How to Add Ellipsis to Text in Android Jetpack Compose
In UI design, dealing with lengthy text that does not fit within its designated space is a common scenario. For Android apps, one popular solution is to display an ellipsis (“…”) at the end of the visible portion of the text to indicate the overflow.
Jetpack Compose, Android’s modern UI toolkit, makes it easy to implement this functionality. In this post, we’ll walk you through the process of adding ellipsis to your text in Jetpack Compose.
What is Ellipsis?
In UI design, an ellipsis is typically used to denote that a text string has been cut off because it’s too long to fit within its container. The ellipsis character, represented by three dots (“…”), is displayed at the point where the text is truncated.
How to Add Ellipsis in Jetpack Compose
In Jetpack Compose, the Text Composable function is used to display text. It includes a property called maxLines that defines the maximum number of lines the text can take up before it is cut off. When combined with the overflow property set to TextOverflow.Ellipsis, it will display an ellipsis at the end of the final line if the text exceeds the maximum lines.
Here’s an example of how to use these properties:
@Composable
fun EllipsisExample() {
Text(
text = "This is a very long text that will not fit in a single line",
maxLines = 1,
fontSize = 24.sp,
overflow = TextOverflow.Ellipsis
)
}
In this code snippet, we’ve set maxLines to 1 and overflow to TextOverflow.Ellipsis. So if the text doesn’t fit in a single line, it’ll be cut off, and an ellipsis will be displayed at the end of the visible portion.
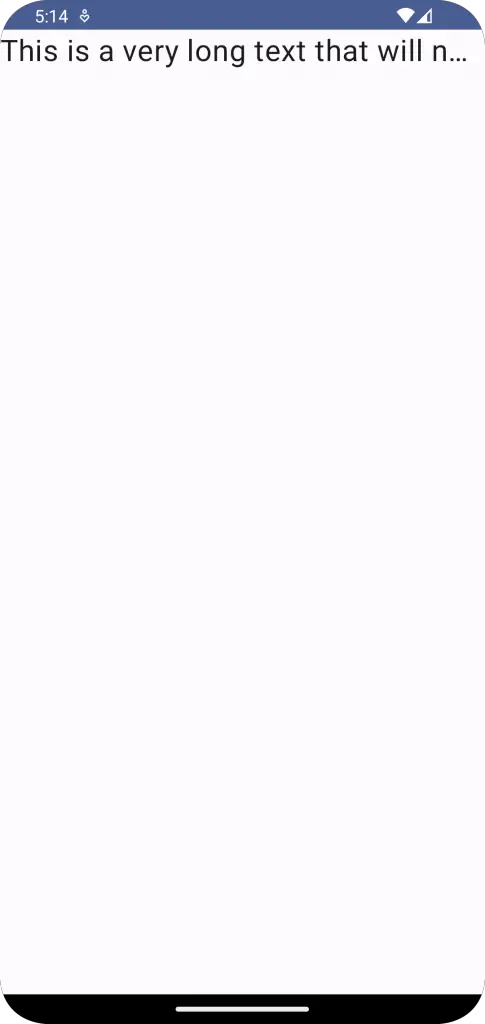
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.style.TextOverflow
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
EllipsisExample()
}
}
}
}
}
@Composable
fun EllipsisExample() {
Text(
text = "This is a very long text that will not fit in a single line",
maxLines = 1,
fontSize = 24.sp,
overflow = TextOverflow.Ellipsis
)
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
EllipsisExample()
}
}
Dealing with lengthy text that doesn’t fit within its designated space is a common task when building UI. With Jetpack Compose, implementing a solution to handle this situation, such as displaying an ellipsis, is straightforward and easy.
Remember to use these properties carefully as overusing ellipsis might make the content less accessible to the users. Understanding your content and applying the ellipsis wisely will ensure your app’s UI remains user-friendly.