How to Add LazyColumn with Sticky Header in Android Jetpack Compose
Jetpack Compose, the modern UI toolkit for Android, offers an array of functionalities that make UI development smoother and more efficient. One of these tools is LazyColumn, which simplifies creating lists with efficient loading.
In this blog post, we’ll learn how to enhance our LazyColumn with a sticky header, a UI component that stays fixed at the top while users scroll through the list.
Introduction to LazyColumn
Before we delve into sticky headers, it’s beneficial to understand LazyColumn. LazyColumn in Jetpack Compose is analogous to RecyclerView in traditional Android development. It loads and displays a large quantity of data efficiently by rendering only those items that are currently in the viewport.
What is a Sticky Header?
A sticky header is a type of header that stays at the top of the viewport as the user scrolls through the list until it is eventually pushed out of view by the subsequent header. This is useful in long lists, providing constant context and improving the user experience.
How to Implement a Sticky Header in LazyColumn
Now, let’s look at how we can implement a sticky header in LazyColumn. For this guide, we’ll create a simple numbered list with a single sticky header.
@OptIn(ExperimentalFoundationApi::class)
@Composable
fun NumberList() {
val items = (1..100).toList()
LazyColumn {
stickyHeader {
Box(modifier = Modifier.fillMaxWidth().background(Color.LightGray)) {
Text(text = "Header", fontSize = 24.sp)
}
}
items(items) { number ->
Text(text = number.toString())
}
}
}
In this composable, we first add a stickyHeader followed by the items. The stickyHeader Composable will remain at the top of the LazyColumn as you scroll through the items.
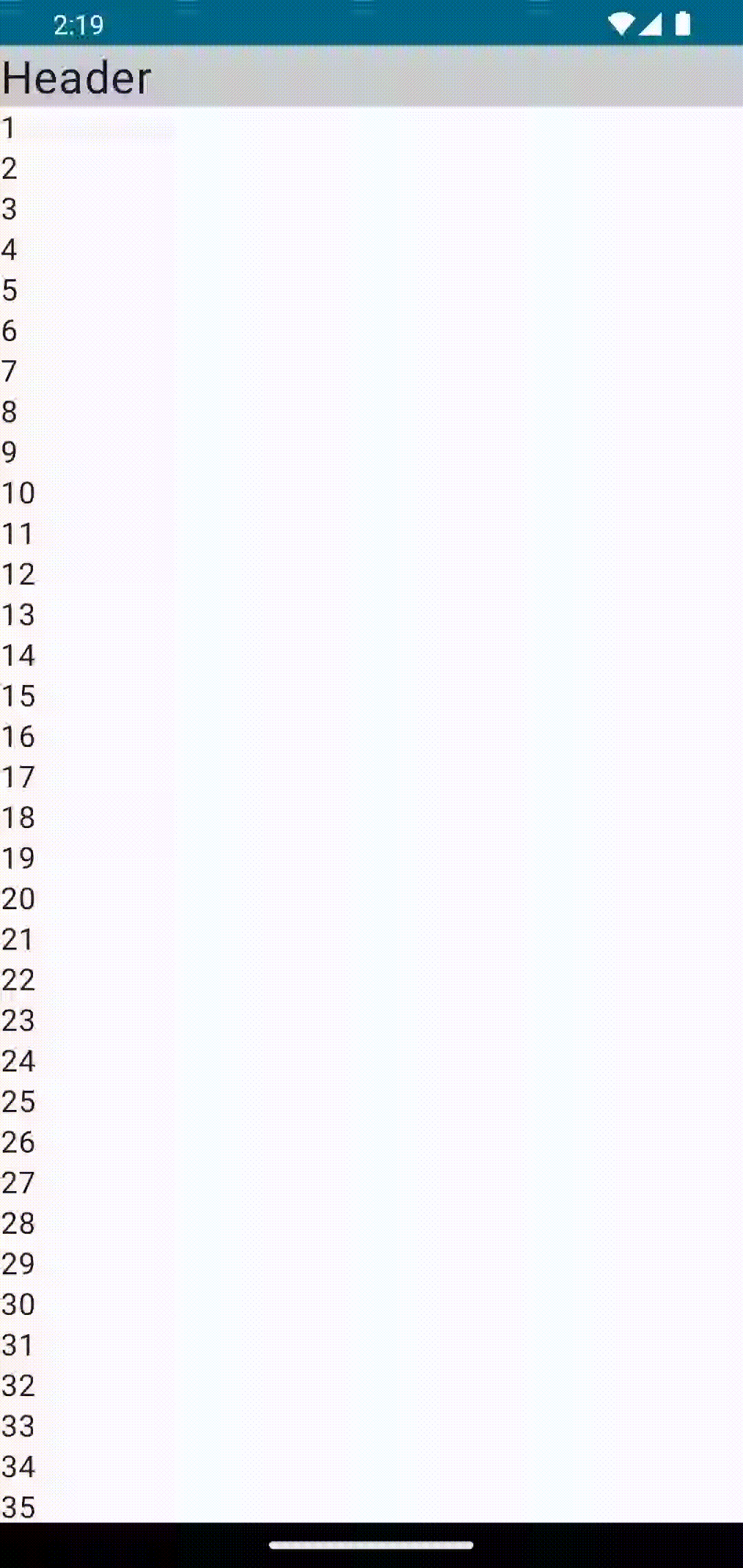
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.ExperimentalFoundationApi
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.foundation.lazy.items
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.sp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
NumberList()
}
}
}
}
}
@OptIn(ExperimentalFoundationApi::class)
@Composable
fun NumberList() {
val items = (1..100).toList()
LazyColumn {
stickyHeader {
Box(modifier = Modifier.fillMaxWidth().background(Color.LightGray)) {
Text(text = "Header", fontSize = 24.sp)
}
}
items(items) { number ->
Text(text = number.toString())
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
NumberList()
}
}
You’ve successfully implemented a sticky header in a LazyColumn with Jetpack Compose. By adding this feature, you have enhanced the user experience by providing a constant reference point.