How to Apply Padding to LazyColumn in Android Jetpack Compose
Jetpack Compose has taken the Android UI development to a new level by offering the ability to create dynamic and flexible layouts. One such powerful layout component is LazyColumn, which is equivalent to RecyclerView in traditional Android development but with less complexity.
In this blog post, we will discuss how to apply padding in a LazyColumn. We’ll specifically focus on two types of padding – generic padding and content padding.
Generic Padding
Generic padding can be applied to the entire LazyColumn, affecting the space around the list, but not within the list items themselves. Here is a basic example of how to do this:
@Composable
fun PaddedLazyColumn() {
val items = (1..50).toList()
LazyColumn(
modifier = Modifier.run {
padding(16.dp) // Applying padding here
.background(Color.LightGray)
}
) {
items(items) { item ->
Text(text = "Item $item", modifier = Modifier.fillMaxWidth())
}
}
}
In the above code, we have a LazyColumn which displays a list of items. We have applied a padding of 16.dp to the entire column using the padding modifier. This will create a uniform 16.dp space around the whole LazyColumn.
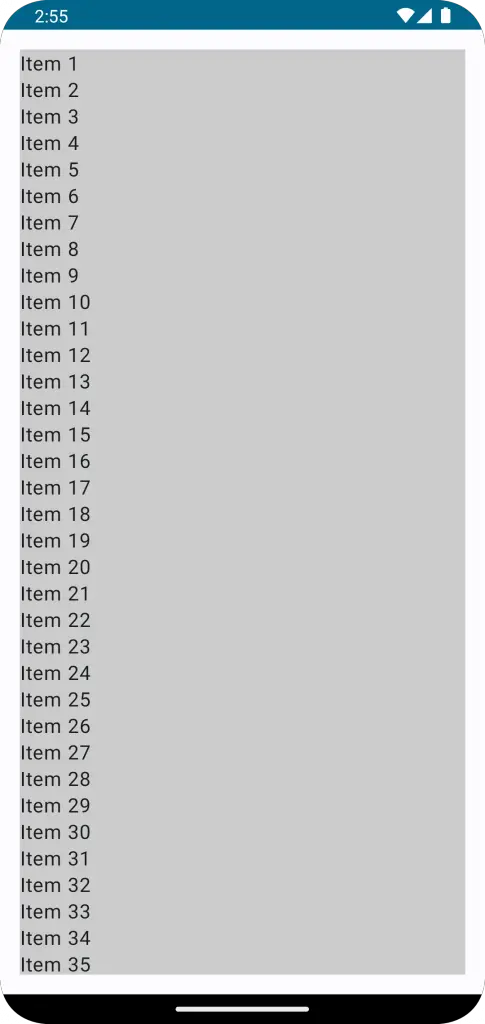
Content Padding
Content padding, on the other hand, affects the space within the list items. It provides a way to have padding between the list’s start and end and the first and last items respectively.
Here is an example of content padding:
@Composable
fun ContentPaddedLazyColumn() {
val items = (1..50).toList()
LazyColumn(
contentPadding = PaddingValues(horizontal = 16.dp, vertical = 8.dp),
modifier = Modifier.background(Color.LightGray)
) {
items(items) { item ->
Text(text = "Item $item", modifier = Modifier.fillMaxWidth())
}
}
}
In this code, we are applying padding directly to the content of our LazyColumn using the contentPadding property. We are adding horizontal padding of 16.dp and vertical padding of 8.dp. This padding will apply between the start and end of the list and the first and last items.
Note: It’s important to understand that contentPadding doesn’t affect the space between individual list items.
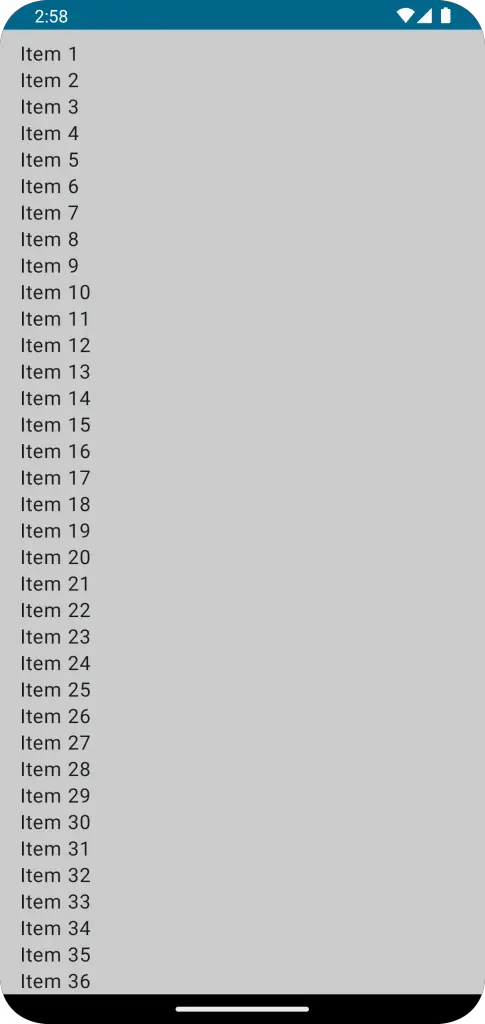
And there you have it! You now know how to add padding to a LazyColumn in Jetpack Compose. Whether you need padding around the whole list or within the list items themselves, Jetpack Compose makes it easy to enhance the look and feel of your app.