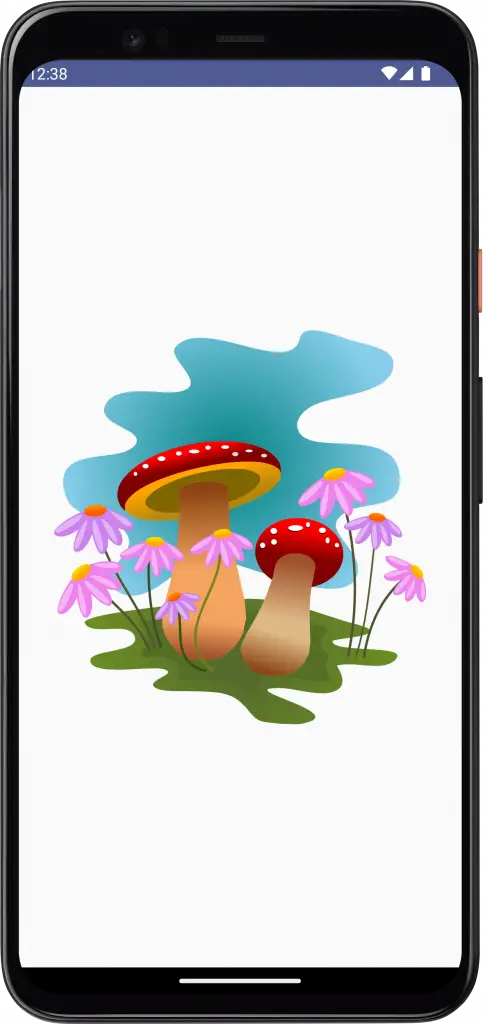
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.foundation.Image
import coil.ImageLoader
import coil.compose.rememberAsyncImagePainter
import coil.decode.SvgDecoder
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
val imageLoader = ImageLoader.Builder(LocalContext.current)
.components {
add(SvgDecoder.Factory())
}
.build()
Image(
painter = rememberAsyncImagePainter(R.drawable.mushroom, imageLoader),
contentDescription = null,
modifier = Modifier.fillMaxSize()
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
That’s how you show SVG images in Jetpack Compose.
With Coil, you can also show gif images in Jetpack Compose.