How to add Horizontal Line in Android Jetpack Compose
Jetpack Compose is the preferred way to build the UI of your native Android app. In this Jetpack Compose tutorial, let’s learn how to show a horizontal line easily.
Jetpack Compose has Divider, which is basically a line that divides the space between elements. Placing a Divider inside the Column helps you to show a horizontal line. See the code snippet given below.
@Composable
fun Example() {
Column(horizontalAlignment = Alignment.CenterHorizontally,verticalArrangement = Arrangement.Center,
modifier = Modifier.fillMaxSize()
) {
Divider(thickness = 1.dp, color = Color.Black)
}
}
The output will be as given below.
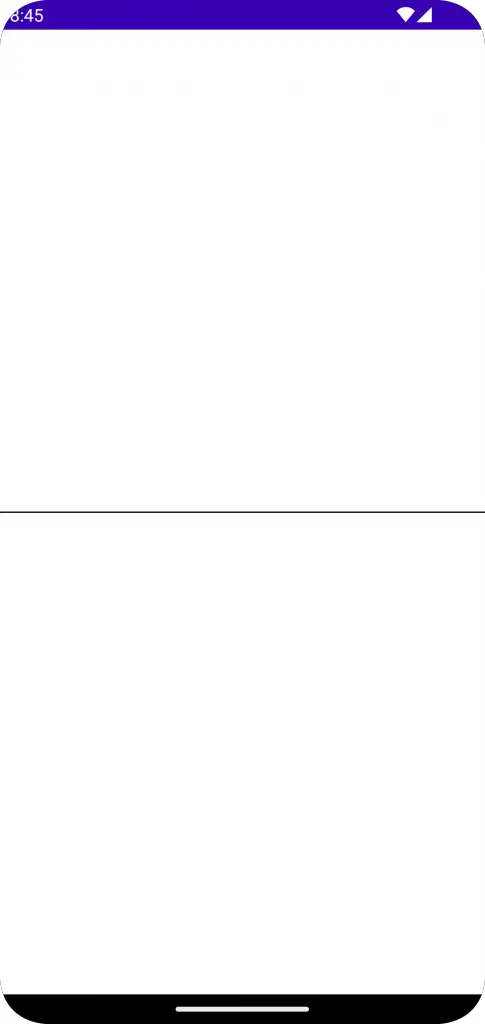
Following is the complete code.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.Divider
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
Example()
}
}
}
}
}
}
@Composable
fun Example() {
Column(horizontalAlignment = Alignment.CenterHorizontally,verticalArrangement = Arrangement.Center,
modifier = Modifier.fillMaxSize()
) {
Divider(thickness = 1.dp, color = Color.Black)
}
}
That’s how you draw horizontal lines in Jetpack Compose. Also, check out how to add a vertical line in jetpack compose.
One Comment