How to make Text Selectable in Android Jetpack Compose
The text selection is a useful feature that allows users to highlight and copy text from a document, webpage, or other sources. In this blog post, let’s learn how to make text selectable in Jetpack Compose.
To start with, we’ll define a Composable function that displays a block of text using the Text component. By default, text in a Text composable is not selectable, which means that users can’t highlight or copy it.
You can make the text selectable by wrapping the text with SelectionContainer. See the code snippet given below.
@Composable
fun SelectableText() {
SelectionContainer {
Text("This is a selectable text")
}
}
The SelectionContainer component provides a way to make its children selectable, which means that the user can highlight and copy the text. It also manages the state of the text selection, including the start and end indices of the selected text.
By wrapping the Text component in a SelectionContainer, we’re making the text selectable without having to manually manage the state of the selection. The SelectionContainer automatically tracks the state of the selection and provides callbacks for handling changes to the selection.
You will get the following output.
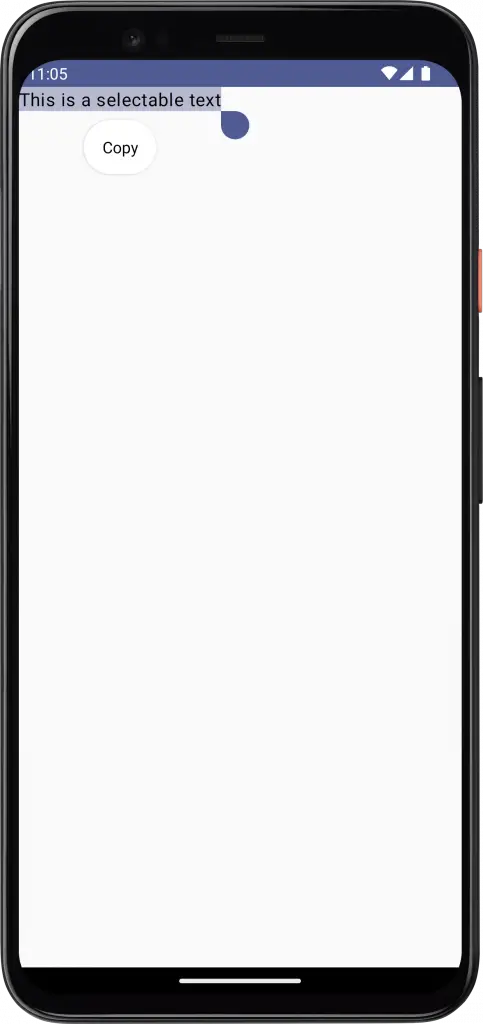
With SelectionContainer you can even make the indirect children selectable. See the code snippet given below.
@Composable
fun SelectableText() {
SelectionContainer {
Column {
Text("Text 1")
Text("Text 2")
Text("Text 3")
}
}
}
You will get the following output.
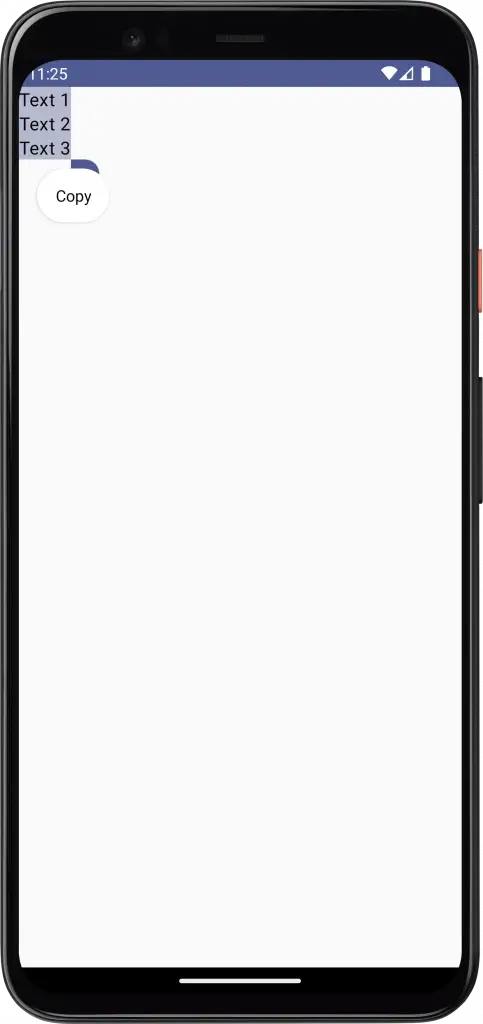
Sometimes, you may want different colors for the selected area and the handle. You can use custom colors as given below.
@Composable
fun SelectableText() {
val customTextSelectionColors = TextSelectionColors(
handleColor = Color.Green,
backgroundColor = Color.Yellow
)
CompositionLocalProvider(LocalTextSelectionColors provides customTextSelectionColors) {
SelectionContainer {
Column {
Text("This is a selectable text")
}
}
}
}
The interesting part of this code is the use of CompositionLocalProvider. The CompositionLocalProvider allows you to define a value for a CompositionLocal object within the current composition tree.
The LocalTextSelectionColors object is a composition local that defines the color scheme for text selection. By providing a custom TextSelectionColors class to the CompositionLocalProvider, we are overriding the default colors used for text selection within the SelectionContainer and its children.
In this case, the TextSelectionColors object provides a custom color scheme with a green handle color and a yellow background color for the text selection.
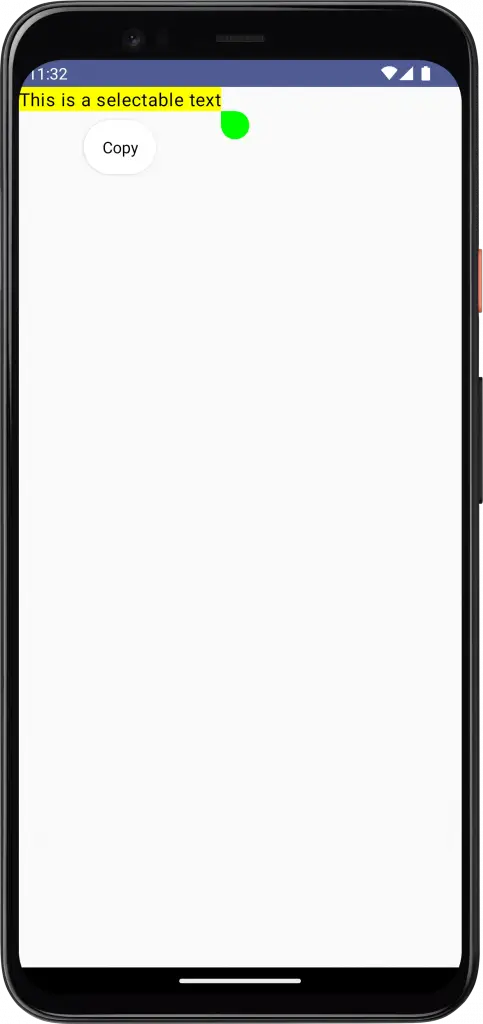
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.text.selection.LocalTextSelectionColors
import androidx.compose.foundation.text.selection.SelectionContainer
import androidx.compose.foundation.text.selection.TextSelectionColors
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
SelectableText()
}
}
}
}
}
@Composable
fun SelectableText() {
val customTextSelectionColors = TextSelectionColors(
handleColor = Color.Green,
backgroundColor = Color.Yellow
)
CompositionLocalProvider(LocalTextSelectionColors provides customTextSelectionColors) {
SelectionContainer {
Column {
Text("This is a selectable text")
}
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
SelectableText()
}
}
That’s how you make the text selectable and copyable in Jetpack Compose. I hope this tutorial is helpful for you.
One Comment