How to Add Rounded Corners to Row in Android Jetpack Compose
In Jetpack Compose, we can easily create user interface components with rounded corners, such as a Row. Row is a simple layout composable that places its children in a horizontal sequence.
To add rounded corners to a Row, we need to use a Modifier. Modifiers in Jetpack Compose are key to decorating or adding behavior to composable functions. The background() function in the Modifier allows us to set the background color and the shape of the composable, which can be used to add rounded corners.
Let’s take a look at the following code example:
Row(
modifier = Modifier
.fillMaxWidth()
.padding(16.dp)
.background(
color = Color.Cyan,
shape = RoundedCornerShape(20.dp)
),
) {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.padding(16.dp),
fontSize = 16.sp
)
}
In the background function, Color.Cyan sets the background color of the Row. The shape parameter accepts a Shape object that determines the shape of the background. We’ve used RoundedCornerShape, a predefined Shape in Jetpack Compose that generates a rectangle with rounded corners.
The Text composable within the Row is simple and displays the message “Hello, Jetpack Compose!”. Following is the output.
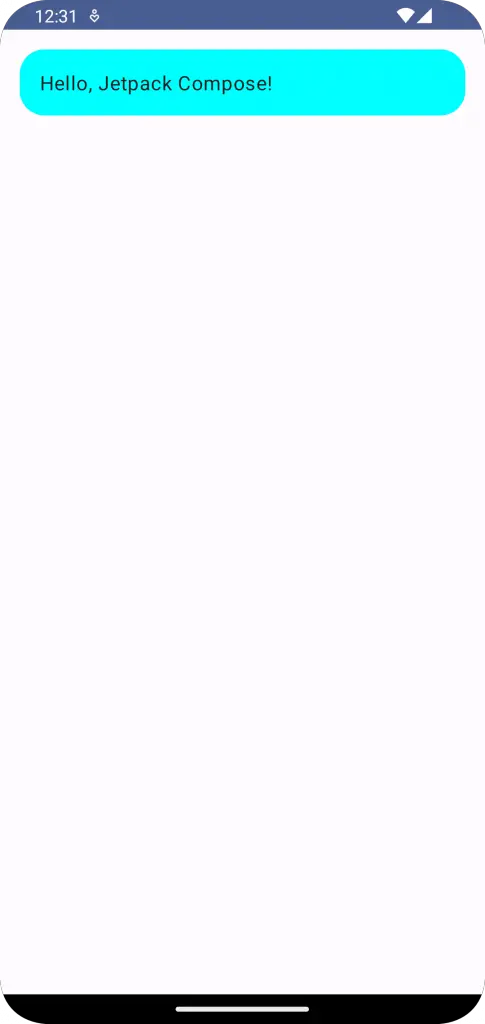
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
RowExample()
}
}
}
}
}
@Composable
fun RowExample() {
Column {
Row(
modifier = Modifier
.fillMaxWidth()
.padding(16.dp)
.background(
color = Color.Cyan,
shape = RoundedCornerShape(20.dp)
)
) {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.padding(16.dp),
fontSize = 16.sp
)
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
RowExample()
}
}
Jetpack Compose has made it convenient and efficient for developers to create complex UIs in a simplified manner. By understanding how to leverage modifiers, we can create rows, columns, or any other composable with rounded corners, helping to enhance the user experience of the application.