How to change Image Brightness in Android Jetpack Compose
Sometimes, you may want to tweak the default brightness of images in your Android app. In this blog post, let’s learn how to change the brightness of an image in Jetpack Compose.
Before proceeding, the following is the image I am going to use in this tutorial.
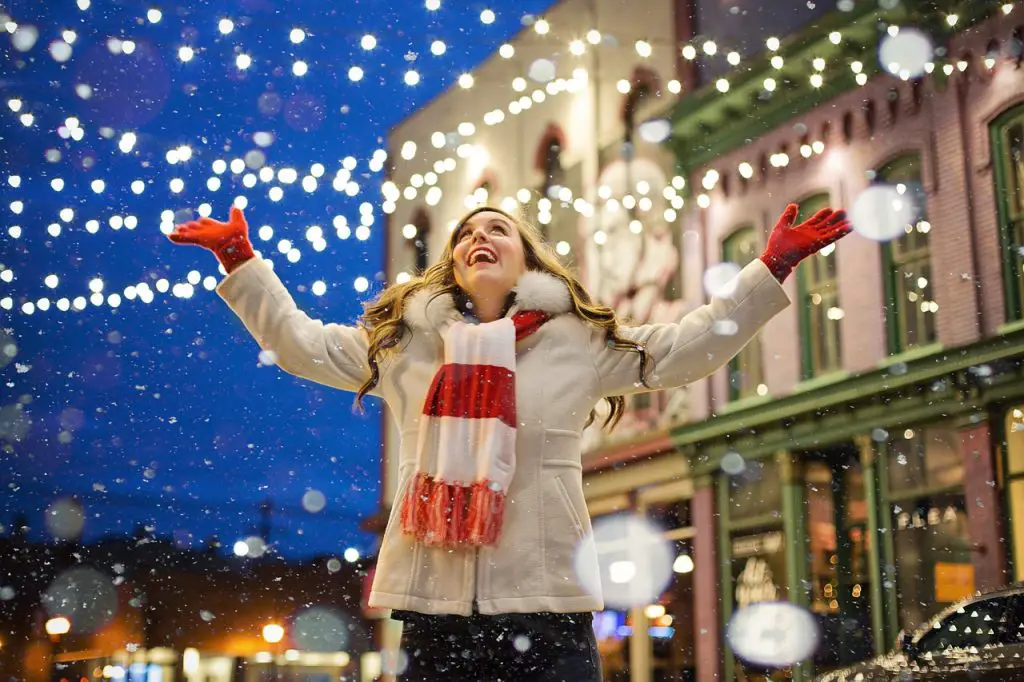
The Image composable has many useful parameters and colorFilter is one among them. We can alter the default brightness using ColorMatrix.
See the code snippet given below.
Image(
painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix().apply{setToScale(1.5f,1.5f,1.5f,1f)}),
modifier = Modifier.fillMaxSize()
)
Here, we used setToScale method. This method takes four parameters: red, green, blue, and alpha. To increase the brightness of the image, you can set each color component to a value greater than 1.
Setting the scale of each color component to 1.5 will increase the brightness of the image by 50%.
The output is given below.
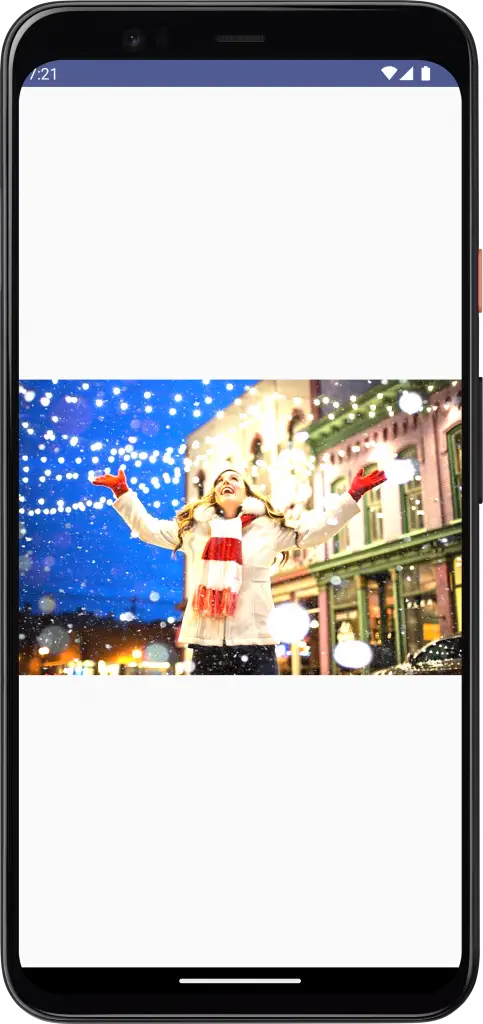
Similarly, you can decrease the brightness by giving a value of less than 1.
Image(
painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix().apply{setToScale(0.5f,0.5f,0.5f,1f)}),
modifier = Modifier.fillMaxSize()
)
You will get a dark image as the output.
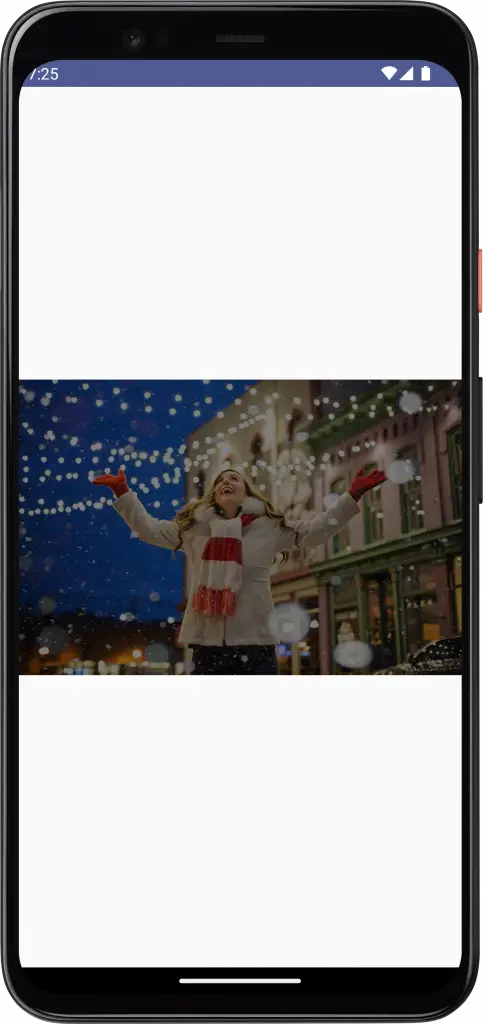
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.graphics.ColorMatrix
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ImageExample()
}
}
}
}
}
@Composable
fun ImageExample() {
Image(
painter = painterResource(id = R.drawable.christmas),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix().apply{setToScale(0.5f,0.5f,0.5f,1f)}),
modifier = Modifier.fillMaxSize()
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
ImageExample()
}
}
That’s how you change image brightness in Jetpack Compose.