How to Implement Email Validation in Android Jetpack Compose
Android’s Jetpack Compose has been a game-changer for UI design, allowing developers to create interfaces with less code and more efficiency. In this blog post, we’ll cover how to perform email validation in Jetpack Compose.
Email validation is an important aspect of user input verification. Before storing or using user-provided emails, it’s good practice to validate them to ensure that they’re in the correct format.
We’ll start by creating a TextField which will allow the user to input their email address.
@Composable
fun EmailTextField(email: String, onEmailChange: (String) -> Unit) {
OutlinedTextField(
modifier = Modifier
.fillMaxWidth(),
value = email,
onValueChange = { onEmailChange(it) },
label = { Text("Email") },
singleLine = true,
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Email)
)
}
Next, we’ll write a function that validates if the entered text is a valid email address or not. We’ll use regex (regular expression) to check the email format.
fun isValidEmail(email: String): Boolean {
val emailRegex = "[a-zA-Z0-9._-]+@[a-z]+\\.+[a-z]+".toRegex()
return email.matches(emailRegex)
}
We now have our email validation function. The next step is to use this function to check the validity of the email entered by the user.
@Composable
fun ValidateEmail() {
var email by remember { mutableStateOf("") }
Column (modifier = Modifier.padding(16.dp)) {
EmailTextField(email = email, onEmailChange = { email = it })
if (email.isNotEmpty()) {
if (isValidEmail(email)) {
Text(text = "Email is valid", color = Color.Blue)
} else {
Text(text = "Email is not valid", color = Color.Red)
}
}
}
}
In the ValidateEmail composable, we first create a mutable state for the email. We then use the EmailTextField composable to display the text field and update the email state. If the user has entered something into the text field, we validate the email and show a message indicating whether the email is valid or not.
Following is the complete code.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.text.KeyboardOptions
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.OutlinedTextField
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.input.KeyboardType
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ValidateEmail()
}
}
}
}
}
@Composable
fun EmailTextField(email: String, onEmailChange: (String) -> Unit) {
OutlinedTextField(
modifier = Modifier
.fillMaxWidth(),
value = email,
onValueChange = { onEmailChange(it) },
label = { Text("Email") },
singleLine = true,
keyboardOptions = KeyboardOptions(keyboardType = KeyboardType.Email)
)
}
fun isValidEmail(email: String): Boolean {
val emailRegex = "[a-zA-Z0-9._-]+@[a-z]+\\.+[a-z]+".toRegex()
return email.matches(emailRegex)
}
@Composable
fun ValidateEmail() {
var email by remember { mutableStateOf("") }
Column (modifier = Modifier.padding(16.dp)) {
EmailTextField(email = email, onEmailChange = { email = it })
if (email.isNotEmpty()) {
if (isValidEmail(email)) {
Text(text = "Email is valid", color = Color.Blue)
} else {
Text(text = "Email is not valid", color = Color.Red)
}
}
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
ValidateEmail()
}
}
Following is the output.
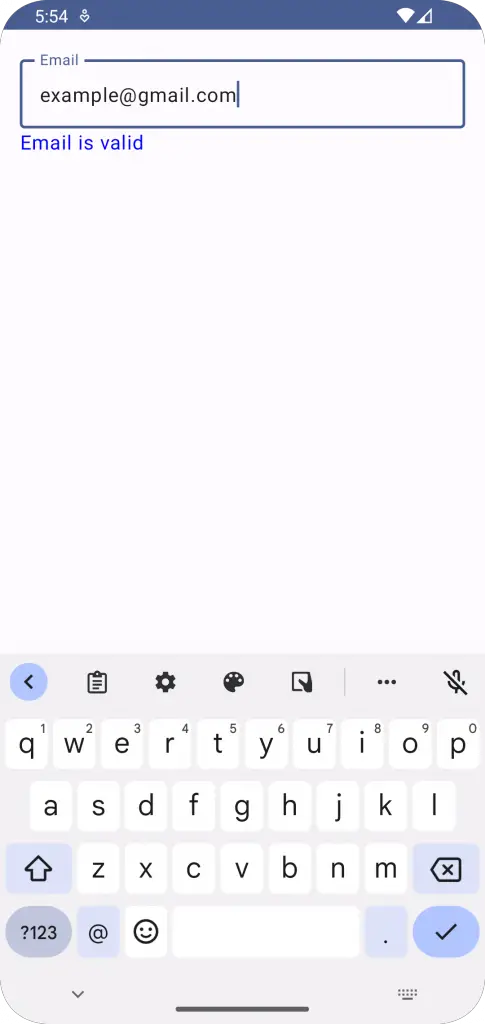
This simple guide showed you how to implement email validation in Jetpack Compose. This is a rudimentary form of validation, and for more sophisticated validation needs, you may want to consider using a form validation library or more complex regex patterns.