How to Set Box Background Color in Android Jetpack Compose
Jetpack Compose, the UI toolkit from Google, aims to make UI development easier and more enjoyable. It employs a declarative style and removes much of the boilerplate traditionally involved in Android UI development.
This blog post will dive into how to set the background color to the Box composable in Jetpack Compose.
The Box Composable
Before we proceed with changing the background color, let’s first understand the Box composable. In Jetpack Compose, Box is a predefined composable that is used to layer its children. It allows you to stack multiple composables, with the first composable as the base and the rest layered on top of it.
It’s a simple and flexible way to design your UI elements and provides the ability to easily align and position your child composables. Now, let’s look at how to change the background color of this versatile Box composable.
How to Set Background Color
The Modifier parameter is key to adjusting and customizing composables in Jetpack Compose. This includes setting the background color for the Box composable. The Modifier.background function can be used to set the background color. Here’s a simple example:
@Composable
fun BoxExample() {
Column{
Box(
modifier = Modifier
.fillMaxWidth().height(200.dp)
.background(Color.Magenta),
contentAlignment = Alignment.Center
) {
Text(
text = "Coding with Rashid",
)
}
}
}
In the above code, we’ve applied the background function to the Modifier of the Box composable. This function sets the background color of the Box to the color specified in the parameter. In our case, it’s Color.Magenta.
Following is the output.
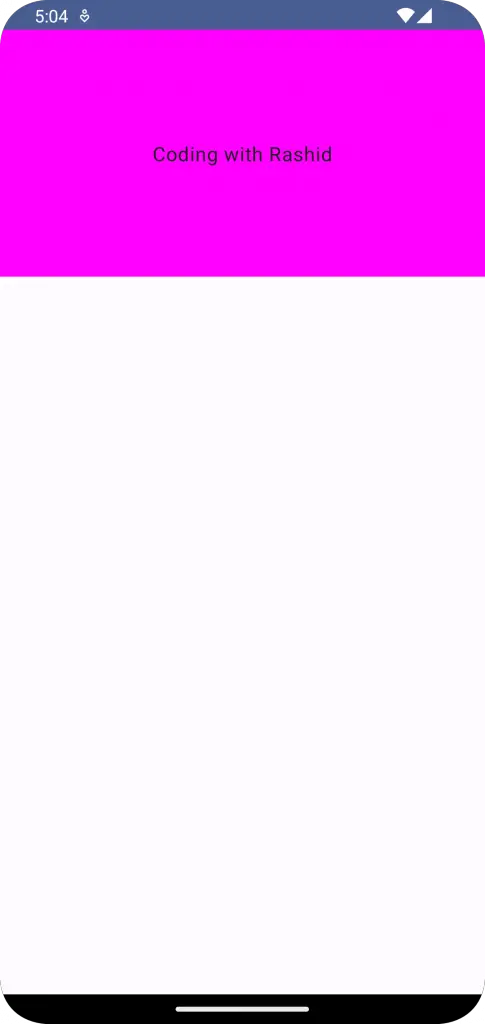
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
BoxExample()
}
}
}
}
}
@Composable
fun BoxExample() {
Column{
Box(
modifier = Modifier
.fillMaxWidth().height(200.dp)
.background(Color.Magenta),
contentAlignment = Alignment.Center
) {
Text(
text = "Coding with Rashid",
)
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
BoxExample()
}
}
In this tutorial, we’ve explored how to set a background color for the Box composable in Jetpack Compose using the Modifier.background function. Jetpack Compose makes it incredibly easy and straightforward to customize UI elements according to your requirements.