How to Draw Line using Canvas in Android Jetpack Compose
Compose’s Canvas component serves as the foundation for creating custom graphics. It is integrated into the layout like any other Compose UI element. With Canvas, you have precise control over the appearance and positioning of the elements you draw.
In this blog post, let’s learn how to draw a line using Canvas in Jetpack Compose.
See the code snippet given below.
Jetpack Compose Canvas Horizontal Line
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawLine(
start = Offset(x = 0f, y = canvasHeight/2),
end = Offset(x = canvasWidth, y = canvasHeight/2),
strokeWidth = 5f,
color = Color.Blue
)
}
Inside the Canvas, it declares two variables canvasWidth and canvasHeight, and assigns them to the width and height of the Canvas. These values can be used later to position elements on the Canvas.
Then, it calls the drawLine function provided by the Canvas class. This function is used to draw a line on the Canvas. Here, it takes four parameters:
- start: The starting point of the line, represented by an Offset object. The x and y properties of the Offset are set to 0f and canvasHeight/2 respectively, which positions the line’s starting point at the left side of the Canvas and in the middle of the height.
- end: The ending point of the line, represented by an Offset object. The x and y properties of the Offset are set to canvasWidth and canvasHeight/2 respectively, which positions the line’s ending point at the right side of the Canvas and in the middle of the height.
- strokeWidth: The width of the line is set to 5f.
- color: The color of the line is set to blue.
So, this code will draw a blue horizontal line in the middle of the canvas, with a width of 5F.
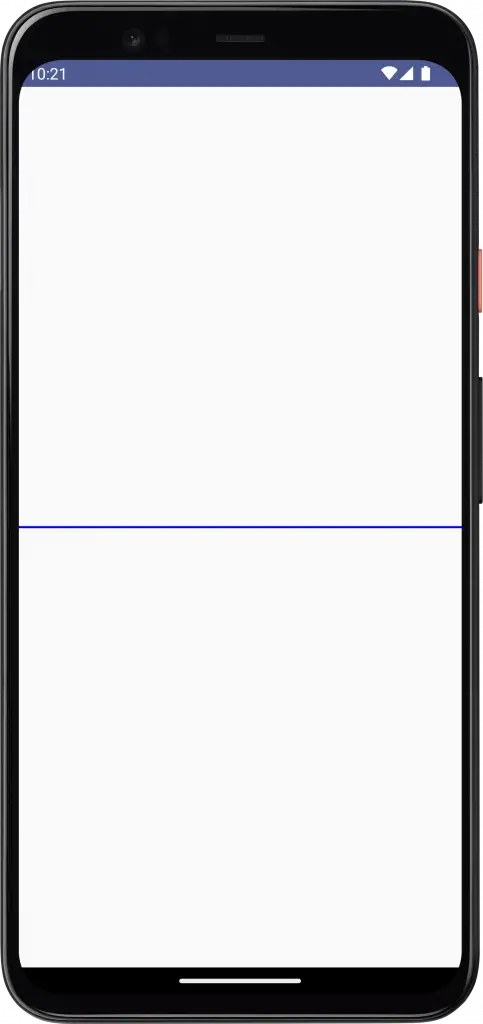
Jetpack Compose Canvas Vertical Line
You can draw the vertical line using Canvas as given below.
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawLine(
start = Offset(x = canvasWidth/2, y = 0f),
end = Offset(x = canvasWidth/2, y = canvasHeight),
strokeWidth = 5f,
color = Color.Blue
)
And you will get the following output.
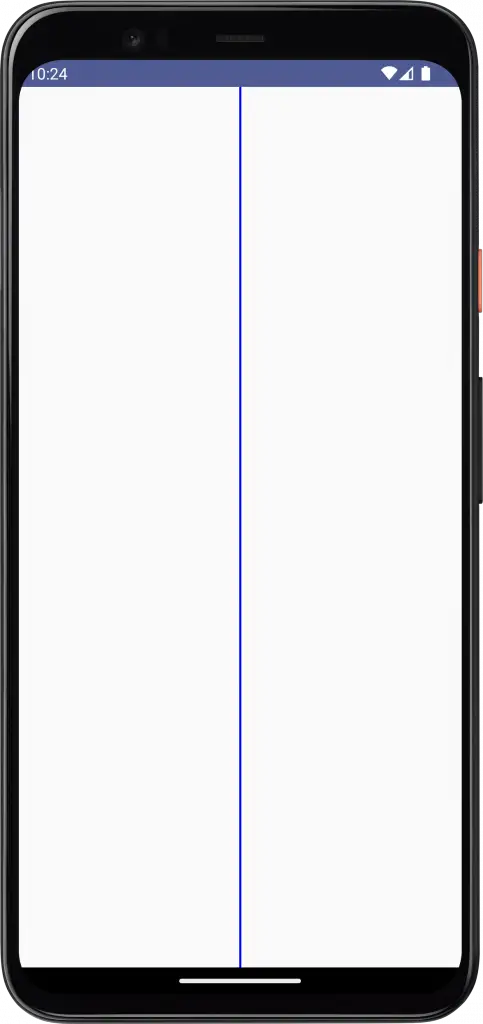
Jetpack Compose Canvas Diagonal Line
You can try various configurations to get the desired result. For example, the following code snippet gives you a diagonal line as output.
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawLine(
start = Offset(x = canvasWidth, y = 0f),
end = Offset(x = 0f, y = canvasHeight),
strokeWidth = 5f,
color = Color.Blue
)
}
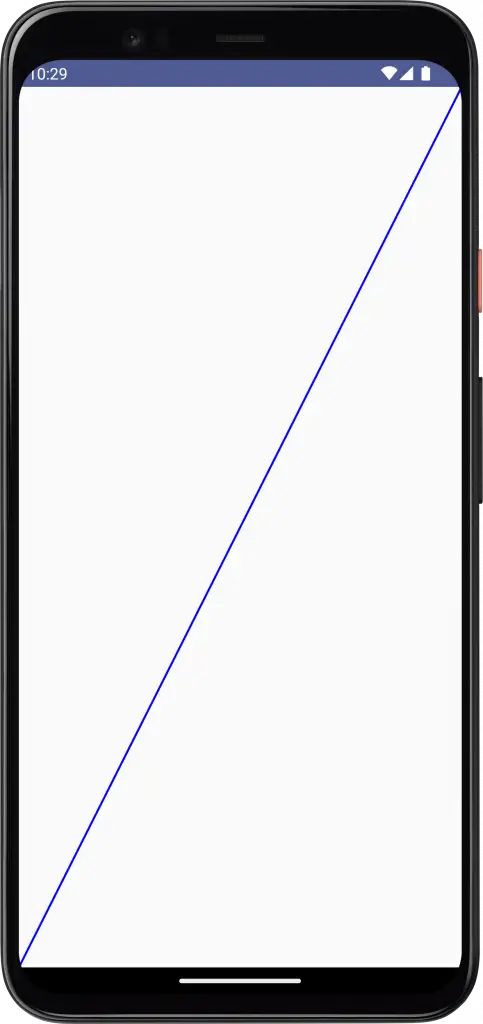
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Canvas
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CanvasExample()
}
}
}
}
}
@Composable
fun CanvasExample() {
Canvas(modifier = Modifier.fillMaxSize()) {
val canvasWidth = size.width
val canvasHeight = size.height
drawLine(
start = Offset(x = canvasWidth, y = 0f),
end = Offset(x = 0f, y = canvasHeight),
strokeWidth = 5f,
color = Color.Blue
)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
CanvasExample()
}
}
That’s how you draw lines using Canvas in Jetpack Compose.