How to Add Multiple Color Styles to Single Text in Android Jetpack Compose
Styling text is a fundamental part of any mobile app development. In Android Jetpack Compose, the process of styling text is done through Composable functions. In this blog post, let’s learn how to apply multiple colors to single Text in Jetpack Compose.
The default Text composable function in Jetpack Compose doesn’t allow us to add multiple styles to a single text string. To add multiple color styles to a single text in Jetpack Compose, we can use the buildAnnotatedString function.
The buildAnnotatedString function allows us to create a string with multiple style spans. Instead of TextStyle use either SpanStyle or ParagraghStyle. See the code snippet given below.
@Composable
fun MultipleColorsInText() {
Text( text =
buildAnnotatedString {
withStyle(style = SpanStyle(color = Color.Red)) {
append("Coding ")
}
append("with ")
withStyle(style = SpanStyle(color = Color.Blue)) {
append("Rashid")
}
},
fontSize = 30.sp
)
}
The function MultipleColorsInText() takes no parameters and returns a Composable function that displays a Text string with multiple colors.
The Text is used to display the text on the screen. It takes a text parameter, which is a string, and the fontSize parameter.
The buildAnnotatedString function is used to create a string with multiple style spans. It allows us to apply different styles to different parts of the text string.
The withStyle is used to apply the SpanStyle to the text. In the first block, a red color is applied to the text “Coding”. In the second block, a blue color is applied to the text “Rashid”.
The append function is used to append text. It is used to append the text “with” to the buildAnnotatedString object without applying any styles.
See the following output.
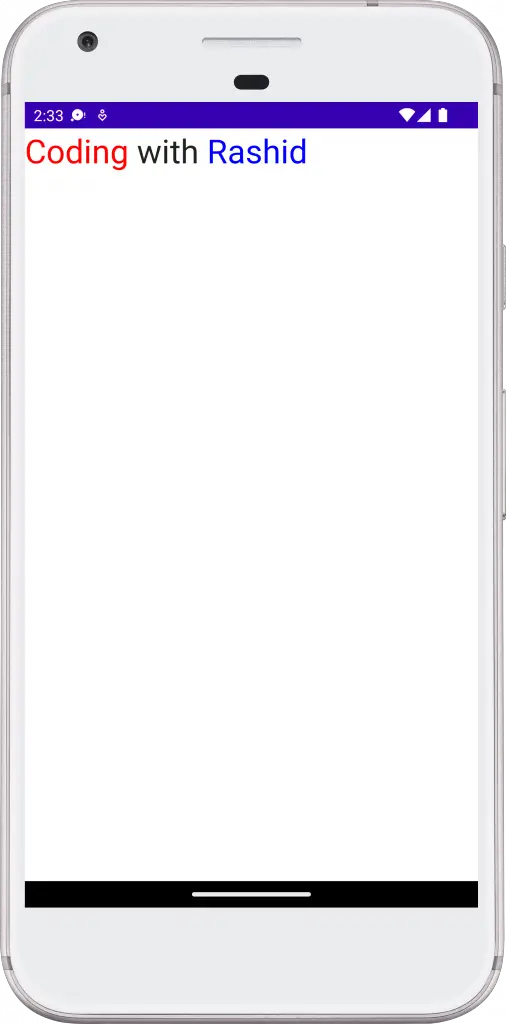
Go through the complete code given below.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.SpanStyle
import androidx.compose.ui.text.buildAnnotatedString
import androidx.compose.ui.text.withStyle
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
MultipleColorsInText()
}
}
}
}
}
@Composable
fun MultipleColorsInText() {
Text( text =
buildAnnotatedString {
withStyle(style = SpanStyle(color = Color.Red)) {
append("Coding ")
}
append("with ")
withStyle(style = SpanStyle(color = Color.Blue)) {
append("Rashid")
}
},
fontSize = 30.sp
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
MultipleColorsInText()
}
}
If you want to create gradient text then see this Jetpack Compose text gradient tutorial.
I hope this Jetpack Compose tutorial on multiple color text style is helpful for you.
One Comment