How to Blur an Image in Android Jetpack Compose
Sometimes, you may want to blur images in your mobile app. In this Android tutorial, let’s learn how to blur an image easily in Jetpack Compose.
Most of the composables including the Image have a modifier parameter and we can use the Modifier.blur to add a blur effect to the images. See the following code snippet.
Image(painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
contentScale = ContentScale.Crop,
modifier = Modifier.fillMaxSize().blur(
radiusX = 5.dp,
radiusY = 5.dp,
edgeTreatment = BlurredEdgeTreatment(RoundedCornerShape(0.dp))
)
)
NB: The blur effect is only supported on Android 12 and above. Attempts to use this Modifier on older Android versions are ignored.
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.BlurredEdgeTreatment
import androidx.compose.ui.draw.blur
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
Image(painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
contentScale = ContentScale.Crop,
modifier = Modifier.fillMaxSize().blur(
radiusX = 5.dp,
radiusY = 5.dp,
edgeTreatment = BlurredEdgeTreatment(RoundedCornerShape(0.dp))
)
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
You will get the following output of a blurred image.
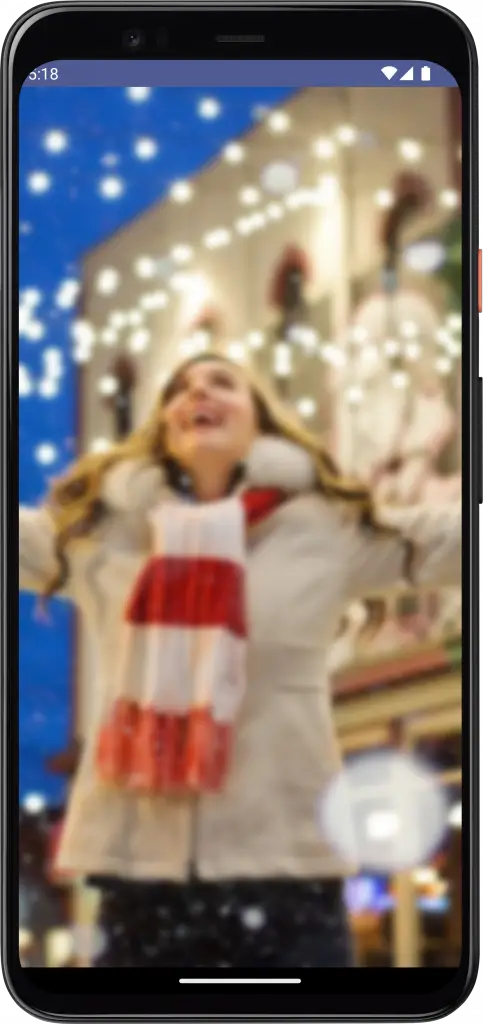
That’s how you blur an image in Jetpack Compose.