How to add Strikethrough Text in Android Jetpack Compose
Strikethrough text is considered as a way to say something without really saying it. In this Jetpack Compose tutorial, let’s learn how to add strikethrough text in Android.
Here we use the Text composable to show text and the TextDecoration class to decorate text with underline, strikethrough, etc.
See the code snippet given below.
@Composable
fun StrikethroughText() {
Column {
Text(text = "This is strikethrough text",
textDecoration = TextDecoration.LineThrough)
}
}
The above code creates a composable function called StrikethroughText that returns a Text composable with the specified text and strikethrough decoration.
TextDecoration.LineThrough changes the normal text into strikethrough text. Following is the output.
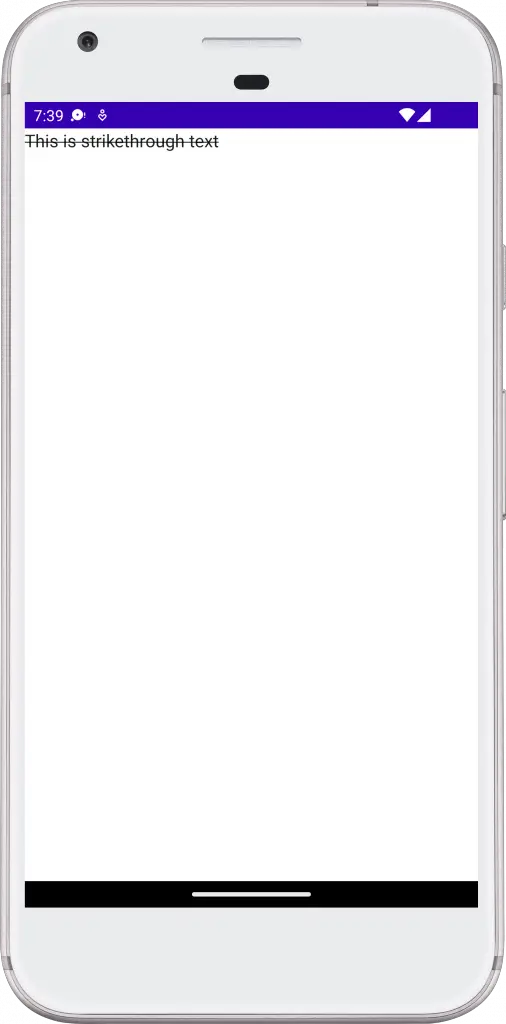
It’s worth noting that the TextDecoration class also provides plus method to combine two properties. For example, you can make a text underlined as well as strikethrough as given below.
@Composable
fun UnderlinedText() {
Text(
text = "This text is underlined",
textDecoration = TextDecoration.Underline.plus(TextDecoration.LineThrough),
)
}
Then you will get the following output.
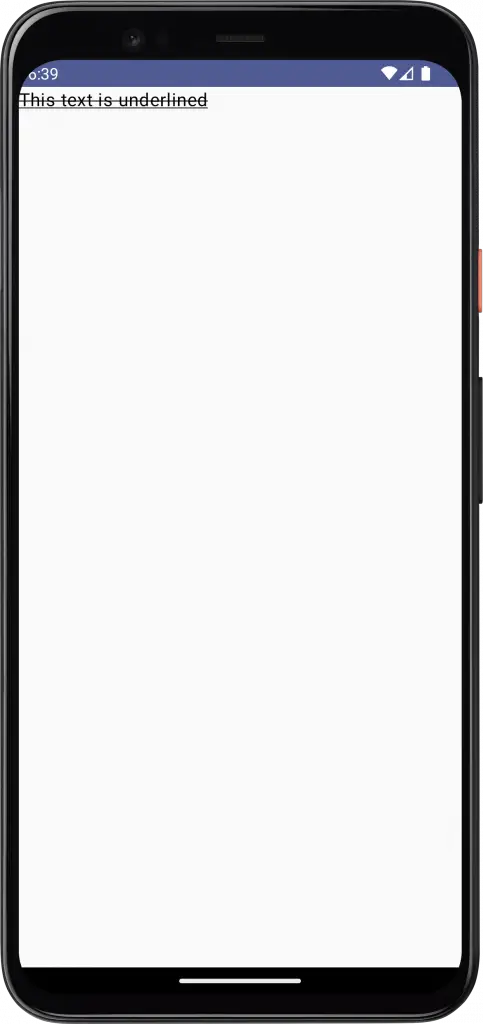
The TextDecoration can be used with other composable functions that display text, such as AnnotatedString. This allows you to apply a strikethrough style to specific words.
@Composable
fun StrikethroughText() {
Text(fontSize = 40.sp,
text = buildAnnotatedString {
append("Coding with ")
withStyle(style = SpanStyle(textDecoration = TextDecoration.LineThrough)) {
append("Rashid!")
}
})
}
And you will get the following output.
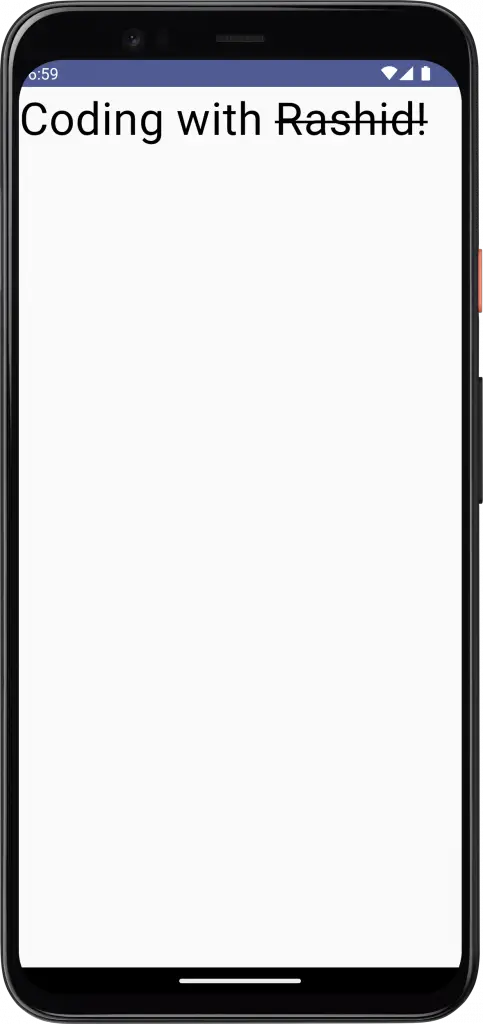
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.SpanStyle
import androidx.compose.ui.text.buildAnnotatedString
import androidx.compose.ui.text.style.TextDecoration
import androidx.compose.ui.text.withStyle
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
StrikethroughText()
}
}
}
}
}
@Composable
fun StrikethroughText() {
Text(fontSize = 40.sp,
text = buildAnnotatedString {
append("Coding with ")
withStyle(style = SpanStyle(textDecoration = TextDecoration.LineThrough)) {
append("Rashid!")
}
})
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
StrikethroughText()
}
}
I hope this Jetpack Compose tutorial is helpful for you.
One Comment