How to Add Shadows to Text in Android Jetpack Compose
Shadows can be a great way to add visual depth to your UI, and Jetpack Compose makes it easy to apply them to your text. In this Android tutorial, let’s learn how to add shadows to Text in Jetpack Compose.
You can use the shadow parameter of the Text and then apply a shadow using the Shadow class for this purpose. See the code snippet given below.
@Composable
fun TextShadow() {
Text(
text = "Coding with Rashid!",
style = TextStyle(
fontSize = 44.sp,
shadow = Shadow(
color = Color.Red,
offset = Offset(2.0f, 5.0f),
blurRadius = 2f
)
)
)
}
TextShadow is a Composable function in Jetpack Compose that displays a text with a shadow effect. It uses the Text composable function to display the text on the screen. The text parameter is set to “Coding with Rashid!” to display that text.
The style parameter is used to set the style of the text, including the font size and the shadow effect. The TextStyle object is created with a font size of 44 sp and a Shadow object. The Shadow object is created with three parameters: color, offset, and blurRadius.
The color parameter is set to Color.Red to create a red shadow. The offset parameter is set to Offset(2.0f, 5.0f) to shift the shadow 2.0 pixels to the right and 5.0 pixels down from the text.
The blurRadius parameter is set to 2f to create a blur radius of 2 pixels. The larger the blur radius, the more blurred the shadow will appear.
Following is the output.
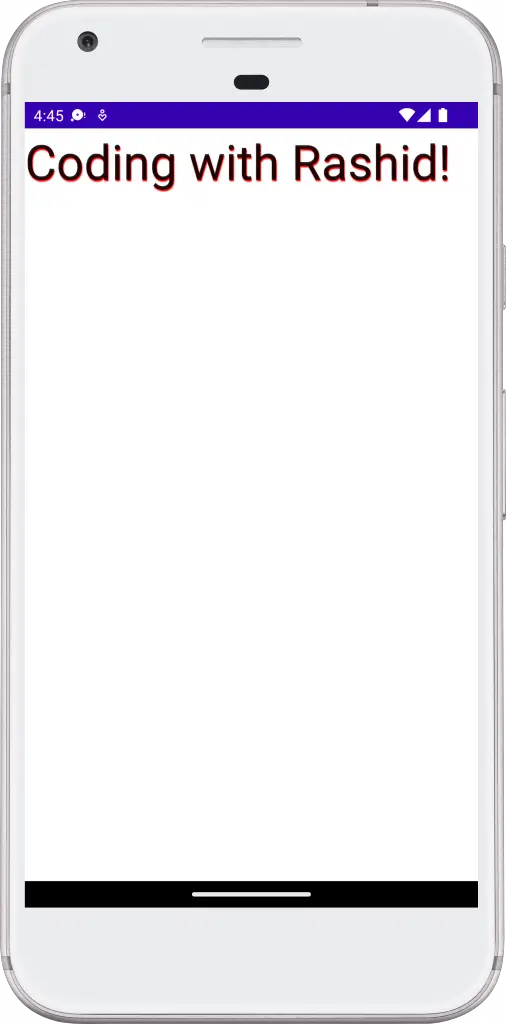
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.Shadow
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
TextShadow()
}
}
}
}
}
@Composable
fun TextShadow() {
Text(
text = "Coding with Rashid!",
style = TextStyle(
fontSize = 44.sp,
shadow = Shadow(
color = Color.Red,
offset = Offset(2.0f, 5.0f),
blurRadius = 2f
)
)
)
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
TextShadow()
}
}
That’s how you add text with shadows in Jetpack Compose.
If you want to style text with gradients then see this Jetpack Compose gradient text tutorial.