How to Disable Slider in Jetpack Compose
As Android apps become more complex, developers need ways to dynamically enable and disable UI components. Disabling parts of the interface allows you to streamline user interactions by only presenting relevant options.
Sliders are a prime target for disabling. By greying out sliders when they don’t apply, you guide users and simplify layouts. In this blog post, we’ll explore techniques for disabling sliders in Jetpack Compose.
When to Disable a Slider
First, let’s discuss common reasons for disabling a slider:
- The slider’s value doesn’t currently affect app behavior
- The user lacks permission to change the value
- Dependent settings haven’t been configured
- The app state prevents interaction
Disabling unused or inaccessible sliders reduces clutter and distracting elements. Just be sure to re-enable sliders when the context changes.
Disable Slider Interaction
Jetpack Compose makes it easy to disable sliders. Here’s an example:
@Composable
fun MySlider() {
var sliderValue by remember { mutableStateOf(0f) }
Slider(
value = sliderValue,
onValueChange = { sliderValue = it },
valueRange = 0f..100f,
enabled = false
)
}
The enabled
parameter disables interaction when set to false
. This greys out the slider and prevents value changes.
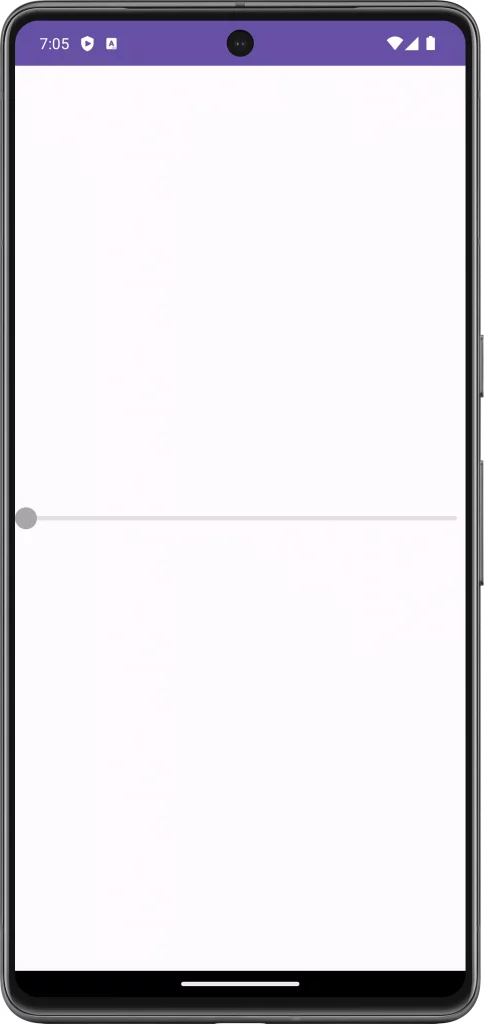
Customize Disabled Appearance
Don’t forget the visual design when disabling sliders. Use SliderDefaults
to customize colors for the disabled state:
@Composable
fun MySlider() {
var sliderValue by remember { mutableStateOf(0f) }
Slider(
value = sliderValue,
onValueChange = { sliderValue = it },
valueRange = 0f..100f,
enabled = false,
colors = SliderDefaults.colors( disabledThumbColor = Color.DarkGray,
disabledInactiveTrackColor = Color.Gray)
)
}
Pick muted shades that match your theme. Just ensure the disabled slider remains visible rather than blending into the background.
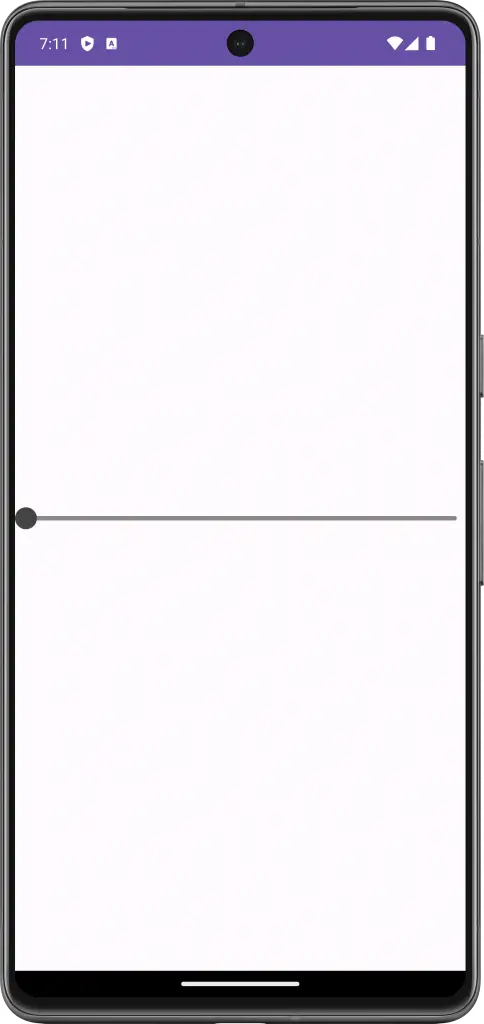
Best Practices
When disabling sliders, keep these guidelines in mind:
- Clearly visually distinguish disabled state
- Use icons/text to indicate why the slider is disabled
- Retain slider order/layout for consistent experience
- Re-enable once interactions become valid
With mindful design, you can build intuitive flows using disabled sliders.
The ability to disable components grants control over interactivity. By disabling sliders in Jetpack Compose, you discourage invalid interactions and streamline user options.
Combine with other dynamic layout techniques to build adaptive UIs. Just remember to provide clear visual feedback when disabling features users might expect. What strategies have you found for streamlining app interactions? Let me know in the comments!