How to Show Snackbar without Scaffold in Android Jetpack Compose
A Snackbar is an excellent way to provide feedback to the users about an operation’s status. It’s a temporary UI element used for displaying brief, auto-expiring (dismissible) notifications to the user. Jetpack Compose makes it easier than ever to create and customize snackbars in your Android apps.
While the Scaffold is a popular choice for displaying Snackbar in Compose, it isn’t the only way. In this post, we’ll learn how to create a Snackbar without using a Scaffold.
Snackbar without Scaffold
First, we need to create a SnackbarHostState. This is used to control and observe the current Snackbar.
val snackbarHostState = remember { SnackbarHostState() }
Next, we’ll create a SnackbarHost which will show our Snackbar when requested.
SnackbarHost(
hostState = snackbarHostState,
modifier = Modifier.align(Alignment.BottomCenter)
)
Next, we need to trigger the Snackbar. We’ll use a Button for this example:
Button(onClick = {
scope.launch {
val result = snackbarHostState.showSnackbar(
message = "Snackbar Example",
actionLabel = "Action",
withDismissAction = true,
duration = SnackbarDuration.Indefinite
)
when (result) {
SnackbarResult.ActionPerformed -> {
//Do Something
}
SnackbarResult.Dismissed -> {
//Do Something
}
}
}},
modifier = Modifier
.fillMaxSize()
.wrapContentSize()) {
Text(text = "Show Snackbar")
}
In the onClick function of the Button, we’re using a CoroutineScope to call the showSnackbar function of our SnackbarHostState. Now, let’s see the full example.
@Composable
fun SnackbarExample() {
val snackbarHostState = remember { SnackbarHostState() }
val scope = rememberCoroutineScope()
Box {
Button(onClick = {
scope.launch {
val result = snackbarHostState.showSnackbar(
message = "Snackbar Example",
actionLabel = "Action",
withDismissAction = true,
duration = SnackbarDuration.Indefinite
)
when (result) {
SnackbarResult.ActionPerformed -> {
//Do Something
}
SnackbarResult.Dismissed -> {
//Do Something
}
}
}},
modifier = Modifier
.fillMaxSize()
.wrapContentSize()) {
Text(text = "Show Snackbar")
}
SnackbarHost(
hostState = snackbarHostState,
modifier = Modifier.align(Alignment.BottomCenter)
)
}
}
In this Composable function, we’re using a Box layout to position our Button and SnackbarHost. The Button is in the center of the Box, and the SnackbarHost is aligned to the bottom center.
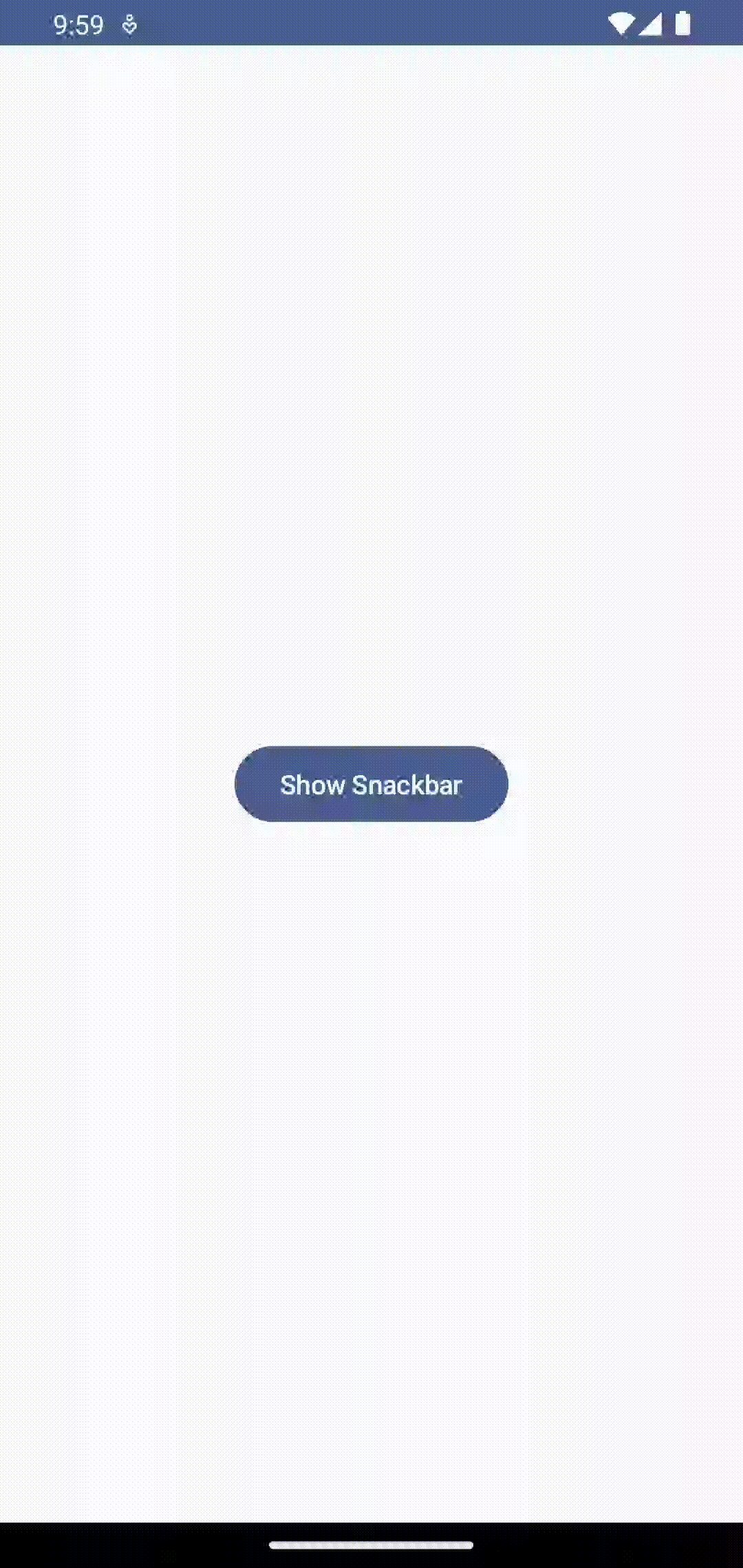
In this tutorial, we explored how to use a Snackbar in Jetpack Compose without relying on a Scaffold. While Scaffold makes it easy to use Snackbar, understanding how to use a Snackbar without it can help you create more flexible and customized UIs.
One Comment