How to add Custom Fonts in Android Jetpack Compose
Fonts have an important place in the UI of an Android app. The right usage of custom fonts can make your app more appealing to users. Let’s learn how to add custom fonts in Jetpack Compose.
Firstly download your custom font and its varieties such as regular, medium, bold, etc. Then under the res directory create a new Android resource directory named font. Remember that the name of the font file should only have lowercase, a-z, 0-9 etc. See the project structure in the screenshot given below.
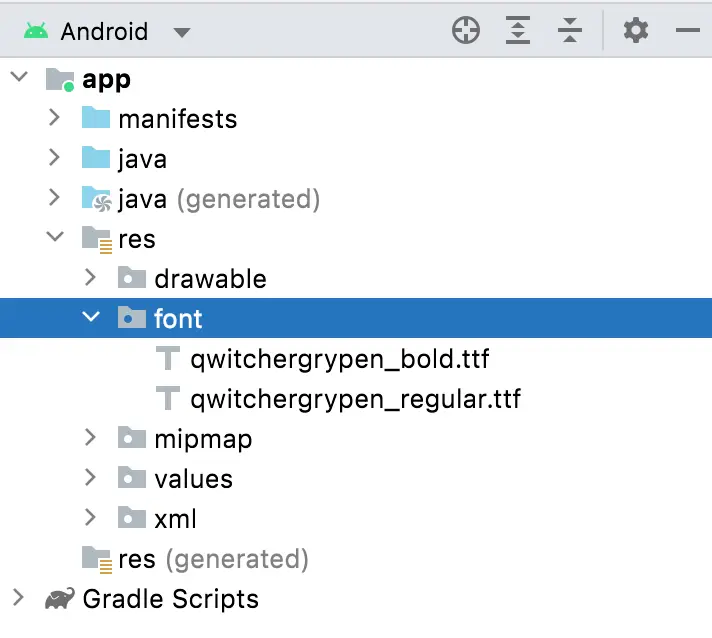
You have to define fontFamily to use these custom fonts.
val qwitcherGrypenFamily = FontFamily(
Font(R.font.qwitchergrypen_regular, FontWeight.Normal),
Font(R.font.qwitchergrypen_bold, FontWeight.Bold),
)
Then call it in the Text composable.
Text("Coding with Rashid", fontFamily = qwitcherGrypenFamily,
fontWeight = FontWeight.Normal, fontSize = 44.sp)
Following is the complete custom font example.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.Shadow
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.text.font.Font
import androidx.compose.ui.text.font.FontFamily
import androidx.compose.ui.text.font.FontStyle
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colors.background
) {
TextCustomFont()
}
}
}
}
}
@Preview(showBackground = true)
@Composable
fun TextCustomFont() {
val qwitcherGrypenFamily = FontFamily(
Font(R.font.qwitchergrypen_regular, FontWeight.Normal),
Font(R.font.qwitchergrypen_bold, FontWeight.Bold),
)
Column {
Text("Coding with Rashid", fontFamily = qwitcherGrypenFamily,
fontWeight = FontWeight.Normal, fontSize = 44.sp)
Text("Coding with Rashid", fontFamily = qwitcherGrypenFamily,
fontWeight = FontWeight.Bold, fontSize = 44.sp)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
ExampleTheme {
TextCustomFont()
}
}
See the output given below.
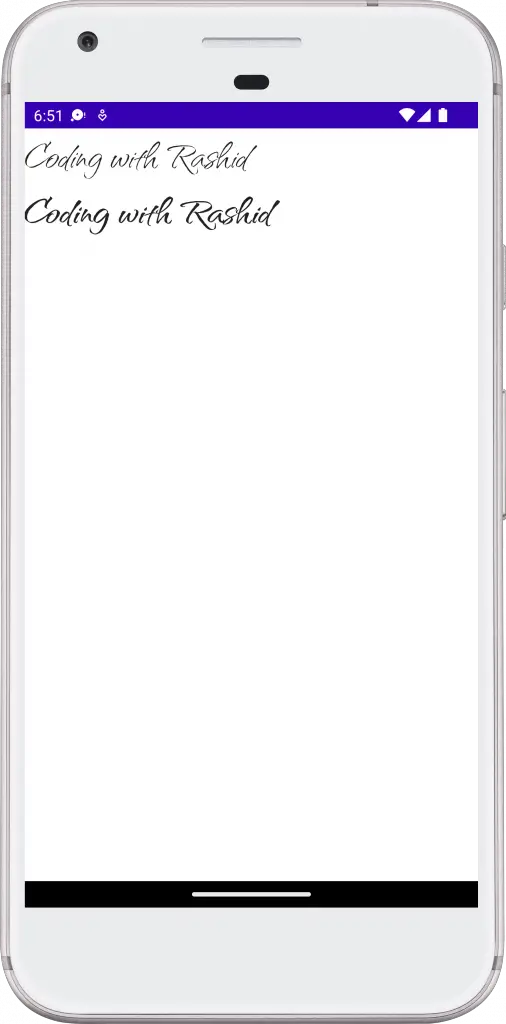
I hope this tutorial to add custom font in Android Jetpack Compose will help you.
2 Comments