How to Use Border Modifier in Android Jetpack Compose
Creating a user interface that is not only functional but also visually appealing is crucial in developing a quality Android application. In Jetpack Compose, modifiers play a significant role in adding and altering visual characteristics of composables.
In this blog post, we’re going to focus on how to add borders to your composables using the border modifier.
The border modifier allows you to add a border around any composable that can accept modifiers. Here is a simple example:
@Composable
fun ModifierExample() {
Column {
Box(
modifier = Modifier
.size(100.dp)
.border(2.dp, Color.Red)
)
}
}
In this example, the border modifier is used to add a red border of 2.dp thickness around the Box composable.
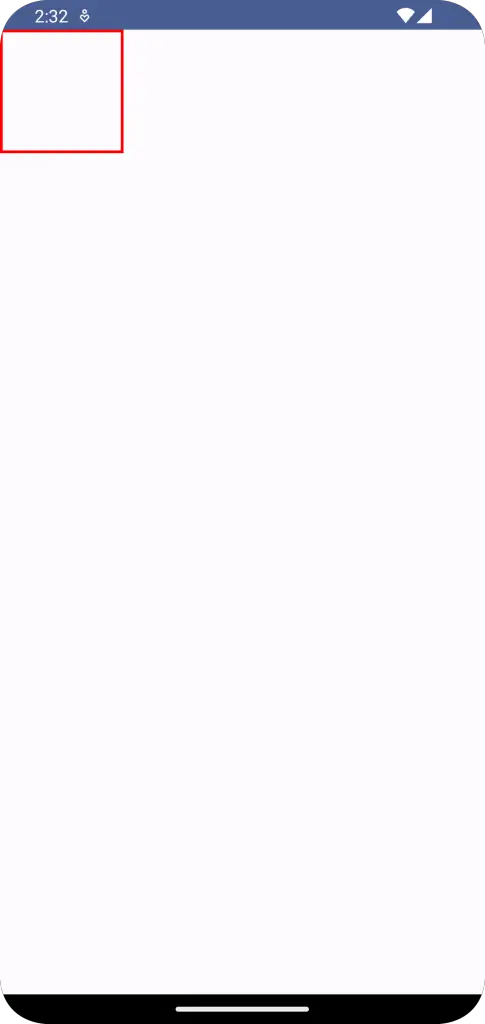
Now, let’s try adding a border to a Text composable.
@Composable
fun ModifierExample() {
Column {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.border(2.dp, Color.Red, shape = RoundedCornerShape(10.dp))
)
}
}
In this case, we’ve added an additional shape parameter to the border modifier to make the corners of the border rounded. Jetpack Compose allows you to specify the shape of your border. RoundedCornerShape is just one of the shapes you can use.
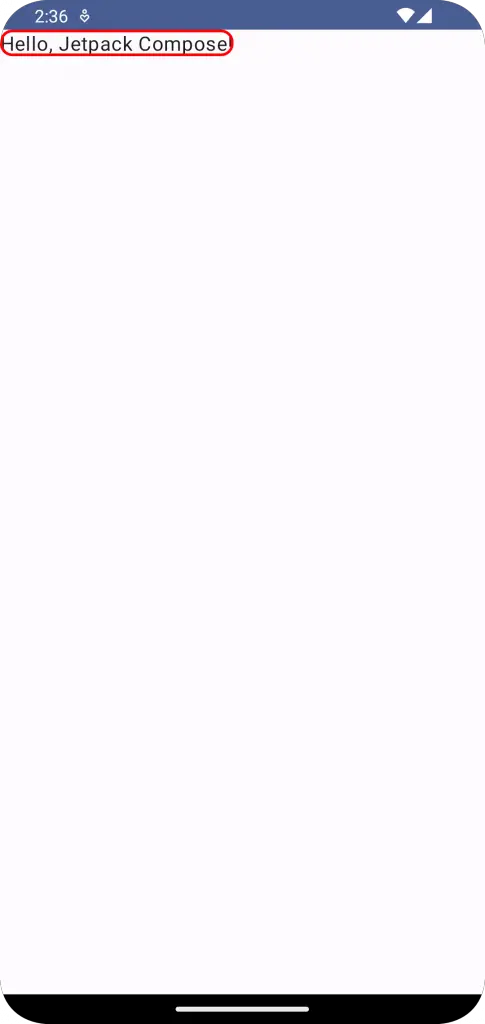
In Jetpack Compose, the border modifier is quite flexible. You can set the color, thickness, and shape of your border. You can even use gradients for your border color. Here’s how you can do it:
Box(
modifier = Modifier
.size(100.dp)
.border(
width = 5.dp,
brush = Brush.horizontalGradient(
colors = listOf(Color.Red, Color.Blue)
),
shape = CircleShape
)
)
In this example, the brush parameter of the border modifier is used to set a horizontal gradient as the border color. You can also use verticalGradient or radialGradient based on your design needs.
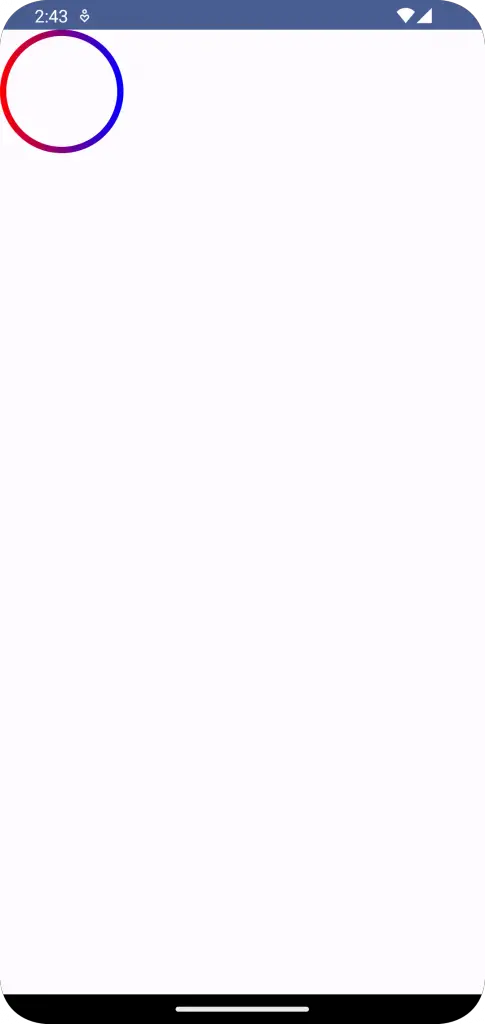
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.border
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.size
import androidx.compose.foundation.shape.CircleShape
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ModifierExample()
}
}
}
}
}
@Composable
fun ModifierExample() {
Column {
Box(
modifier = Modifier
.size(100.dp)
.border(
width = 5.dp,
brush = Brush.horizontalGradient(
colors = listOf(Color.Red, Color.Blue)
),
shape = CircleShape
)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
ModifierExample()
}
}
That’s it! You now know how to add borders to your composables in Jetpack Compose. Start experimenting with different shapes, colors, and widths to enhance the visual appeal of your app.