How to Create Transparent TopAppBar in Android Jetpack Compose
When crafting user interfaces with Android’s Jetpack Compose, flexibility and customization are key. One of the common requirements that developers might encounter is making the TopAppBar component transparent.
This blog post will guide you through the process of creating a transparent TopAppBar in Jetpack Compose.
What is TopAppBar?
In Jetpack Compose, the TopAppBar is a composable that displays information and actions related to the current screen. It’s commonly used to hold the title of the screen, navigation icons, and action items.
Making the TopAppBar Transparent
In order to make the TopAppBar transparent, you can leverage TopAppBarDefaults.smallTopAppBarColors. This function allows you to customize the colors of the TopAppBar.
Here’s an example:
@Composable
fun AppBarExample() {
Scaffold(
topBar = {
TopAppBar(
title = {
Text("My App")
},
colors = TopAppBarDefaults.smallTopAppBarColors(containerColor = Color.Transparent )
)
}
) { contentPadding ->
// Screen content
Text(text = "Jetpack Compose App Bar Example",
modifier = Modifier.padding(contentPadding))
}
}
Following is the output with a transparent appbar.
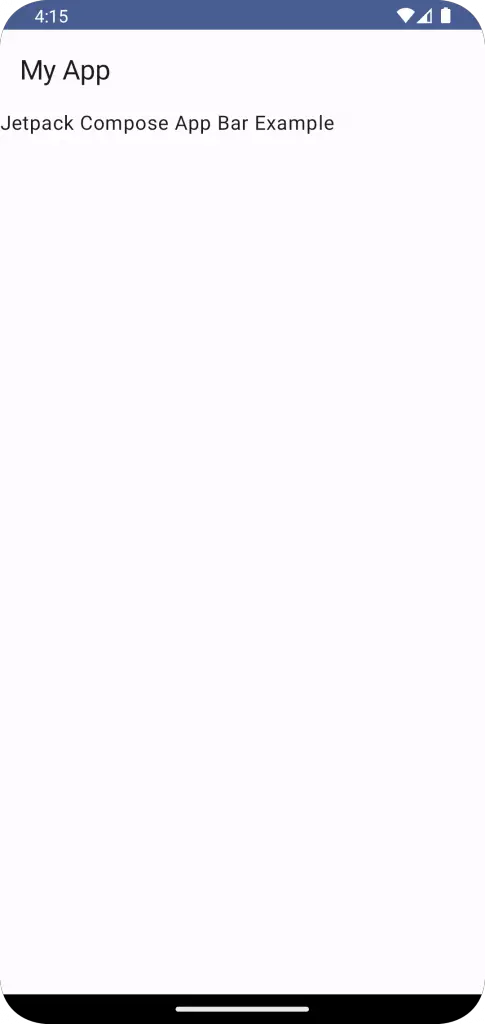
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TopAppBar
import androidx.compose.material3.TopAppBarDefaults
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AppBarExample()
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun AppBarExample() {
Scaffold(
topBar = {
TopAppBar(
title = {
Text("My App")
},
colors = TopAppBarDefaults.smallTopAppBarColors(containerColor = Color.Transparent )
)
}
) { contentPadding ->
// Screen content
Text(text = "Jetpack Compose App Bar Example",
modifier = Modifier.padding(contentPadding))
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
AppBarExample()
}
}
Creating a transparent TopAppBar in Jetpack Compose is a straightforward process. I hope this tutorial is helpful for you.