How to Change TextField Indicator Color in Android Jetpack Compose
An indicator line in a TextField serves multiple purposes in a user interface. It indicates focus, error, and even disabled states. Knowing how to change these indicator colors can make your app more intuitive and visually appealing.
In this tutorial, we’ll walk you through the steps to customize the indicator colors for various states in Jetpack Compose.
Use TextFieldColors to Customize
Jetpack Compose provides a textFieldColors
function. This function lets you change different aspects of a TextField, including placeholder color, label color, and indicator colors. Here’s a sample code snippet that demonstrates this:
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("") }
TextField(
value = textInput,
onValueChange = { textInput = it },
placeholder = { Text("Username") },
colors = TextFieldDefaults.textFieldColors(
focusedIndicatorColor = Color.Red,
disabledIndicatorColor = Color.Gray,
errorIndicatorColor = Color.Gray,
unfocusedIndicatorColor = Color.Gray
),
modifier = Modifier.fillMaxWidth()
.padding(10.dp)
)
}
In the code above, the TextFieldDefaults.textFieldColors
function takes in four parameters for the indicator:
focusedIndicatorColor
: Sets the color when the TextField is focused.disabledIndicatorColor
: Sets the color when the TextField is disabled.errorIndicatorColor
: Sets the color when there’s an error during validation.unfocusedIndicatorColor
: Sets the color when the TextField is not focused.
Each parameter takes a Color
object. This makes it easy to match your TextField to the overall theme of your app.
Once you implement the code, you’ll see that the TextField’s indicator color will change based on its state. For example, it will turn red when focused, as shown in our code snippet.
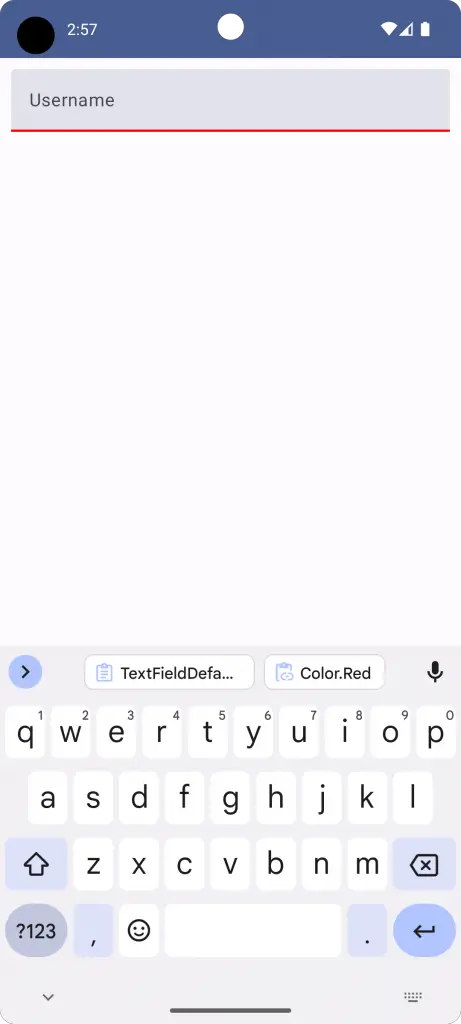
Complete Code
Below is the complete code that includes the MainActivity and the composable function:
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.material3.TextFieldDefaults
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Username") },
colors = TextFieldDefaults.textFieldColors(focusedIndicatorColor = Color.Red,
disabledIndicatorColor = Color.Gray, errorIndicatorColor = Color.Gray,
unfocusedIndicatorColor = Color.Gray),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
Customizing the TextField indicator color in Jetpack Compose is straightforward. You can easily set the color for different states like focus, error, and disabled. This allows you to make your app more user-friendly and aligned with your design language.