How to Add Checkbox with Text in Android Jetpack Compose
Checkboxes play a significant role in user interfaces. They offer a straightforward, interactive way for users to make selections or indicate preferences in a form. From agreeing to terms and conditions, selecting multiple items from a list, to setting up user preferences, checkboxes provide a visual indicator for a two-way choice.
In mobile app development, coupling checkboxes with text helps to provide context for the choice being made. This can enhance user understanding and improve the overall user experience.
Jetpack Compose, Android’s modern toolkit for building native UI, shines in its ability to customize these elements easily. In this blog post, we’re going to discuss how to create a composite UI element, a combination of a Checkbox and Text in a Row layout in Jetpack Compose.
We’ll begin with the end solution and then break it down to understand each part:
@Composable
fun CheckboxExample() {
Column() {
val (checkedState, onStateChange) = remember { mutableStateOf(false) }
Row(
Modifier
.fillMaxWidth()
.height(56.dp)
.toggleable(
value = checkedState,
onValueChange = { onStateChange(!checkedState) },
role = Role.Checkbox
)
.padding(horizontal = 16.dp),
verticalAlignment = Alignment.CenterVertically
) {
Checkbox(
checked = checkedState,
onCheckedChange = null
)
Text(
text = "Select Option",
modifier = Modifier.padding(start = 16.dp)
)
}
}
}
Let’s break down this code:
We use the remember { mutableStateOf(false) } to create a mutable state of the Checkbox. This state will change each time the Checkbox is clicked.
We wrap the Checkbox and Text within a Row layout, giving it a width that spans the full screen (fillMaxWidth()) and a height of 56 dp. The entire Row is made toggleable to mimic the behavior of a Checkbox, changing the state each time the Row is clicked.
Inside the Row, we place a Checkbox and Text. The Checkbox’s state is tied to the checkedState. The Text is styled and given a padding to separate it from the Checkbox. Following is the output.
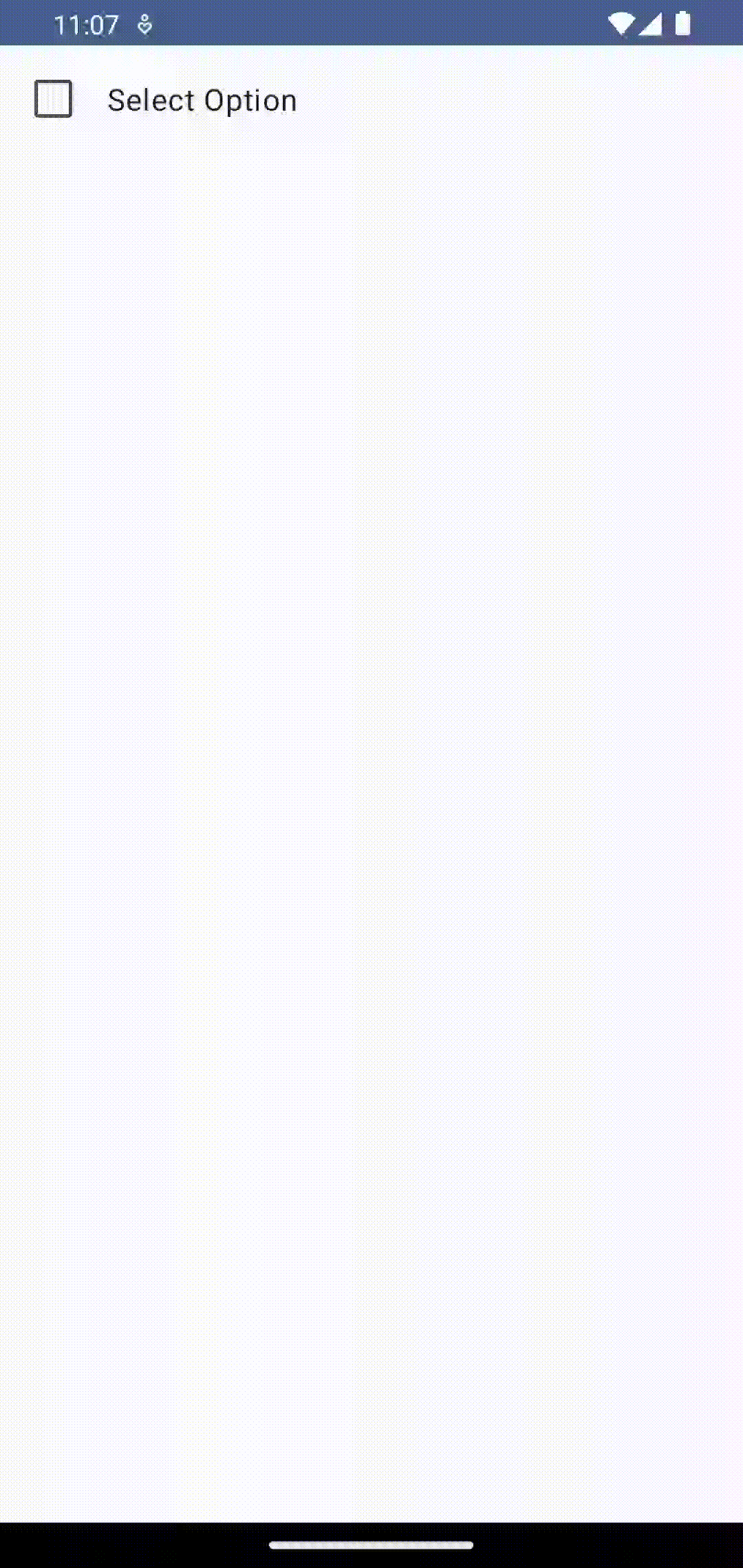
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.selection.toggleable
import androidx.compose.material3.Checkbox
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.semantics.Role
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CheckboxExample()
}
}
}
}
}
@Composable
fun CheckboxExample() {
Column() {
val (checkedState, onStateChange) = remember { mutableStateOf(false) }
Row(
Modifier
.fillMaxWidth()
.height(56.dp)
.toggleable(
value = checkedState,
onValueChange = { onStateChange(!checkedState) },
role = Role.Checkbox
)
.padding(horizontal = 16.dp),
verticalAlignment = Alignment.CenterVertically
) {
Checkbox(
checked = checkedState,
onCheckedChange = null
)
Text(
text = "Select Option",
modifier = Modifier.padding(start = 16.dp)
)
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
CheckboxExample()
}
}
In conclusion, we’ve managed to create a combination of a Checkbox and Text within a Row layout. This not only demonstrates the ability of Jetpack Compose to create complex UI elements, but also the power of state management in building interactive components.