How to Display Base64 Data as Image in Android Jetpack Compose
Images not only enhance the visual appeal of the app but also help in conveying important information to the users. In this blog post, let’s learn how to show base64 data as an image in Jetpack Compose.
See the code snippet given below to display the base64 image.
val base64String = "iVBORw0KGgoAAAANSUhEUgAAADMAAAAzCAYAAAA6oTAqAAAAEXRFWHRTb2Z0d2FyZQBwbmdjcnVzaEB1SfMAAABQSURBVGje7dSxCQBACARB+2/ab8BEeQNhFi6WSYzYLYudDQYGBgYGBgYGBgYGBgYGBgZmcvDqYGBgmhivGQYGBgYGBgYGBgYGBgYGBgbmQw+P/eMrC5UTVAAAAABJRU5ErkJggg=="
val byteArray = Base64.decode(base64String, Base64.DEFAULT)
val bitmap = BitmapFactory.decodeByteArray(byteArray, 0, byteArray.size)
Image(bitmap = bitmap.asImageBitmap(),
contentDescription = null,
modifier = Modifier.size(200.dp)
)
The image data is provided as a Base64 encoded string, which is first decoded into a byte array using the Base64.decode() method. The byte array is then used to create a Bitmap object using the BitmapFactory.decodeByteArray() method.
The created bitmap is then passed to the Image component as the “bitmap” parameter, along with a modifier to set the size of the image to 200 dp.
You will get the following output.
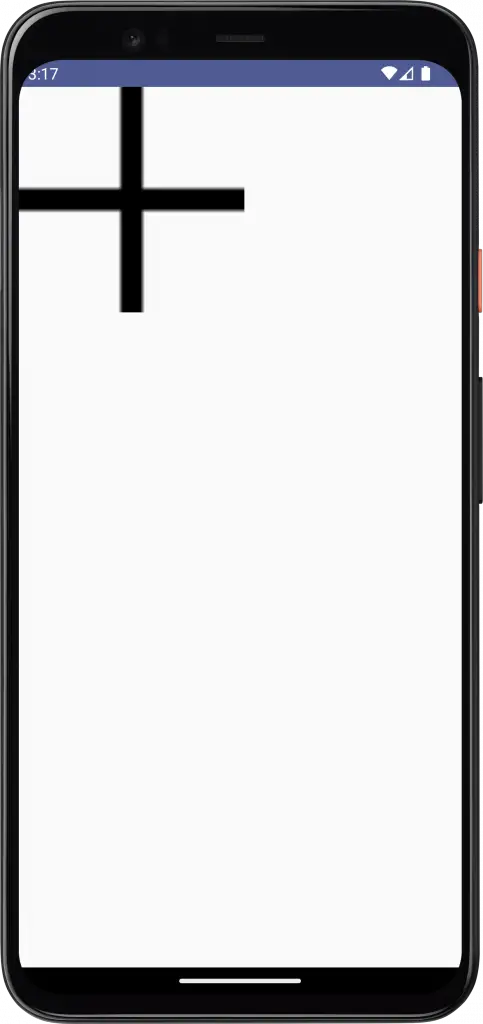
Following is the complete code for reference.
package com.example.myapplication
import android.graphics.BitmapFactory
import android.os.Bundle
import android.util.Base64
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.material3.*
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.asImageBitmap
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
val base64String = "iVBORw0KGgoAAAANSUhEUgAAADMAAAAzCAYAAAA6oTAqAAAAEXRFWHRTb2Z0d2FyZQBwbmdjcnVzaEB1SfMAAABQSURBVGje7dSxCQBACARB+2/ab8BEeQNhFi6WSYzYLYudDQYGBgYGBgYGBgYGBgYGBgZmcvDqYGBgmhivGQYGBgYGBgYGBgYGBgYGBgbmQw+P/eMrC5UTVAAAAABJRU5ErkJggg=="
val byteArray = Base64.decode(base64String, Base64.DEFAULT)
val bitmap = BitmapFactory.decodeByteArray(byteArray, 0, byteArray.size)
Image(bitmap = bitmap.asImageBitmap(),
contentDescription = null,
modifier = Modifier.size(200.dp)
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
That’s how you add base64 images in Jetpack Compose.