How to Use Conditional Modifier in Android Jetpack Compose
Jetpack Compose is revolutionizing the way we build user interfaces in Android. One of the key features of Jetpack Compose is the Modifier system. A Modifier is an ordered, immutable collection of decoration and layout settings that you can use to change the properties of Composable functions.
In this blog post, we’re going to explore the concept of applying modifiers conditionally – a powerful feature that enables dynamic UI updates based on certain conditions. We’ll explain the concept through detailed examples.
What are Conditional Modifiers
A conditional modifier in Jetpack Compose is a modifier that gets applied based on a certain condition. This provides flexibility when designing UIs, as the appearance and behavior of a Composable can be changed dynamically at runtime.
How to Implement Conditional Modifier
The implementation of conditional modifiers involves evaluating certain conditions and applying modifiers accordingly. The conditions could be anything from the state of a variable to the result of a complex calculation.
Let’s look at some examples:
Example 1: Conditionally Apply Background
In this example, let’s consider a scenario where we need to change the background color of a Box based on whether a button has been clicked.
@Composable
fun ModifierExample() {
var isClicked by remember { mutableStateOf(false) }
Column {
Button(onClick = { isClicked = !isClicked }) {
Text("Click me")
}
Box(
modifier = Modifier
.background(if (isClicked) Color.Green else Color.Red)
.size(100.dp)
)
}
}
In the above example, when the button is clicked, the state of isClicked changes and the Box changes its background color accordingly.
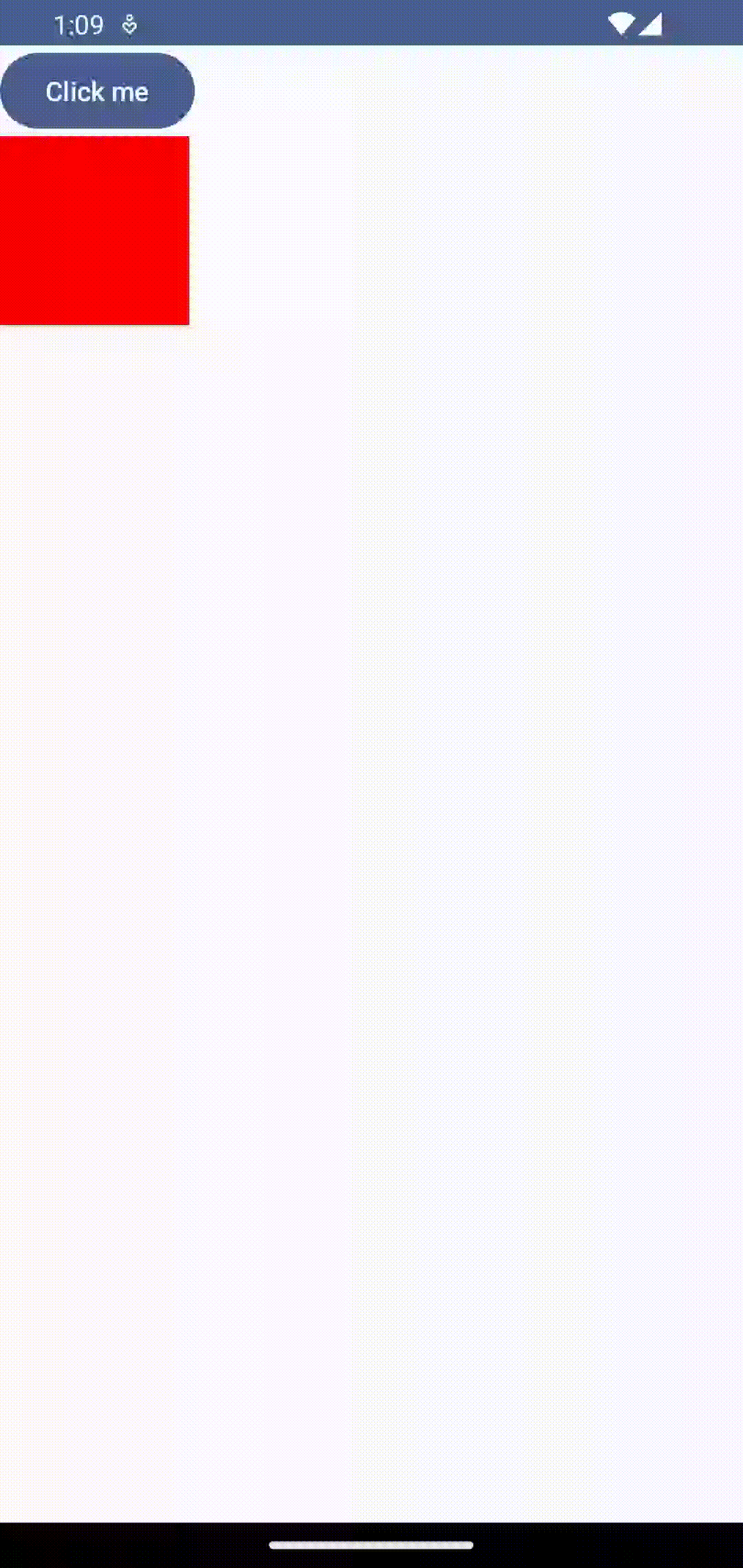
Example 2: Conditionally Apply Padding
Let’s consider a scenario where we need to adjust the padding of a Text Composable based on the length of the text:
@Composable
fun ModifierExample() {
val text = remember { mutableStateOf("Hello") }
Column {
TextField(
value = text.value,
onValueChange = { text.value = it },
label = { Text("Enter text") }
)
Text(
text = text.value,
modifier = Modifier.padding(if (text.value.length > 5) 20.dp else 10.dp)
)
}
}
In this example, the padding of the Text changes based on the length of the text in the TextField. If the length of the text is more than 5, the padding is set to 20.dp; otherwise, it’s set to 10.dp.
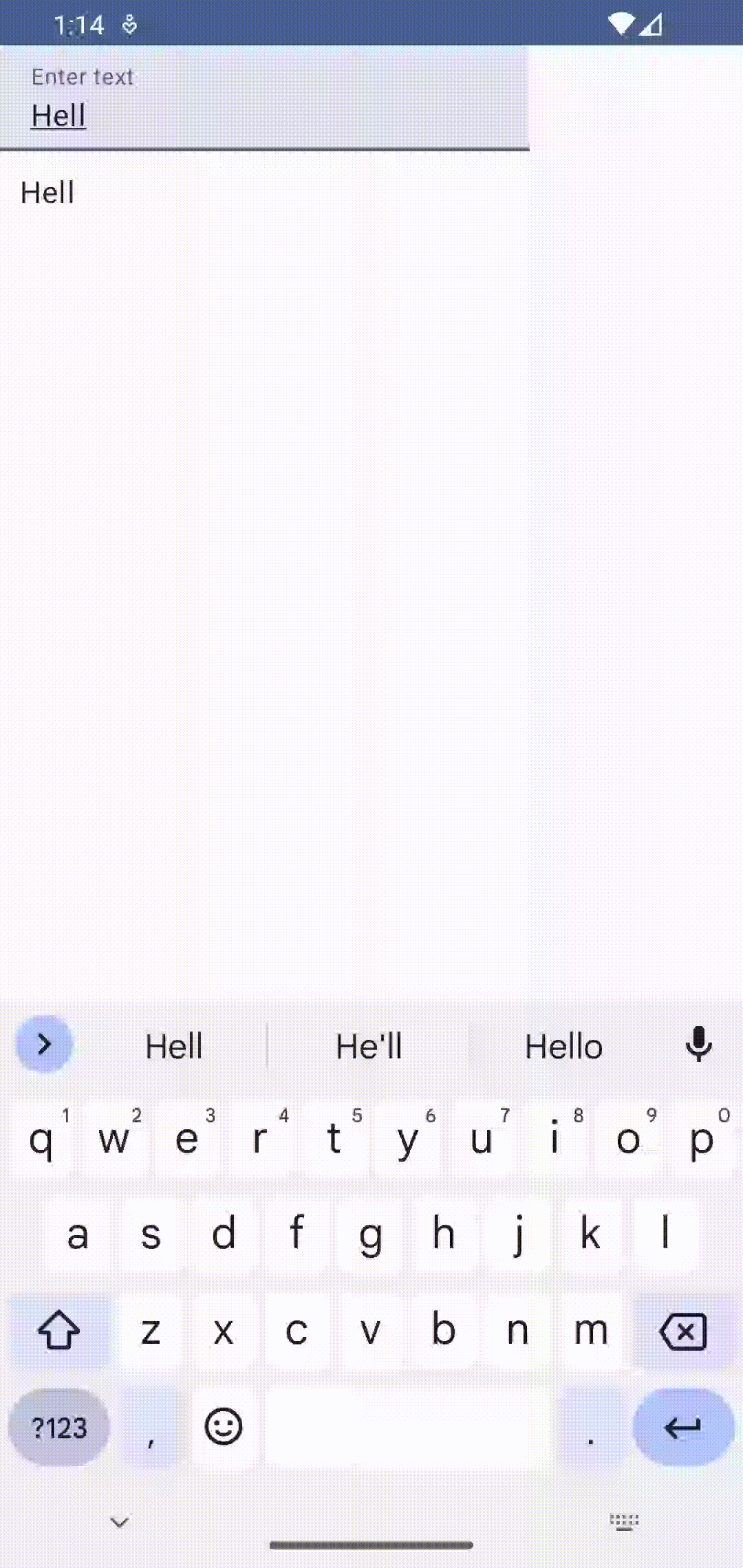
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.material3.TextField
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ModifierExample()
}
}
}
}
}
@Composable
fun ModifierExample() {
val text = remember { mutableStateOf("Hello") }
Column {
TextField(
value = text.value,
onValueChange = { text.value = it },
label = { Text("Enter text") }
)
Text(
text = text.value,
modifier = Modifier.padding(if (text.value.length > 5) 20.dp else 10.dp)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
ModifierExample()
}
}
Conditional modifiers in Jetpack Compose are a powerful tool to create dynamic and interactive user interfaces. By understanding and implementing them effectively, you can make your app’s UI respond to user interactions and state changes, creating a rich and engaging user experience.