How to Set Card Elevation in Android Jetpack Compose
Jetpack Compose, the modern, declarative UI toolkit from Android, offers a range of components to build flexible and beautiful UIs. One such component is Card, which is an essential element in Material Design.
It allows you to encapsulate related pieces of information in a neatly arranged manner, making it easier for users to understand the data. A major aspect of the Card composable that can greatly enhance the user interface’s depth and dimension is elevation.
This blog post will guide you on how to work with card elevation in Jetpack Compose.
What is Elevation?
The elevation is a concept from Material Design that allows you to define the visual hierarchy of your UI by raising elements in your layout. It essentially allows a Card (or any other elevated view) to cast a shadow, providing the perception of depth within the UI.
The higher the elevation level, the bigger the shadow, creating the impression that the element is raised above the rest of the UI.
How to Add Card Elevation in Jetpack Compose
Jetpack Compose with Material You (Material 3) presents a slightly different approach to handle card elevation as compared to Material 2. The default Card composable now has a cardElevation parameter in the CardDefaults function to set the elevation.
Here is an example of how to use it:
Card(
modifier = Modifier.fillMaxWidth().padding(20.dp),
elevation = CardDefaults.cardElevation(
defaultElevation = 8.dp
)
) {
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
In the code snippet above, the Card composable has an elevation of 10.dp. This will create a shadow effect that makes the card appear elevated from the rest of the UI.
Following is the output.
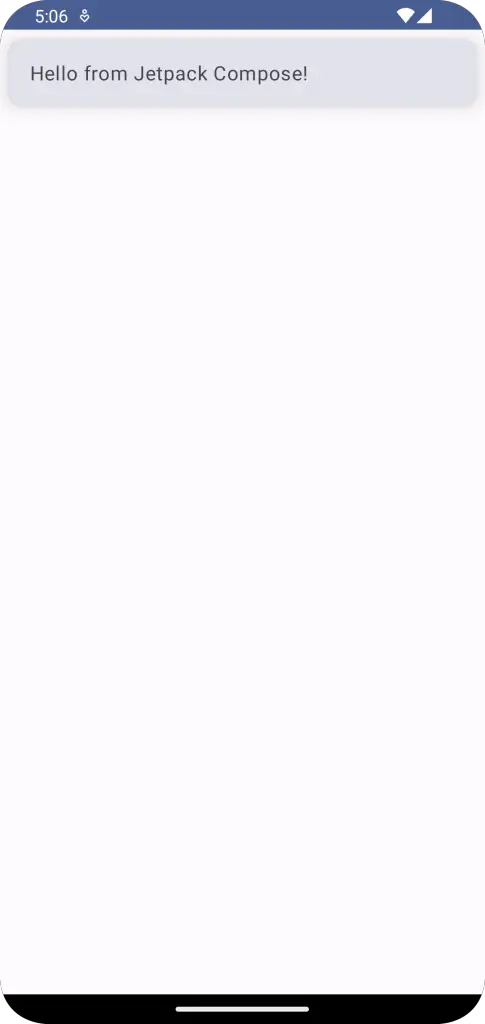
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.Card
import androidx.compose.material3.CardDefaults
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CardExample()
}
}
}
}
}
@Composable
fun CardExample() {
Column {
Card(
modifier = Modifier.fillMaxWidth().padding(20.dp),
elevation = CardDefaults.cardElevation(
defaultElevation = 8.dp
)
) {
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
CardExample()
}
}
In Jetpack Compose, using elevation with the Card composable provides a sense of depth and hierarchy to your UI. With the above guide, you should be able to utilize the cardElevation function to enrich your user interface effectively.