How to Create Slider with Label in Jetpack Compose
In the realm of Android development, Jetpack Compose is revolutionizing how we build user interfaces. One key component in many apps is the Slider
, often used for settings like volume control or brightness adjustment.
Enhancing a Slider
with a label not only improves functionality but also enhances user experience. This blog post will guide you through creating a Slider
with a label in Jetpack Compose.
Slider in Jetpack Compose
A Slider
in Jetpack Compose is a versatile component that allows users to select a value from a continuous or discrete range. Adding a label to a Slider
provides context, making it more intuitive for users to interact with.
Implement a Slider with a Label
Creating a slider with a label in Jetpack Compose involves combining the Slider
, Text
and Column composables within a layout. Here’s an example implementation:
@Composable
fun MySlider() {
var sliderValue by remember { mutableStateOf(0f) }
Column(modifier = Modifier.padding(10.dp)) {
Text("Volume")
Slider(
value = sliderValue,
onValueChange = { sliderValue = it },
valueRange = 0f..100f
)
}
}
This code snippet defines a MySlider
composable function that displays a slider with a label. The Column
composable organizes the label and the slider vertically. The Text
composable displays the label “Volume”, and the Slider
composable allows users to select a value from 0 to 100.
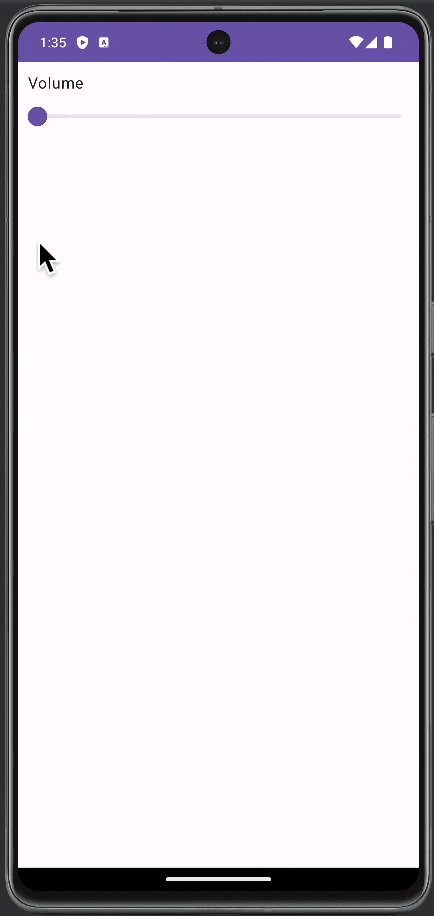
Style the Label
Customize the label using Text
composable properties like fontSize
, fontWeight
, and color
to match your app’s design language.
Customize Slider Properties
Jetpack Compose allows you to customize the Slider
‘s colors and thumb size using the sliderColors
and thumbSize
parameters.
Best Practices
User Experience
Ensure the label clearly describes the purpose of the Slider
. This is crucial for accessibility and usability.
Consistency
Maintain a consistent look and feel with other UI elements in your app. This includes using similar fonts, colors, and spacing.
Accessibility
Consider adding accessibility features like contentDescription
for the visually impaired. Testing with accessibility tools like TalkBack is also recommended.
Adding a label to a Slider
in Jetpack Compose enhances the user’s understanding of what the slider controls, thereby improving the overall user experience. With customization options, you can ensure that the slider fits seamlessly into your app’s design, making it both functional and aesthetically pleasing.
3 Comments