How to Add Icon Button in Android Jetpack Compose
Icon buttons are an important part of user interface design, as they provide users with a quick and easy way to access key features within an app. They can be used to perform actions such as saving or deleting data or accessing settings and other app-specific functions.
In this blog post, let’s learn how to add an icon button in Jetpack Compose.
The IconButton composable helps you to add an icon button easily. See the code snippet given below.
IconButton(
onClick = { /* ... */ },
modifier = Modifier.size(50.dp)
) {
Icon(imageVector = Icons.Filled.Add,
contentDescription = null,
modifier = Modifier.size(50.dp),
tint = Color.Red)
}
You will get the following output.
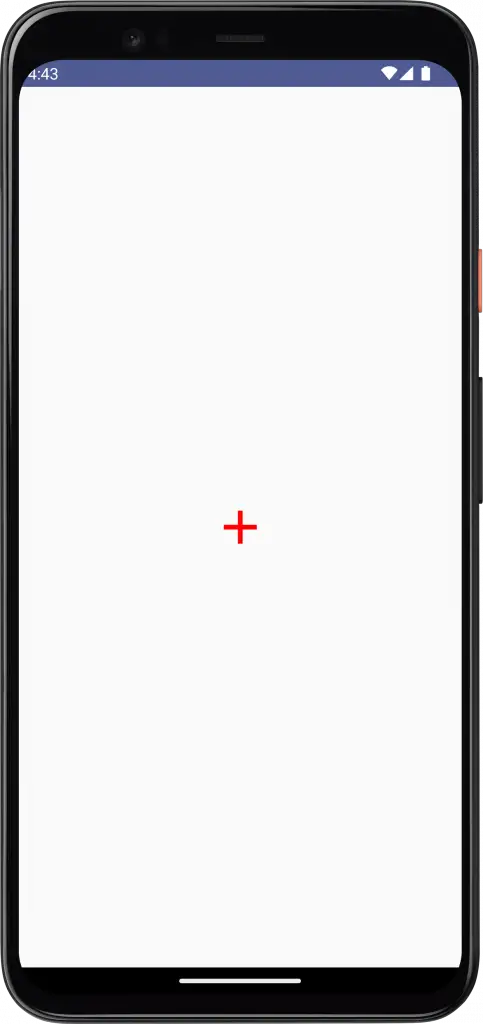
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Add
import androidx.compose.material3.*
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.Alignment
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ButtonExample()
}
}
}
}
}
@Composable
fun ButtonExample() {
Column(modifier = Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center) {
IconButton(
onClick = { /* ... */ },
modifier = Modifier.size(50.dp)
) {
Icon(imageVector = Icons.Filled.Add,
contentDescription = null,
modifier = Modifier.size(50.dp),
tint = Color.Red)
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ButtonExample()
}
}
That’s how you add IconButton in Jetpack Compose.