How to Capitalize TextField in Android Jetpack Compose
In Android development, the TextField is a crucial element for user input. There are times when you might want to limit the input to uppercase letters. This tutorial explains how to capitalize the text in a TextField using Jetpack Compose.
Use KeyboardOptions for Capitalization
Jetpack Compose offers a KeyboardOptions
parameter within the TextField
composable. This allows you to manage different keyboard features, such as text capitalization.
By setting the KeyboardCapitalization
attribute, you can control whether to capitalize characters, words, or even sentences.
Here’s a code snippet that demonstrates how to capitalize text within a TextField
.
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(
value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Username") },
keyboardOptions = KeyboardOptions(capitalization = KeyboardCapitalization.Characters),
modifier = Modifier.fillMaxWidth().padding(10.dp)
)
}
How Does It Work?
In this example, we define a TextField
composable function. Inside this function:
var textInput by remember { mutableStateOf("")}
initializes a mutable state variable.TextField()
is the UI element where the user can input text.keyboardOptions = KeyboardOptions(capitalization = KeyboardCapitalization.Characters)
sets the keyboard to capitalize characters.
Complete CodeExample
For a complete reference, let’s include the main activity and other related elements.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.text.KeyboardOptions
import androidx.compose.material3.ExperimentalMaterial3Api
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.input.KeyboardCapitalization
import androidx.compose.ui.unit.dp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextFieldExample()
}
}
}
}
}
}
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun TextFieldExample() {
var textInput by remember { mutableStateOf("")}
TextField(value = textInput,
onValueChange = {textInput = it},
placeholder = { Text("Username") },
keyboardOptions = KeyboardOptions(capitalization = KeyboardCapitalization.Characters),
modifier = Modifier.fillMaxWidth()
.padding(10.dp))
}
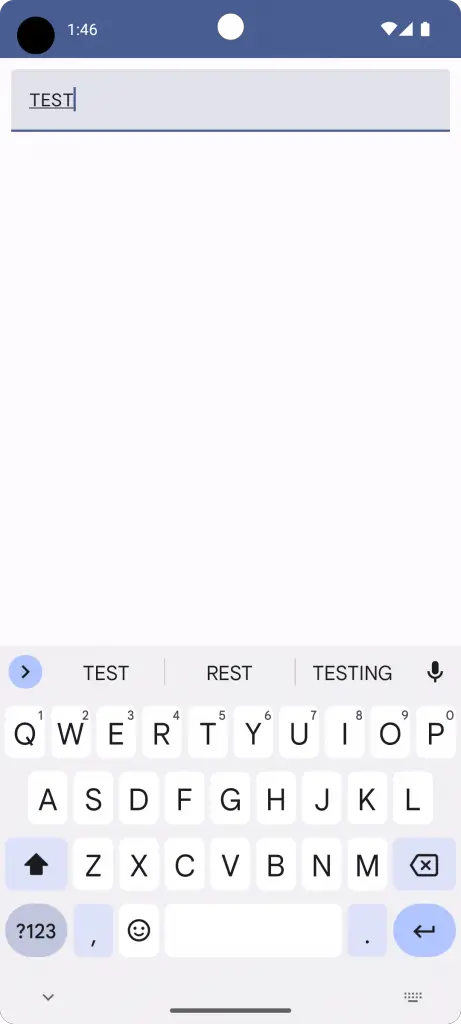
The code makes it straightforward to enforce capital letters in a TextField. By using Jetpack Compose’s KeyboardOptions
and KeyboardCapitalization
features, you can easily control the text input behavior.