How to Set Gradient Background for Card in Android Jetpack Compose
Jetpack Compose, a declarative UI framework for Android, allows developers to build beautiful, high-quality applications with ease. One of the UI elements that you can customize to enhance the user interface of your application is a Card.
In this blog post, we’re going to learn how to create a stylish Card with a gradient background.
Introduction to Card in Jetpack Compose
In Jetpack Compose, a Card is a container used to present information related to a single subject. It is flexible and can hold any composable, which makes it one of the go-to UI elements for most developers. Now, let’s see how to make our Card more visually appealing by giving it a gradient background.
How to Apply Gradient Background to Card
To create a gradient background for a Card, we will use a combination of Card, Box, and Modifier.background that utilizes a Brush.verticalGradient or Brush.horizontalGradient. Here is how it’s done:
@Composable
fun CardExample() {
Column {
val gradient = Brush.verticalGradient(
colors = listOf(Color.Cyan, Color.Green)
)
Card(
modifier = Modifier
.fillMaxWidth()
.padding(20.dp)
) {
Box(
Modifier
.background(brush = gradient)
.height(150.dp)
.fillMaxWidth()
) {
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
}
}
}
In the above example, we first define our gradient using Brush.verticalGradient, passing in a list of colors we want in our gradient. Then, we create a Card and use a Box composable within it.The Box is given a height and is made to fill the width of the parent, with the gradient being applied as the background using the Modifier.background function.
The Text composable placed within the Box displays our content, and the entire card layout is encapsulated within a Column composable, which can be handy when you want to stack more composables vertically.
This example uses a vertical gradient. If you need a horizontal gradient, replace Brush.verticalGradient with Brush.horizontalGradient.
Following is the output.
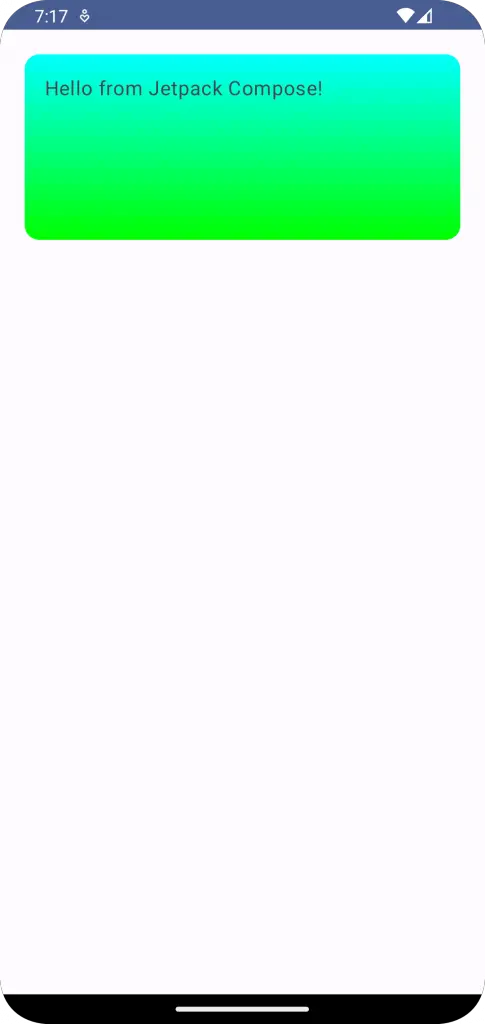
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.Card
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Brush
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CardExample()
}
}
}
}
}
@Composable
fun CardExample() {
Column {
val gradient = Brush.verticalGradient(
colors = listOf(Color.Cyan, Color.Green)
)
Card(
modifier = Modifier
.fillMaxWidth()
.padding(20.dp)
) {
Box(
Modifier
.background(brush = gradient)
.height(150.dp)
.fillMaxWidth()
) {
Text("Hello from Jetpack Compose!", Modifier.padding(16.dp))
}
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
CardExample()
}
}
Jetpack Compose makes UI customization in Android effortless and intuitive. With the ability to apply a gradient background to a Card, you can further enrich the user interface of your application, making it more visually pleasing and engaging.