How to Add Checkbox in Android Jetpack Compose
Checkboxes are fundamental UI components used to enable users to make a binary choice. In Android’s Jetpack Compose, adding a checkbox is easier than you might think. This tutorial aims to guide you through the process of integrating a simple checkbox using Jetpack Compose.
The Checkbox Composable
Before diving into the example, let’s get a basic understanding of the Checkbox
composable. The primary parameters it takes are checked
and onCheckedChange
.
The checked
parameter is a Boolean value that represents the current state of the checkbox. The onCheckedChange
parameter is a lambda function that gets triggered whenever the checkbox state changes.
A Simple Checkbox Example
To help you understand better, here’s an example of how to create a simple checkbox in Jetpack Compose.
@Composable
fun CheckboxExample() {
var checkedState by remember { mutableStateOf(false) }
Checkbox(
checked = checkedState,
onCheckedChange = { checkedState = it },
colors = CheckboxDefaults.colors(Color.Red)
)
}
Code Explanation
var checkedState by remember { mutableStateOf(false) }
: This line initializes the state for our checkbox and sets it to false initially.Checkbox(...)
: This is the composable that creates our checkbox.colors = CheckboxDefaults.colors(Color.Red)
: This sets the color of the checkbox to red.
Complete Code.
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.Checkbox
import androidx.compose.material3.CheckboxDefaults
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
CheckboxExample()
}
}
}
}
}
}
@Composable
fun CheckboxExample() {
var checkedState by remember { mutableStateOf(false) }
Checkbox(
checked = checkedState,
onCheckedChange = { checkedState = it },
colors = CheckboxDefaults.colors(Color.Red)
)
}
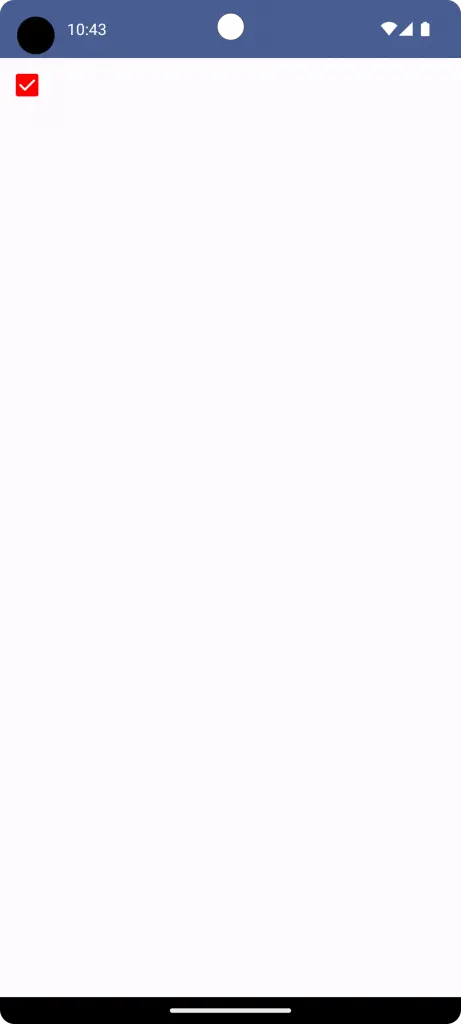
How to Use Checkbox State
Since checkedState
is a mutable state variable, you can use it in other composables or even for triggering certain actions based on its value. For example, you can display text that reflects the checkbox’s state like so:
Text(text = if (checkedState) "Checkbox is checked" else "Checkbox is not checked")
Customize the Checkbox
Jetpack Compose allows you to customize checkboxes further by adjusting attributes like color, size, and more. For example, in the Checkbox
composable code above, we changed the color to red by using the colors
parameter.
Adding a checkbox in Jetpack Compose is a simple yet powerful way to gather binary input from users. You can customize the checkbox’s appearance and behavior according to your application’s requirements.
The example provided should serve as a good starting point for integrating checkboxes into your Android applications built with Jetpack Compose.