How to Set LazyColumn Background Color in Android Jetpack Compose
In this blog post, we will discuss how to set a background color for a LazyColumn in Jetpack Compose, the toolkit for creating UIs in Android apps.
Jetpack Compose is a modern, declarative UI toolkit for Android that simplifies UI development and improves app performance. LazyColumn is one of the key components in Jetpack Compose. It’s a vertical list that only composes and lays out the visible items, making it highly efficient for displaying large datasets.
The Modifier in Jetpack Compose is a powerful tool for modifying the appearance and behavior of composables. You can use it to set a background color for a LazyColumn. Here’s an example of how to do it:
@Composable
fun NumberList() {
val items = (1..100).toList()
LazyColumn(
modifier = Modifier.background(Color.Cyan)
) {
items(items) { number ->
Text(text = number.toString(),
modifier = Modifier.fillMaxWidth())
}
}
}
In this example, Modifier.background(Color.Cyan) is used to set the background color of the LazyColumn to cyan.
This applies the cyan color to the entire background of the LazyColumn. Each Text item in the LazyColumn maintains its own background.
With the use of Modifier.background(), you can easily modify the background color of the LazyColumn and hence customize the appearance of your app.
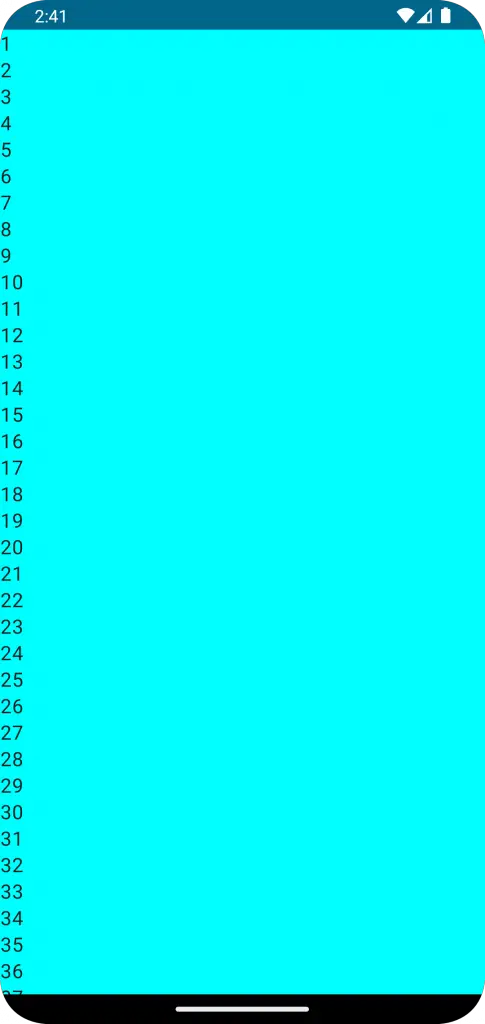
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.foundation.lazy.items
import androidx.compose.ui.graphics.Color
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
NumberList()
}
}
}
}
}
@Composable
fun NumberList() {
val items = (1..100).toList()
LazyColumn(
modifier = Modifier.background(Color.Cyan)
) {
items(items) { number ->
Text(text = number.toString(),
modifier = Modifier.fillMaxWidth())
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
NumberList()
}
}
That’s it! With this guide, you can now set a background color for LazyColumn in Jetpack Compose. Start experimenting with different colors and create unique, visually appealing lists in your app.