How to make Text Italic in Android Jetpack Compose
As a mobile app developer, you always want to style text appropriately. Let’s check how to make a text italic in Android using Jetpack Compose.
The FontStyle help to make the Text composable italic.
@Composable
fun TextExample() {
Text("Italic Text Example",
fontSize = 24.sp,
fontStyle = FontStyle.Italic
)
}
Following is the output.
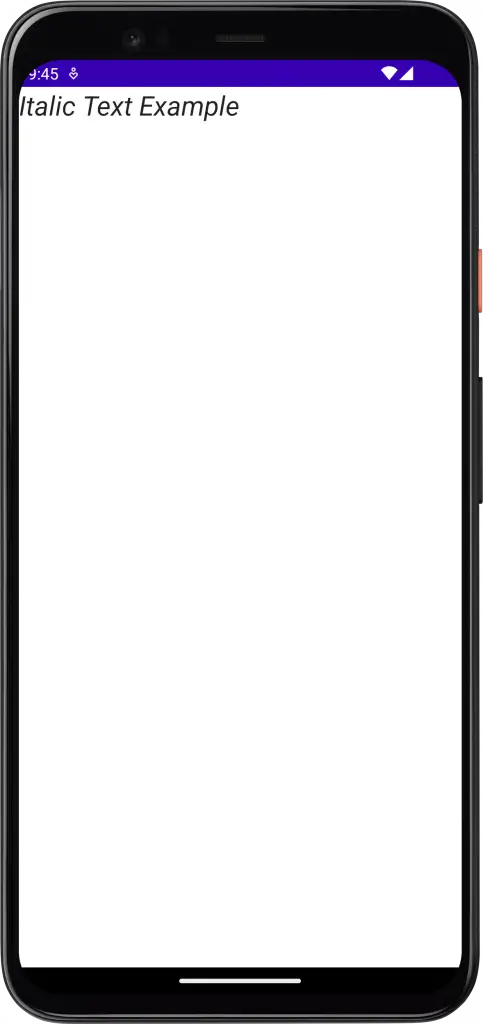
Following is the complete code for reference.
package com.codingwithrashid.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.text.font.FontStyle
import androidx.compose.ui.unit.sp
import com.codingwithrashid.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Column {
TextExample()
}
}
}
}
}
}
@Composable
fun TextExample() {
Text("Italic Text Example",
fontSize = 24.sp,
fontStyle = FontStyle.Italic
)
}
If you want to make text bold then see this blog post.
I hope this Jetpack Compose Text Italic tutorial is helpful for you.
2 Comments