How to Set Button Border Color in Android Jetpack Compose
Creating visually appealing buttons in the mobile app is essential for providing a great user experience. One way to enhance the appearance of a button is by adding a border with a specific color. In this blog post, let’s check how to set the border color of a button in Jetpack Compose.
The Button composable is one of the most used components in Jetpack Compose. It has some useful parameters and the border is one among them. With BorderStroke class you can change the thickness as well as the color of the border too.
See the code snippet given below.
Button(
onClick = { /* ... */ },
colors = ButtonDefaults.buttonColors(Color.Blue),
border = BorderStroke(width = 2.dp, color = Color.Red)
) {
Text("Button")
}
You will get the following output.
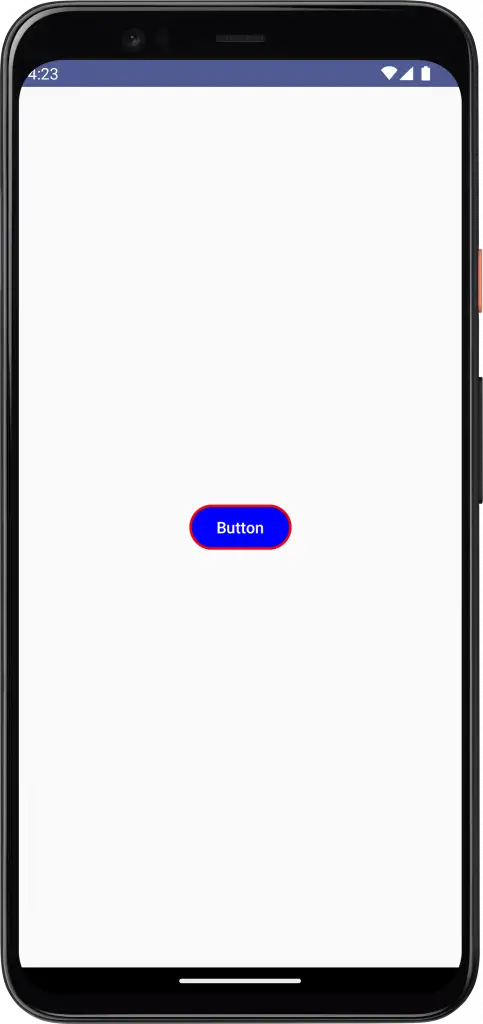
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.BorderStroke
import androidx.compose.foundation.layout.*
import androidx.compose.material3.*
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.Alignment
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ButtonExample()
}
}
}
}
}
@Composable
fun ButtonExample() {
Column(modifier = Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center) {
Button(
onClick = { /* ... */ },
colors = ButtonDefaults.buttonColors(Color.Blue),
border = BorderStroke(width = 2.dp, color = Color.Red)
) {
Text("Button")
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ButtonExample()
}
}
That’s how you change the button border color in Jetpack Compose.