How to Add Image Border in Android Jetpack Compose
Borders add a touch of refinement to your UI elements, making them stand out. In this updated tutorial, we’ll delve deeper into how to wrap an image with a border using Android Jetpack Compose.
What is Jetpack Compose?
Before we dive in, it’s good to understand what Jetpack Compose is. Jetpack Compose is Android’s modern toolkit for building native UI. It simplifies and accelerates UI development on Android.
Image with Border
The Image
composable is key to displaying images on your screen. To add a border, you can use the border Modifier
. Here is an example with the code.
@Composable
fun ImageExample() {
Image(
painter = painterResource(R.drawable.pet),
contentDescription = null,
contentScale = ContentScale.Crop,
modifier = Modifier
.size(250.dp)
.padding(20.dp)
.border(3.dp, Color.Red)
)
}
We have given the thickness and color of the border using the border modifier. Like this, you can also add borders to circular images.
Complete Code Example
Here’s the full code to include the ImageExample
composable within your Android app.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.border
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.size
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
ImageExample()
}
}
}
}
}
}
@Composable
fun ImageExample() {
Image(
painter = painterResource(R.drawable.pet),
contentDescription = null,
contentScale = ContentScale.Crop,
modifier = Modifier
.size(250.dp)
.padding(20.dp)
.border(3.dp, Color.Red)
)
}
Output
Once you run this code, you’ll see an image of a pet with a red border. It’s a simple yet effective way to make your image pop.
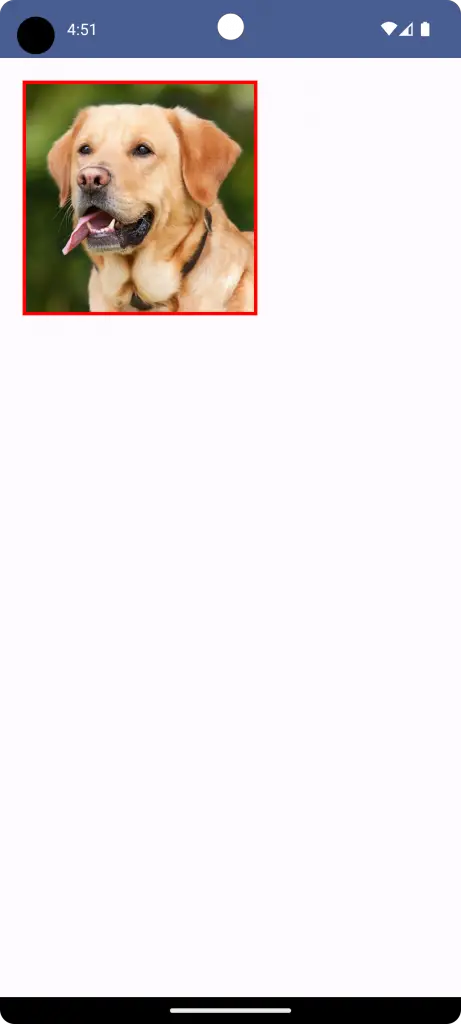
Adding borders to your images in Jetpack Compose is pretty straightforward. By using the border Modifier
function along with the Image
composable, you can easily give your images a decorative edge.