How to Center Crop an Image in Android Jetpack Compose
Sometimes, you may want to show an image after the center crop in your app. In this blog post, let’s check how to center crop an image in Jetpack Compose.
The Image composable has many properties for customization. The contentScale option is one among them. By default the Image composable use ContentScale.Fit. In order to center crop we should use ContentScale.Crop and this center crop the image into the available space.
See the code snippet given below.
Image(painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
contentScale = ContentScale.Crop,
modifier = Modifier.width(400.dp).height(400.dp)
)
I used the following image to demonstrate the case in this example.

Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.aspectRatio
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.width
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.graphics.ColorMatrix
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
Image(painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
contentScale = ContentScale.Crop,
modifier = Modifier.width(400.dp).height(400.dp)
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
Then you will get the center cropped image as output.
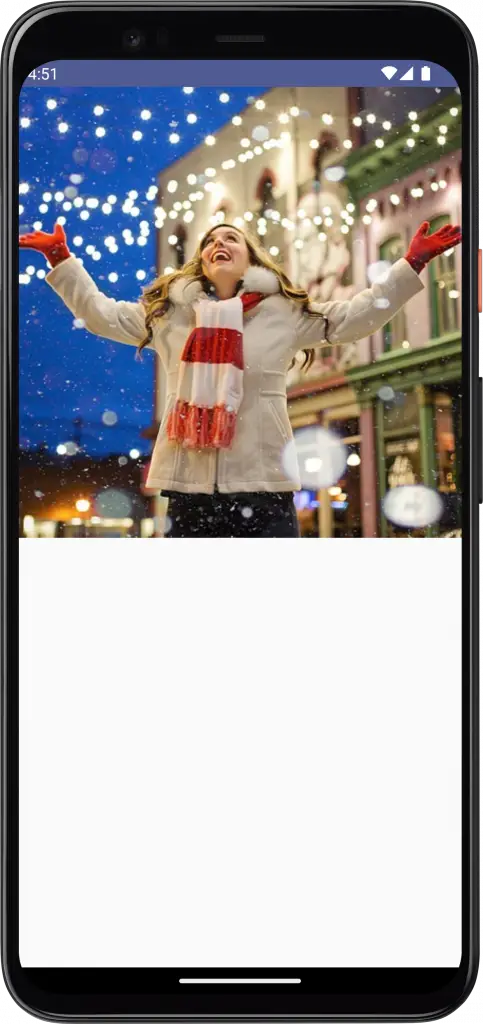
That’s how you center crop images in Jetpack Compose.