How to Customize Checkbox Colors in Android Jetpack Compose
Checkboxes play a crucial role in user interface design. They offer a simple way for users to select one or more options from a set, making them instrumental in gathering user preferences, settings, and more.
However, to maintain a consistent aesthetic with the rest of your app or to emphasize their importance in the user’s decision-making process, you may want to customize the colors of your checkboxes. In this blog post, we’ll guide you on how to adjust checkbox colors in Jetpack Compose.
Jetpack Compose allows developers to create highly customizable UI elements with less code compared to traditional View-based UI. This includes checkboxes, which can have their colors changed based on their state. Here’s an example:
@Composable
fun CheckboxExample() {
Column() {
var checked by remember { mutableStateOf(false) }
Checkbox(
checked = checked,
onCheckedChange = { checked = it },
colors = CheckboxDefaults.colors(
checkedColor = Color.Red,
uncheckedColor = Color.Blue,
checkmarkColor = Color.White
)
)
}
}
In the above code, we’ve created a Checkbox composable whose colors change based on its checked state. We use CheckboxDefaults.colors to specify the color when the checkbox is checked (checkedColor), the color when it’s not checked (uncheckedColor), and the color of the checkmark (checkmarkColor).
The checked variable dictates whether the checkbox is selected or not. Clicking on the checkbox will trigger onCheckedChange, causing the checked state to update. Jetpack Compose’s state-driven design ensures the UI updates to reflect this new state.
Following is the output.
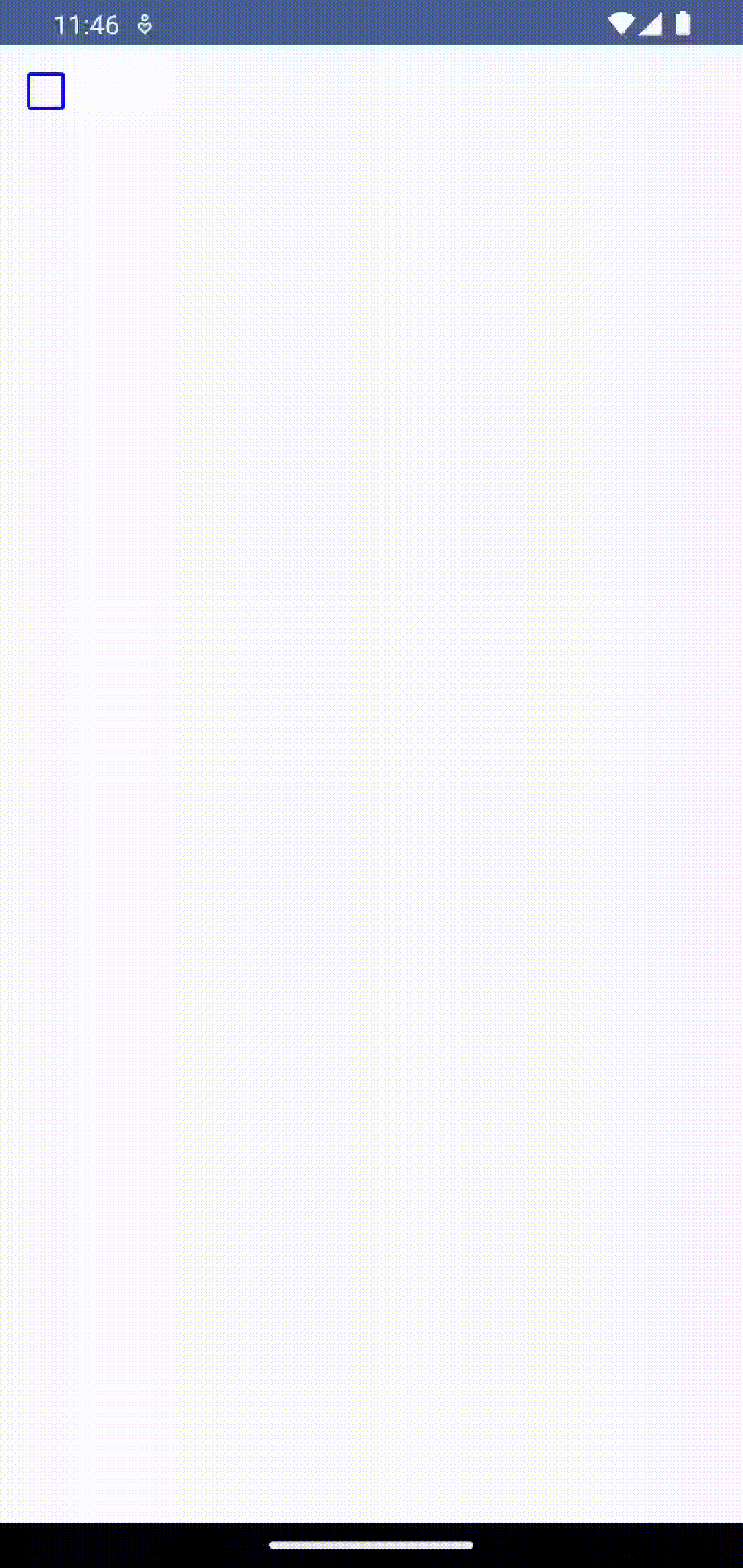
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.Checkbox
import androidx.compose.material3.CheckboxDefaults
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CheckboxExample()
}
}
}
}
}
@Composable
fun CheckboxExample() {
Column {
var checked by remember { mutableStateOf(false) }
Checkbox(
checked = checked,
onCheckedChange = { checked = it },
colors = CheckboxDefaults.colors(
checkedColor = Color.Red,
uncheckedColor = Color.Blue,
checkmarkColor = Color.White
)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
CheckboxExample()
}
}
Remember that good design is about more than just making your app look good. It’s also about creating an intuitive experience for your users. Consistently using colors for active and inactive states can go a long way in helping users understand how to interact with your app.
With Jetpack Compose, Android developers can create beautiful, customized UI with ease, and checkboxes are just the beginning.
One Comment