How to make Text Bold in Android Jetpack Compose
Text is a very important composable to display text while developing the UI of your Android app. In this blog post let’s learn how to make text bold in Jetpack Compose.
To style the text in a Compose function, we can use various parameters of the Text composable. The text composable has a fontWeight parameter to make the defined text bold.
The fontWeight takes a value from the FontWeight class, which includes options for various font weights such as bold, medium, and light. To make text bold, we use the fontWeight attribute with the bold value.
See the code snippet given below.
@Composable
fun BoldText() {
Text("Coding with Rashid",
fontSize = 40.sp,
fontWeight = FontWeight.Bold)
}
You will get the following output.
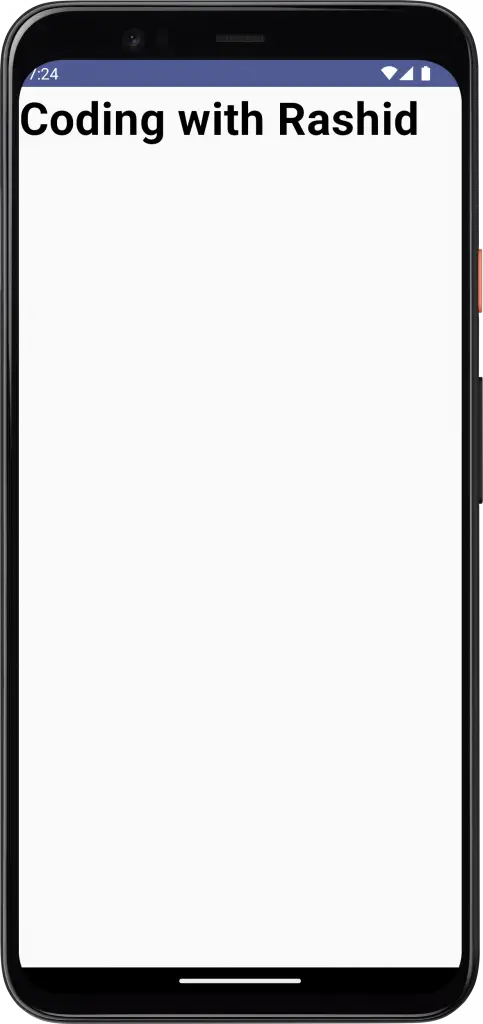
If you want the text to be extra bold you should use FontWeight.ExtraBold as value.
@Composable
fun BoldText() {
Text("Coding with Rashid", fontWeight = FontWeight.ExtraBold)
}
Then you will get the following output.
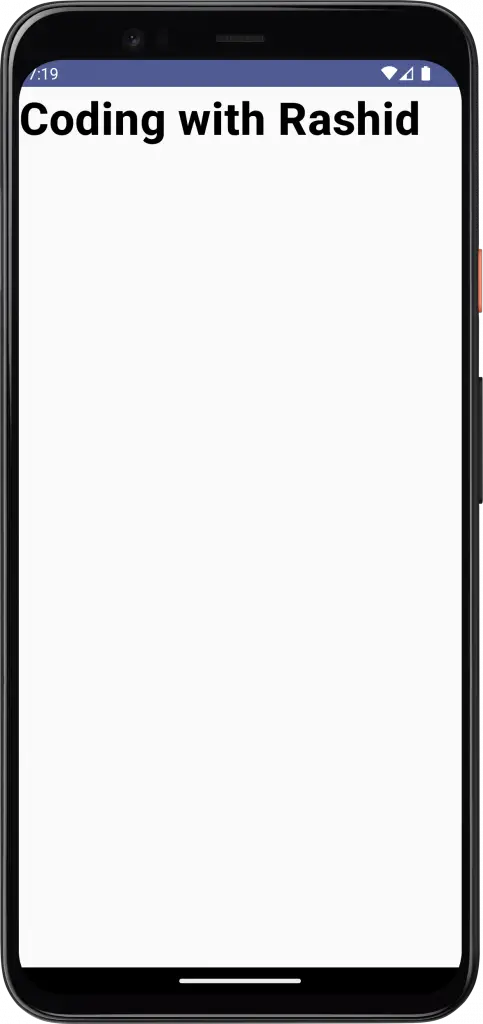
In addition to these basic styling options, Jetpack Compose also supports text spans, which allow us to apply different styles to different parts of a text string. Here’s an example of how to use text spans to make one word bold.
@Composable
fun BoldText() {
Text(fontSize = 40.sp,
text = buildAnnotatedString {
append("Coding with ")
withStyle(style = SpanStyle(fontWeight = FontWeight.Bold)) {
append("Rashid!")
}
})
}
You will get the following output with only one word made bold.
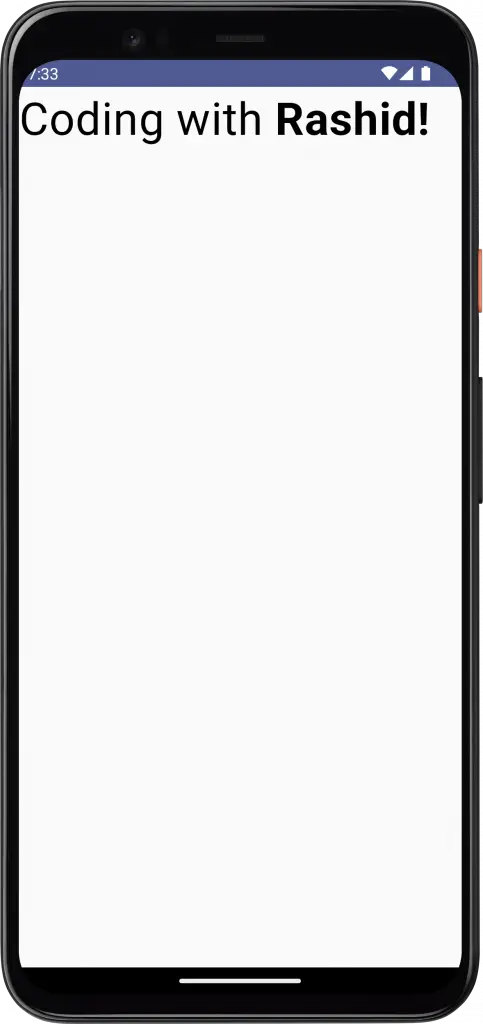
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.SpanStyle
import androidx.compose.ui.text.buildAnnotatedString
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.text.withStyle
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
BoldText()
}
}
}
}
}
@Composable
fun BoldText() {
Text(fontSize = 40.sp,
text = buildAnnotatedString {
append("Coding with ")
withStyle(style = SpanStyle(fontWeight = FontWeight.Bold)) {
append("Rashid!")
}
})
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
BoldText()
}
}
That’s how you add text with bold style in Jetpack Compose.
2 Comments