How to Add Space Between LazyRow Items in Android Jetpack Compose
Android Jetpack Compose is revolutionizing how we create UIs on Android. The old view-based system is making way for a more declarative and more intuitive Composable-based system.
In this blog post, we will focus on LazyRow, a Composable that can be used to create horizontal lists. Specifically, we’ll discuss how to add space between items in a LazyRow using the horizontalArrangement parameter.
We can add space between items using Arrangement.spacedBy(). This arranges the children with equal spacing between them. This spacing can be specified using any unit of measurement, but we’ll use density-independent pixels (dp) to ensure our UI looks consistent across devices with different screen densities.
@Composable
fun HorizontalListView() {
val items = (1..50).toList()
LazyRow(horizontalArrangement = Arrangement.spacedBy(32.dp)) {
items(items) { item ->
Text(
text = "Item $item",
modifier = Modifier
.background(Color.Gray)
.padding(20.dp)
)
}
}
}
We used Arrangement.spacedBy(32.dp) in the LazyRow Composable, which adds a 32.dp space between each item in the horizontal list.
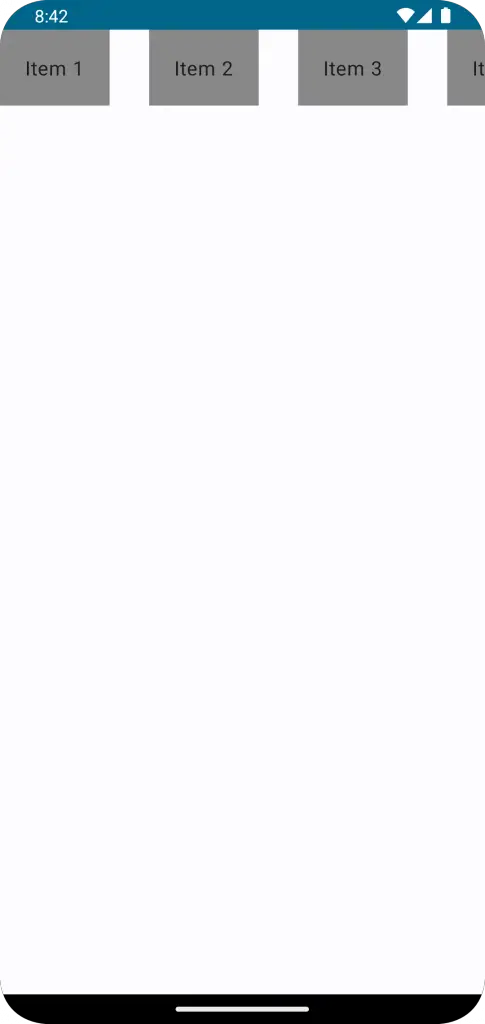
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.lazy.items
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
import androidx.compose.foundation.lazy.LazyRow
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
HorizontalListView()
}
}
}
}
}
@Composable
fun HorizontalListView() {
val items = (1..50).toList()
LazyRow(horizontalArrangement = Arrangement.spacedBy(32.dp)) {
items(items) { item ->
Text(
text = "Item $item",
modifier = Modifier
.background(Color.Gray)
.padding(20.dp)
)
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
HorizontalListView()
}
}
And that’s it! You now know how to add space between LazyRow items in Android Jetpack Compose. This concept is extremely useful in creating cleaner and more readable layouts.