How to Create Alert Dialog in Android Jetpack Compose
Alert dialogs are crucial UI components in Android apps, often used to display important messages or prompt user action. With Jetpack Compose taking center stage, building these alert dialogs has never been simpler.
In this tutorial, we will walk through the steps to create a simple yet effective alert dialog using Jetpack Compose.
Importance of Alert Dialogs
Alert dialogs serve multiple purposes. They can notify users about important updates, ask for user confirmation before performing a certain task, or even provide quick options for user actions. Understanding how to implement them is essential for any Android developer.
The Alert Dialog Code Snippet
Below is the code snippet for creating an alert dialog in Jetpack Compose.
@Composable
fun AlertExample() {
var dialogVisibility by remember { mutableStateOf(true) }
if (dialogVisibility) {
AlertDialog(
onDismissRequest = {
dialogVisibility = false
},
title = {
Text(text = " Alert Title")
},
text = {
Text("This is a Jetpack Compose tutorial on AlertDialog.")
},
confirmButton = {
Button(
modifier = Modifier.fillMaxWidth(),
onClick = { dialogVisibility = false }
) {
Text("Okay")
}
}
)
}
}
In this code, dialogVisibility
controls whether the dialog is shown or not. We used remember { mutableStateOf(true) }
to hold the dialog’s state.
Code Explanation
dialogVisibility
is a mutable state variable that controls the visibility of the AlertDialog.onDismissRequest
is a function that is called when the dialog is dismissed. We setdialogVisibility
to false when this happens.title
andtext
parameters are self-explanatory. They define the dialog’s title and text content, respectively.confirmButton
defines what happens when the user clicks the “Okay” button. In our example, the dialog is hidden.
Complete Example Including Main Activity
If you’re interested in seeing how this all ties together, here’s how you can include this composable function in your main activity.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.material3.AlertDialog
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
AlertExample()
}
}
}
}
}
}
@Composable
fun AlertExample() {
var dialogVisibility by remember { mutableStateOf(true) }
if (dialogVisibility) {
AlertDialog(
onDismissRequest = {
dialogVisibility = false
},
title = {
Text(text = " Alert Title")
},
text = {
Text("This is a Jetpack Compose tutorial on AlertDialog.")
},
confirmButton = {
Button(
modifier = Modifier.fillMaxWidth(),
onClick = { dialogVisibility = false }
) {
Text("Okay")
}
}
)
}
}
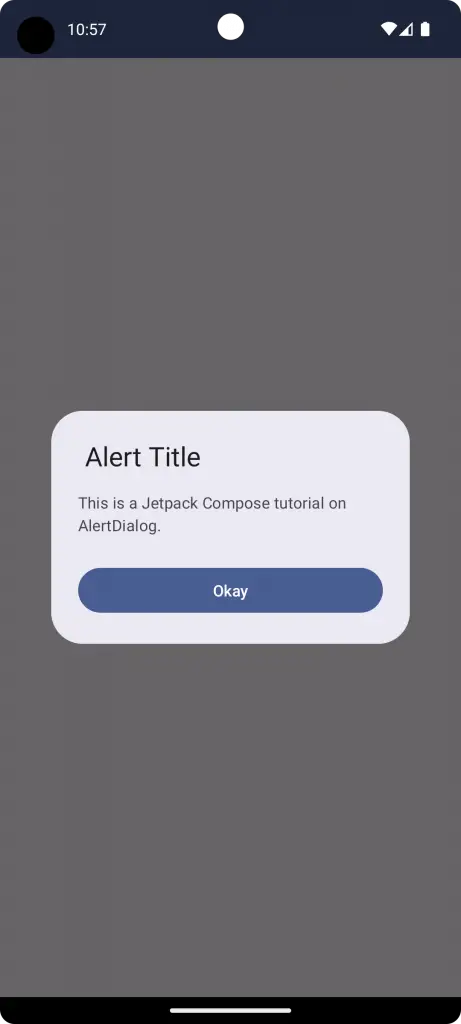
Creating an alert dialog in Jetpack Compose is relatively straightforward. You can easily customize it to fit the needs of your Android application. As Jetpack Compose continues to evolve, it will likely become an increasingly important tool for Android developers.