How to Create Linear Gradient in Android Jetpack Compose
In modern UI design, gradients can bring depth, volume, and vibrancy, transforming your app interface from plain and simple to dynamic and lively. In Android development, this process has been simplified with Jetpack Compose.
Among its array of features, one of the most visually appealing is the ability to create gradient backgrounds. This blog post focuses on the creation and usage of linear gradients in Jetpack Compose.
How to Define Linear Gradient
Linear gradients transition colors along a straight line. With Jetpack Compose, linear gradients can be created using the Brush.linearGradient function. This function takes a list of color-stop pairs or a list of colors and optionally, the start and end points for the gradient.
Here’s an example of a linear gradient transitioning from blue to green:
val gradient = Brush.linearGradient(
colors = listOf(Color.Blue, Color.Green)
)
In the above example, the gradient starts from blue and transitions to green.
The colors in the gradient are dispersed along the line defined by the start and end points. When no points are specified, the gradient is drawn from the top-left to the bottom-right of the component.
You can also customize the gradient’s start and end points:
val gradient = Brush.linearGradient(
colors = listOf(Color.Blue, Color.Green)
start = Offset(0f, 0f),
end = Offset(1000f, 0f)
)
In this snippet, the gradient starts from the left (Offset(0f, 0f)) and ends to the right (Offset(1000f, 0f)).
Moreover, you can specify how each color in your gradient should be dispersed along the line by using color-stop pairs:
val gradient = Brush.linearGradient(
colorStops = arrayOf(
0.0f to Color.Red,
0.3f to Color.Green,
1.0f to Color.Blue
),
start = Offset(0.0f, 50.0f),
end = Offset(0.0f, 100.0f)
)
In this example, we define a gradient that starts with Red at 0% (0.0f), transitions to Green at 30% (0.3f), and then to Blue at 100% (1.0f). The gradient starts and ends at the specified offsets.
How to Use Linear Gradient
After defining your gradient, you can use it in any composable that takes a Brush parameter. For example, to set the background of a Box.
@Composable
fun GradientExample() {
val linear = Brush.linearGradient(listOf(Color.Cyan, Color.Green))
Column() {
Box(modifier = Modifier.fillMaxWidth()
.height(100.dp)
.background(linear))
}
}
We use the Modifier.background function with our gradient to set the Box’s background.
Following is the output.
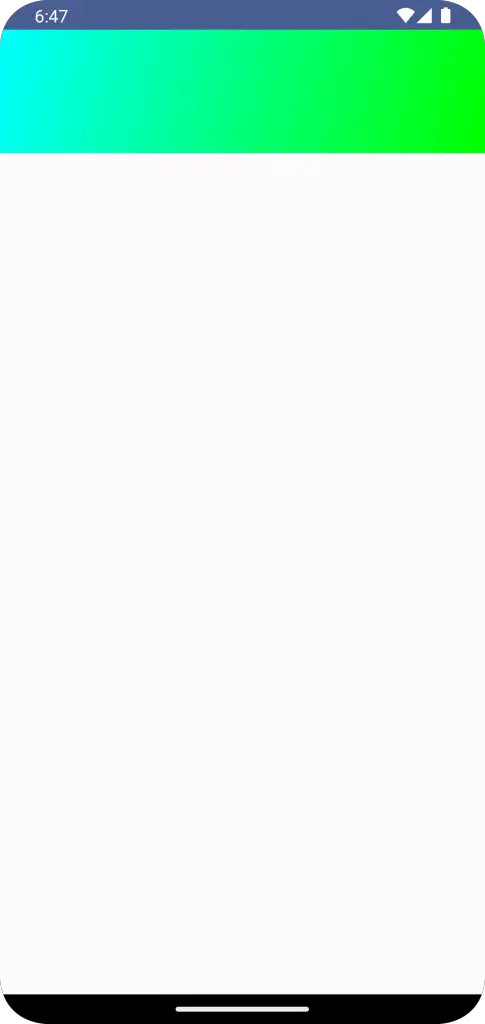
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
GradientExample()
}
}
}
}
}
@Composable
fun GradientExample() {
val linear = Brush.linearGradient(listOf(Color.Cyan, Color.Green))
Column() {
Box(modifier = Modifier.fillMaxWidth()
.height(100.dp)
.background(linear))
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
GradientExample()
}
}
Creating linear gradients in Jetpack Compose is straightforward with the Brush.linearGradient function. This capability empowers developers to design visually appealing interfaces with ease.
One Comment