How to Create Sweep Gradient in Android Jetpack Compose
Gradients have always been a staple in UI design, offering unique and eye-catching transitions that can enhance the visual appeal of an application. Sweep gradients, in particular, provide a vibrant circular color transition that can add an interesting touch to your designs.
This blog post will guide you through the creation and implementation of sweep gradients in Jetpack Compose.
How to Create Sweep Gradient
Sweep gradients create a color transition in a sweeping, circular pattern around a defined center. In Jetpack Compose, you can create these gradients using the Brush.sweepGradient function.
Let’s create a simple sweep gradient that transitions from red to green, and then to blue in a circular pattern.
val gradient = Brush.sweepGradient(
colorStops = arrayOf(
0.0f to Color.Red,
0.3f to Color.Green,
1.0f to Color.Blue
),
center = Offset(0.0f, 100.0f)
)
In this example, we’ve defined a sweep gradient with three color-stop pairs. Each pair includes a position along the gradient (ranging from 0.0f to 1.0f) and a color. The center parameter specifies the center of the gradient’s circle.
The gradient starts with red at the top (0.0f), transitions to green at about a third of the way around the circle (0.3f), and finally transitions to blue at the bottom (1.0f).
How to Implement Sweep Gradient
Once you’ve created your gradient, you can apply it to any composable that takes a Brush parameter. For instance, to use it as the background of a Box.
@Composable
fun GradientExample() {
val gradient = Brush.sweepGradient(
0.0f to Color.Red,
0.3f to Color.Green,
1.0f to Color.Blue,
)
Box(modifier = Modifier
.fillMaxSize()
.background(gradient))
}
In this snippet, the Modifier.background function is used to set our sweep gradient as the Box’s background, and Modifier.fillMaxSize() ensures that the Box fills all the available space. You will get the following output.
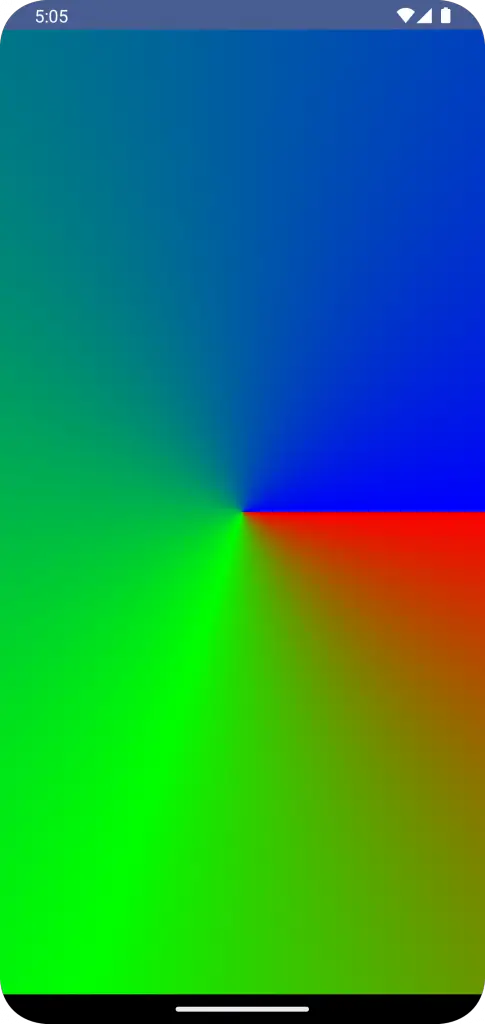
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
GradientExample()
}
}
}
}
}
@Composable
fun GradientExample() {
val gradient = Brush.sweepGradient(
0.0f to Color.Red,
0.3f to Color.Green,
1.0f to Color.Blue,
)
Box(modifier = Modifier
.fillMaxSize()
.background(gradient))
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
GradientExample()
}
}
Creating sweep gradients in Jetpack Compose is a straightforward process that can add a unique and interesting touch to your app’s UI. They are an excellent tool for introducing dynamic color transitions to your app and enhancing its aesthetic appeal.