How to add Vertical Line in Android Jetpack Compose
Lines are helpful to divide different UI elements of mobile apps. In this simple and short Jetpack Compose tutorial let’s learn how to add a vertical line.
You can use Divider from Jetpack Compose to draw lines- horizontal or vertical. Wrapping a Divider with Row makes the line vertical. See the code snippet given below.
@Composable
fun Example() {
Row(
horizontalArrangement = Arrangement.Center, modifier = Modifier.fillMaxSize()
) {
Divider(modifier = Modifier.fillMaxHeight().width(1.dp), color = Color.Black)
}
}
Following is the output.
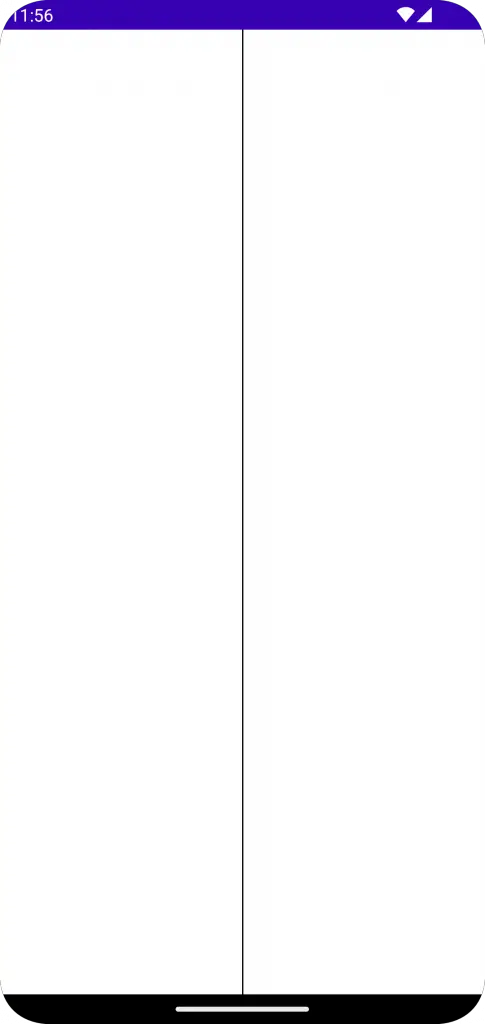
Complete Code
Following is the complete code of this Jetpack Compose example to show vertical line.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.fillMaxHeight
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.width
import androidx.compose.material3.Divider
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
Example()
}
}
}
}
}
}
@Composable
fun Example() {
Row(
horizontalArrangement = Arrangement.Center, modifier = Modifier.fillMaxSize()
) {
Divider(modifier = Modifier.fillMaxHeight().width(1.dp), color = Color.Black)
}
}
I hope this blog post will be helpful for you.
One Comment