How to Change TextField Placeholder Color in Android Jetpack Compose
Placeholders serve as guiding hints that help users understand what to enter into a TextField
. In Android Jetpack Compose, you have the power to customize these placeholders to fit the look and feel of your app.
This tutorial will guide you through the process of changing the color of the TextField
placeholder and delve into some more advanced features.
Why Placeholder Colors Matter
Before we dive into the code, let’s understand why customizing placeholder colors is essential. A well-chosen color can improve user experience by making the interface more intuitive and visually appealing.
The Basics: Change Placeholder Color
To start off, you can use textFieldColors
with the TextField
composable to set your desired placeholder color. Here’s the code snippet that demonstrates this:
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(
value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Username") },
colors = TextFieldDefaults.textFieldColors(
placeholderColor = Color.Red,
disabledPlaceholderColor = Color.Gray),
modifier = Modifier.fillMaxWidth().padding(10.dp)
)
}
With this code, you can use two parameters: placeholderColor
and disabledPlaceholderColor
. The former sets the active placeholder color, and the latter sets the color when the TextField
is disabled.
Further Customization: Change Label Color
Not just the placeholder, but you can also change the label color if your TextField
uses one. The attribute to look for is labelColor
within the textFieldColors
function.
Complete Code Example
Here’s the full code including the MainActivity
and the TextFieldExample
function:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.material3.TextFieldDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Username") },
colors = TextFieldDefaults.textFieldColors(placeholderColor = Color.Red,
disabledPlaceholderColor = Color.Gray),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
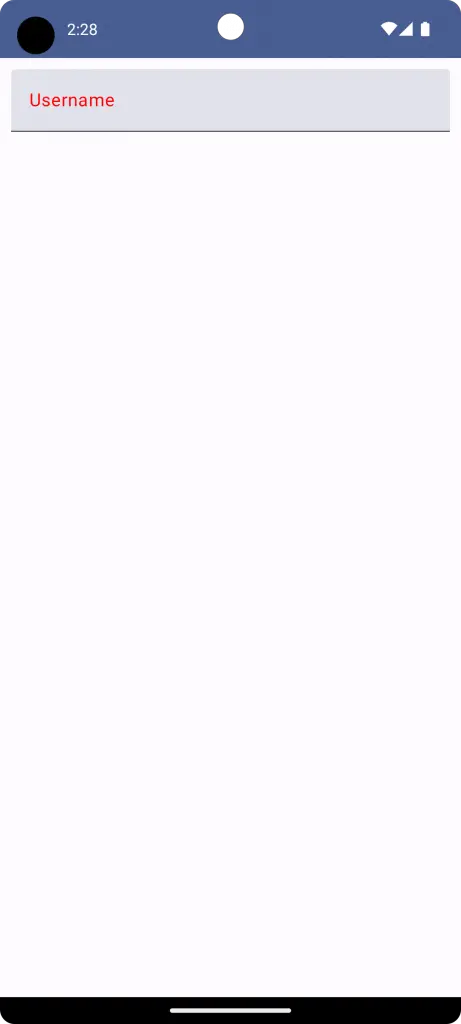
Customizing your app’s TextField
placeholder color can add a touch of personality and improve the user interface. Jetpack Compose provides an easy way to make these changes, offering a more tailored experience for your users.
One Comment