How to Create Slide In and Slide Out Animations in Android Jetpack Compose
Animations play an important role in enhancing the overall user experience in a mobile app. In this tutorial, let’s learn how to create a slide in animation as well as slide out animation in Jetpack Compose.
Jetpack Compose has some powerful APIs to create animations. In this tutorial, we use AnimatedVisibility composable to create slide in and slide out animations.
See the following code snippet.
var visibility by remember { mutableStateOf(false) }
AnimatedVisibility(
visible = visibility,
enter = slideIn(initialOffset = { IntOffset(100,100) }),
exit = slideOut(targetOffset = { IntOffset(-100,100) })
) {
Box(modifier = Modifier.size(100.dp).background(Color.Red))
}
We use the slideIn function to create slide in animation and the slideOut function to add slide out animation. The offset is added to control the direction of the animation.
Following is the complete code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.AnimatedVisibility
import androidx.compose.animation.slideIn
import androidx.compose.animation.slideOut
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.IntOffset
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
var visibility by remember { mutableStateOf(false) }
Column(Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally)
{
Button(onClick = { visibility = !visibility }) {
Text(text = "Click Here!")
}
AnimatedVisibility(
visible = visibility,
enter = slideIn(initialOffset = { IntOffset(100,100) }),
exit = slideOut(targetOffset = { IntOffset(-100,100) })
) {
Box(modifier = Modifier.size(100.dp).background(Color.Red))
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
The content of the activity is defined in the AnimationExample function. It uses the remember function to remember the state of the visibility of a composable component. The component is a button that changes the value of visibility on click.
The component that is being animated is a red box, which is wrapped in an AnimatedVisibility composable. The visibility of this component is controlled by the visibility state. The AnimatedVisibility composable animates the appearance and disappearance of its content using two animations: slideIn and slideOut.
The slideIn animation defines the animation that will be applied when the component appears on the screen, and slideOut defines the animation that will be applied when the component disappears from the screen.
Both animations take an initialOffset and targetOffset parameter, which specify the starting and ending positions of the animation.
Following is the output.
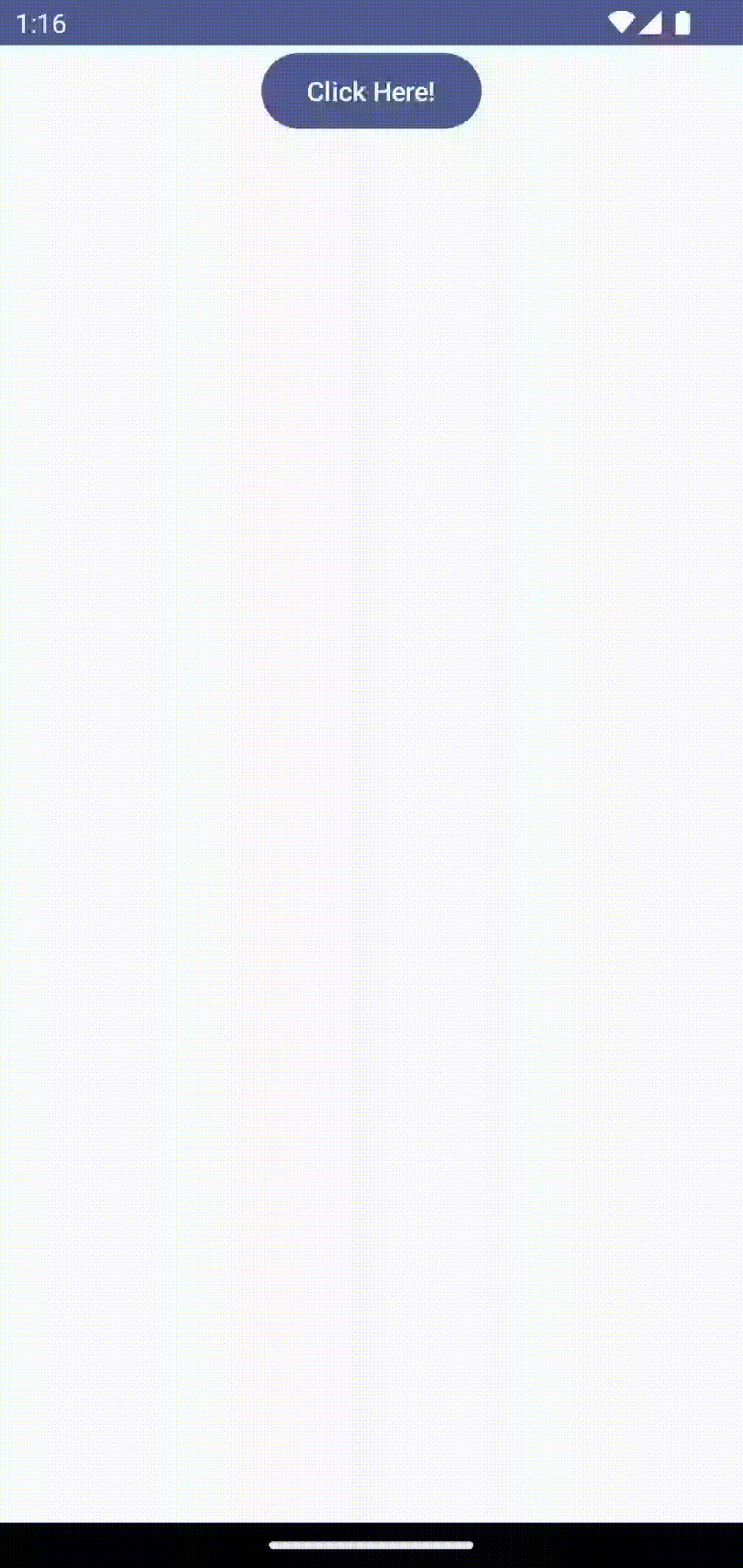
If you want more customization you can make use of AnimatedVisibility’s animationSpec parameter. See an example in the following code.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.animation.AnimatedVisibility
import androidx.compose.animation.core.LinearEasing
import androidx.compose.animation.core.tween
import androidx.compose.animation.slideIn
import androidx.compose.animation.slideOut
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material3.Button
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.IntOffset
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimationExample()
}
}
}
}
}
@Composable
fun AnimationExample() {
var visibility by remember { mutableStateOf(false) }
Column(Modifier.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally)
{
Button(onClick = { visibility = !visibility }) {
Text(text = "Click Here!")
}
AnimatedVisibility(
visible = visibility,
enter = slideIn(initialOffset = { IntOffset(100,100) },
animationSpec = tween(durationMillis = 3000, easing = LinearEasing)),
exit = slideOut(targetOffset = { IntOffset(-100,100) },
animationSpec = tween(durationMillis = 3000, easing = LinearEasing)),
) {
Box(modifier = Modifier.size(100.dp).background(Color.Red))
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
AnimationExample()
}
}
You can also use other slide animation related functions such as slideInHorizontally, slideInVertically, etc according to your needs.
For fade in and fade out animation, see this jetpack compose animation tutorial.