How to Implement Empty Input Validation in Android Jetpack Compose
Ensuring that users fill in all required fields in a form is a crucial part of building a responsive and user-friendly app. In this blog post, we will go over how to perform empty input validation in Jetpack Compose, the modern toolkit for building native Android UI.
Here is a basic example of how you can implement empty input validation in Jetpack Compose:
@Composable
fun EmptyInputValidationExample() {
var text by remember { mutableStateOf("") }
var errorText by remember { mutableStateOf("") }
Column (modifier = Modifier.padding(16.dp)) {
OutlinedTextField(
value = text,
onValueChange = { text = it },
label = { Text("Input field") },
modifier = Modifier.fillMaxWidth(),
isError = errorText.isNotEmpty(),
singleLine = true
)
Text(
text = errorText,
color = Color.Red,
)
Button(
onClick = {
errorText = if (text.isBlank()) {
"Input field cannot be empty!"
} else {
""
// Continue with your processing here
}
},
modifier = Modifier.padding(top = 16.dp)
) {
Text("Submit")
}
}
}
In this example, we’re using OutlinedTextField for the input field. We also use the Button to submit the input. If the input is blank (either empty or consisting solely of whitespace), we set the errorText to a corresponding error message. If the input isn’t blank, we reset the errorText and continue with the processing (like moving to the next screen, making a network request, etc.)
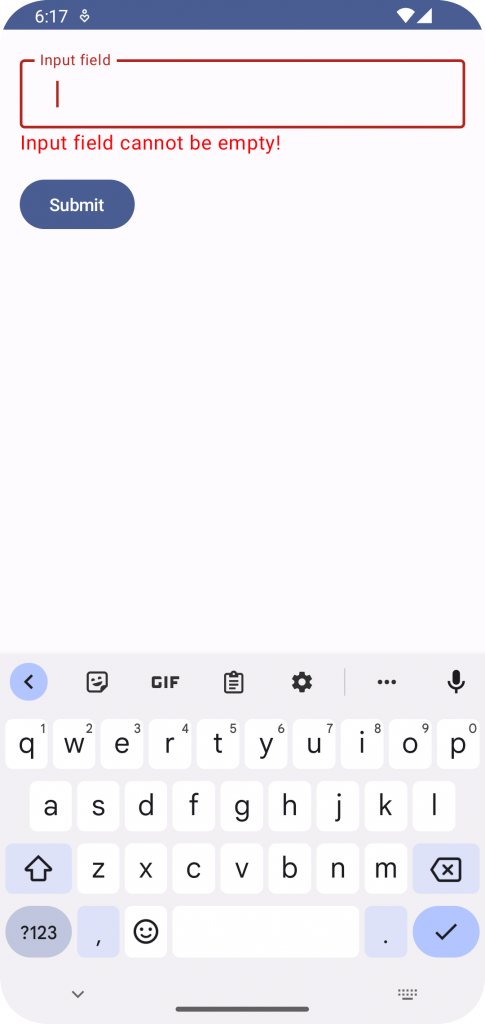
Difference Between isEmpty and isBlank in Kotlin
While validating input data in Kotlin, it is important to understand the difference between the isEmpty and isBlank methods. They are quite similar, but they handle whitespace differently:
isEmpty: This function checks if a string is empty or not. It returns true if the string length is 0. However, it treats spaces like any other character, so a string of just spaces would not be considered empty.
isBlank: This function checks if a string is blank or not. It returns true if the string length is 0 or consists solely of whitespace characters.
So in the context of input validation, if you want to ensure that the user does not just enter spaces and treat it as valid input, you should use isBlank. On the other hand, if you consider spaces as valid input, you should use isEmpty.
In our example, we used isBlank for the input validation as it covers both scenarios: when the user doesn’t enter anything and when the user only enters spaces. Both cases should not be valid input, hence isBlank is the right choice.