How to add Icon Toggle Button in Android Jetpack compose
Toggle buttons play a significant role in mobile applications by allowing users to change a setting between two states. When combined with icons, they become more intuitive and visually appealing. In this blog post, we will delve into creating an icon toggle button in Jetpack Compose.
What is an Icon Toggle Button?
An icon toggle button is a button that alters its state and icon when tapped. For instance, a common use case is a favorite button that toggles between a filled and an outlined heart icon.
The IconToggleButton Composable
Jetpack Compose provides the IconToggleButton composable which can represent two states and react to click events.
Here is a simple usage of the IconToggleButton:
IconToggleButton(
checked = liked.value,
onCheckedChange = { liked.value = it }) {
Icon(
modifier = Modifier.size(78.dp),
imageVector = if (liked.value) Icons.Default.Favorite else Icons.Default.FavoriteBorder,
contentDescription = null
)
}
IconToggleButton is a composable that displays an icon and responds to click events, essentially functioning as a button that can be toggled on or off.
The checked parameter of IconToggleButton indicates the current state of the button. liked.value is a state variable, with true indicating the “liked” state and false indicating the “not liked” state. This state determines the appearance of the icon as well as the action when the button is clicked.
The onCheckedChange is a callback that is triggered when the button is clicked. The function { liked.value = it } is a function that updates the liked state based on the new state of the button (it).
The Icon composable inside the IconToggleButton is the icon that is displayed. It changes between Icons.Default.Favorite (a filled heart icon) and Icons.Default.FavoriteBorder (an outlined heart icon) based on the liked.value state.
Following is the output.
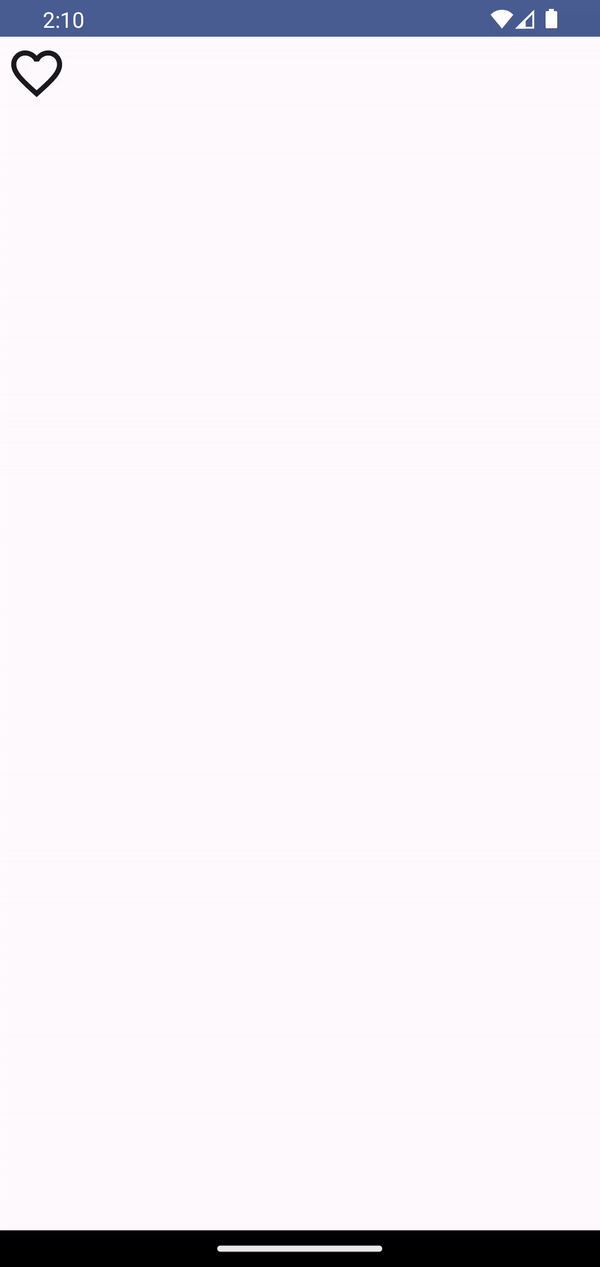
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.size
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.Favorite
import androidx.compose.material.icons.filled.FavoriteBorder
import androidx.compose.material3.Icon
import androidx.compose.material3.IconToggleButton
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
IconExample()
}
}
}
}
}
@Composable
fun IconExample() {
Column{
val liked = remember { mutableStateOf(false) }
IconToggleButton(
checked = liked.value,
onCheckedChange = { liked.value = it }) {
Icon(
modifier = Modifier.size(78.dp),
imageVector = if (liked.value) Icons.Default.Favorite else Icons.Default.FavoriteBorder,
contentDescription = null
)
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
IconExample()
}
}
Icon toggle buttons are a great way to provide an interactive element in your app’s UI, enhancing usability and visual appeal. Jetpack Compose, with its wide range of composables like IconToggleButton, empowers developers to create dynamic and responsive UIs with relative ease.