How to Add Elevation to Surface in Android Jetpack Compose
In the world of Android UI development, Jetpack Compose has become a favorite among developers for its simplicity, conciseness, and power. One of the essential elements in a Material Design styled Android application is the Surface Composable. It allows you to add elevation and rounded corners to your UI components, creating a paper-like visual metaphor.
In this tutorial, we will focus on one of the most significant properties of the Surface Composable, the elevation. The elevation attribute is used to cast shadows and create the illusion of depth, making a component appear lifted above the background. Let’s get started on how to set the elevation in Jetpack Compose Surface.
Here’s a simple example of a Surface with an elevation:
@Composable
fun SurfaceExample() {
Surface(
modifier = Modifier.padding(32.dp).fillMaxSize(),
color = Color.Cyan,
shadowElevation = 8.dp,
) {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.padding(16.dp)
)
}
}
In this example, we have a Surface with a cyan color. The shadowElevation is set to 8.dp, which will render a subtle shadow beneath the surface.
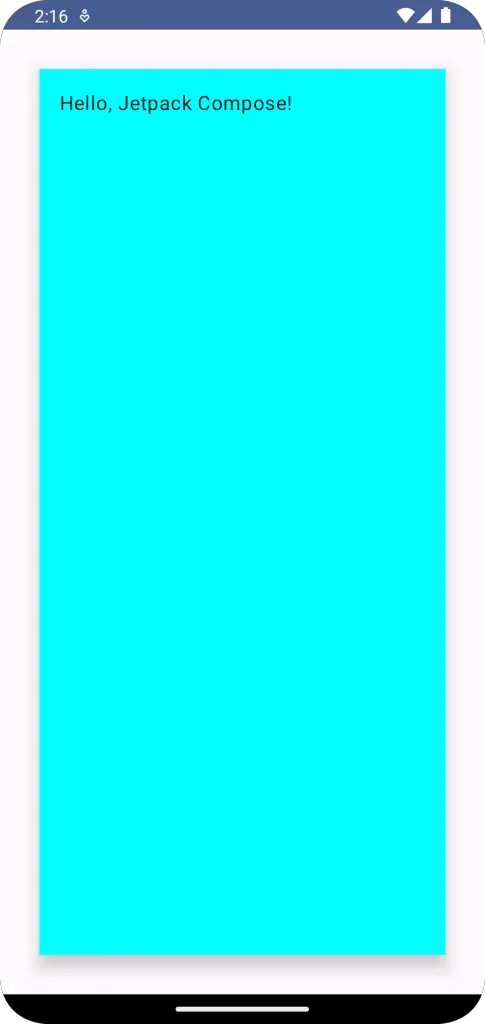
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
SurfaceExample()
}
}
}
}
}
@Composable
fun SurfaceExample() {
Surface(
modifier = Modifier.padding(32.dp).fillMaxSize(),
color = Color.Cyan,
shadowElevation = 8.dp,
) {
Text(
text = "Hello, Jetpack Compose!",
modifier = Modifier.padding(16.dp)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
SurfaceExample()
}
}
By using the Surface Composable in Jetpack Compose, you can add depth to your application’s UI by playing around with different elevations. A higher value of elevation would increase the intensity of the shadow, giving an impression of a component being closer to the user, while a lower value will make the component appear further away.