How to Center Align Text in Android Jetpack Compose
Text is one of the most essential UI elements in mobile app development, and properly aligning it can make a significant difference in the overall user experience. In this tutorial, we will learn how to center align text in Jetpack Compose.
Jetpack Compose is a modern UI toolkit for Android that simplifies UI development with a declarative approach. With Compose, you can create beautiful and responsive UIs with less code.
The Text composable is used to display text content in Jetpack compose. The TextAlign class helps to center align Text in compose.
See the code snippet given below.
@Composable
fun CenterAlignedText() {
Box(modifier = Modifier.fillMaxSize(),
contentAlignment = Alignment.Center) {
Text(text = "Center Align Text Example",
textAlign = TextAlign.Center)
}
}
In the above code, we first create a Box composable and set its modifier to fill the entire screen using Modifier.fillMaxSize(). The contentAlignment parameter is set to Alignment.Center, which will center the Text composable inside the Box.
Finally, we create a Text composable with the text “Center Align Text Example” and set its textAlign parameter to TextAlign.Center to center align the text.
Following is the output.
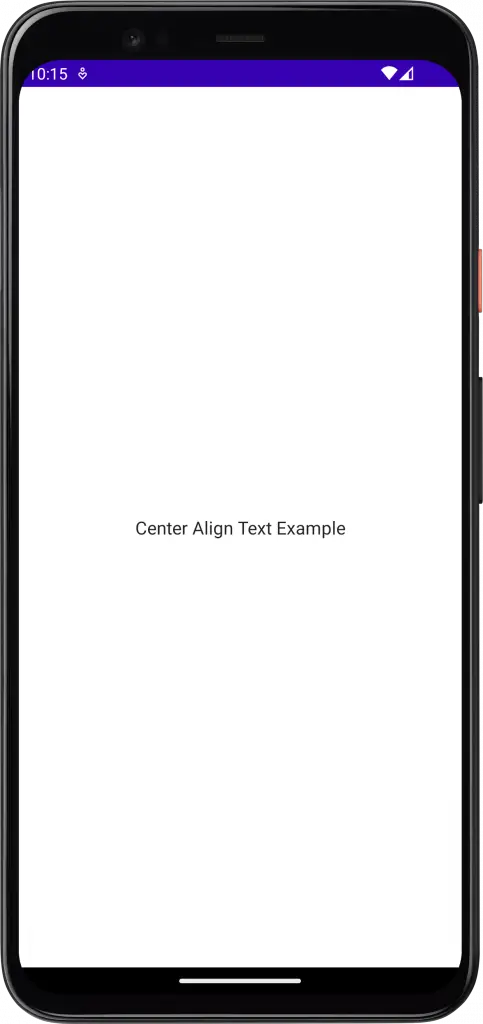
Following is the complete code for reference.
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.*
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
CenterAlignedText()
}
}
}
}
}
@Composable
fun CenterAlignedText() {
Box(modifier = Modifier.fillMaxSize(),
contentAlignment = Alignment.Center) {
Text(text = "Center Align Text Example",
textAlign = TextAlign.Center)
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyApplicationTheme {
CenterAlignedText()
}
}
In this tutorial, we learned how to center align text in Android Jetpack Compose. We used the Box and Text composables to create a simple example that centers the text on the screen.