Importance of Modifier Order in Android Jetpack Compose
In the world of Jetpack Compose, Modifiers are pivotal in designing and structuring our UI elements. They allow us to apply alterations to our composables, such as padding, background color, click events, and so much more.
But did you know that the order in which you apply these modifiers can drastically affect the final output of your UI? This might sound a bit confusing, so in this blog post, we will unpack the intricacies of modifier order and illustrate its significance with multiple examples.
In Jetpack Compose, the sequence in which modifiers are applied to a composable is crucial. This is because modifiers are read from left to right, and each one operates on the result of the one before it. Changing the order of modifiers could potentially lead to a completely different result.
Example 1: Padding and Background
Consider a composable that has a padding and a background modifier.
@Composable
fun ModifierExample() {
Column() {
Box(
modifier = Modifier
.padding(10.dp)
.background(Color.Red)
.size(100.dp)
)
}
}
In the above example, the padding modifier is applied first. This means that the padding is added to the Box, and then the background color is applied. The result is a 100×100 dp box with a red background and a 10 dp padding around it.
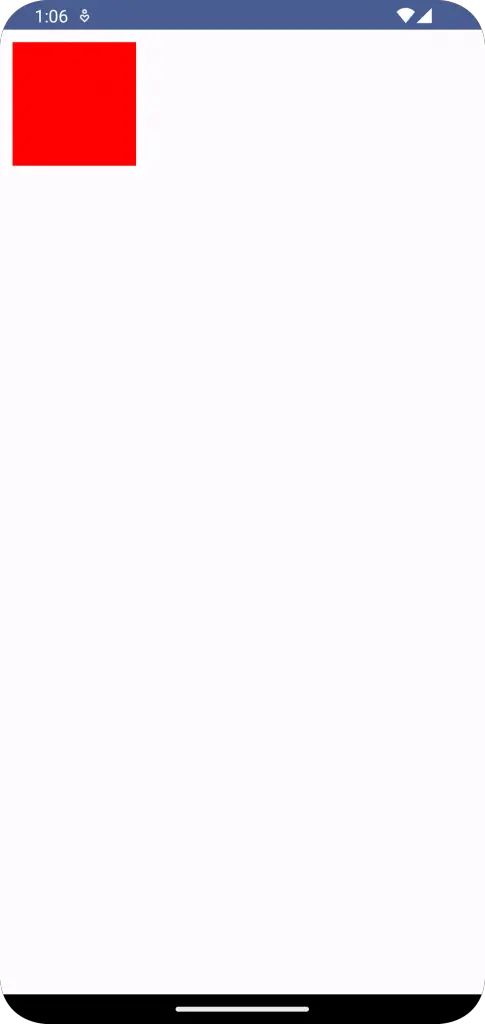
Now, let’s switch the order of the padding and background modifiers.
@Composable
fun ModifierExample() {
Column() {
Box(
modifier = Modifier
.background(Color.Red)
.padding(10.dp)
.size(100.dp)
)
}
}
In this case, the background color is applied first and then the padding. The end result is quite different: the padding is now part of the red background. Essentially, you have a larger red box of size 120×120 dp, with the actual box size still being 100×100 dp.
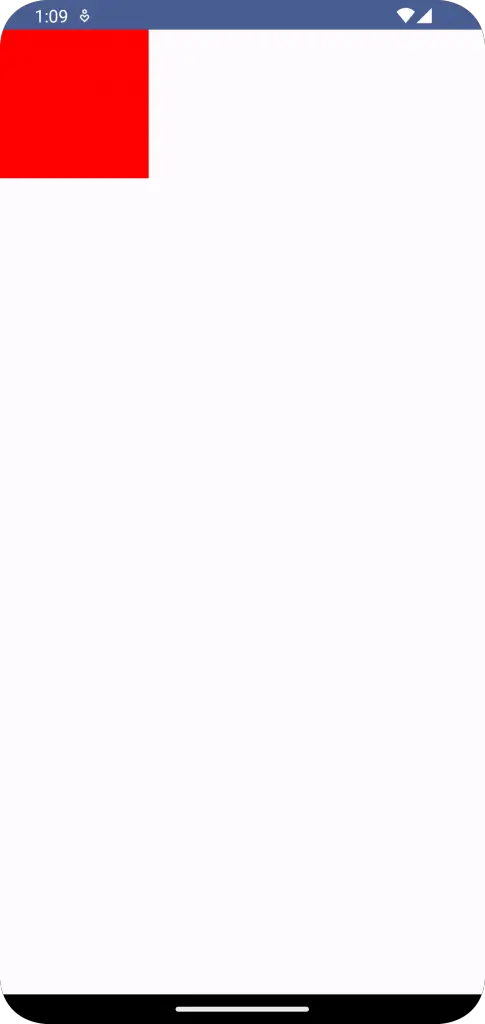
Example 2: Border and Padding
Consider a composable that has a border and padding modifier.
@Composable
fun ModifierExample() {
Column() {
Box(
modifier = Modifier
.padding(10.dp)
.border(2.dp, Color.Red)
.size(100.dp)
)
}
}
In this case, we first apply the padding modifier, then we apply a red border. The end result will be a 100×100 dp box with a 10 dp padding and a red border outside of the padding. This means that the border is drawn around the padding.
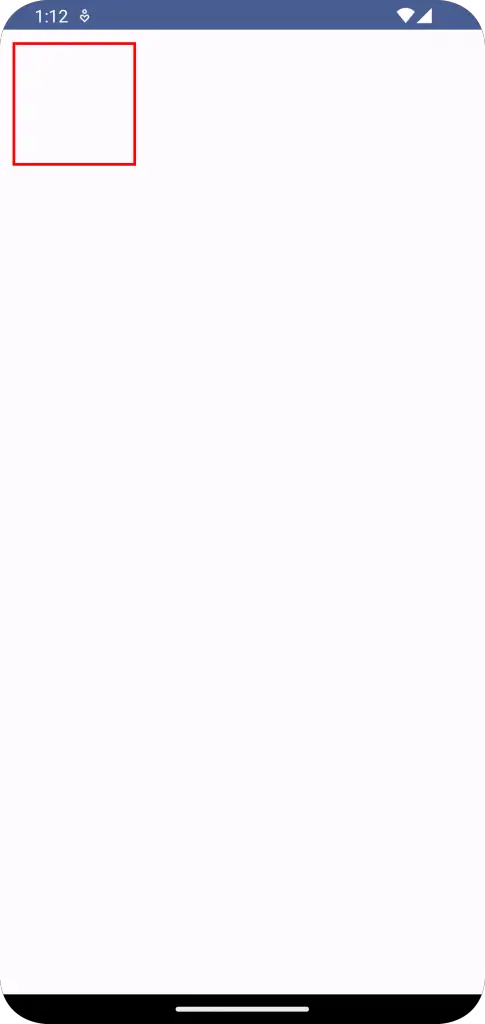
Now, let’s switch the order of the padding and border modifiers.
@Composable
fun ModifierExample() {
Column() {
Box(
modifier = Modifier
.border(2.dp, Color.Red)
.padding(10.dp)
.size(100.dp)
)
}
}
Here, we first apply the border and then the padding. So the border is now drawn around the box without padding, and the padding is then added inside the box. This means the border is closer to the box compared to the previous example.
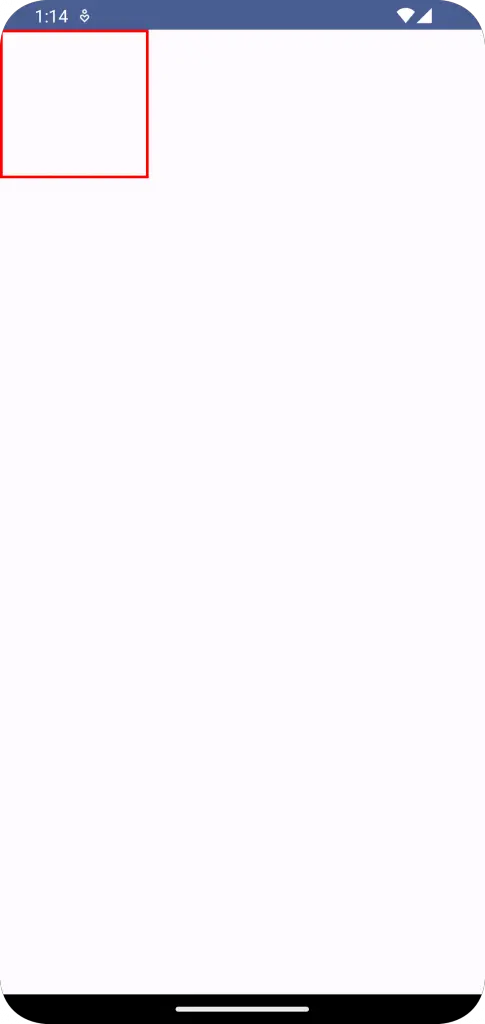
Example 4: Clip and Background
Another interesting pair of modifiers to consider is clip and background.
@Composable
fun ModifierExample() {
Column() {
Box(
modifier = Modifier
.clip(RoundedCornerShape(10.dp))
.background(Color.Red)
.size(100.dp)
)
}
}
In this example, we first clip the corners of the box and then apply a red background. This results in a box with rounded corners filled with a red color.
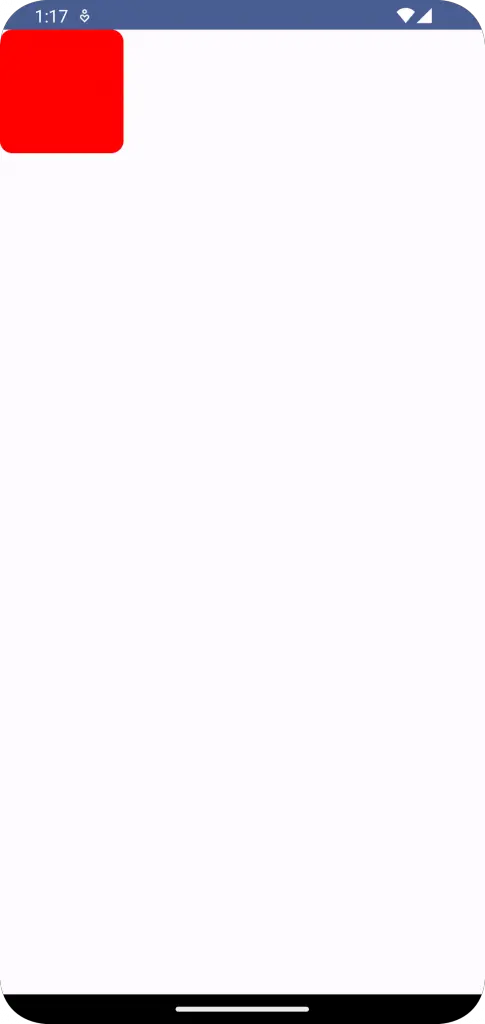
Now, let’s switch the order.
@Composable
fun ModifierExample() {
Column() {
Box(
modifier = Modifier
.background(Color.Red)
.clip(RoundedCornerShape(10.dp))
.size(100.dp)
)
}
}
Here, we first fill the box with red color and then clip the corners. This also results in a box with rounded corners filled with red color, but it differs from the first one in terms of performance.
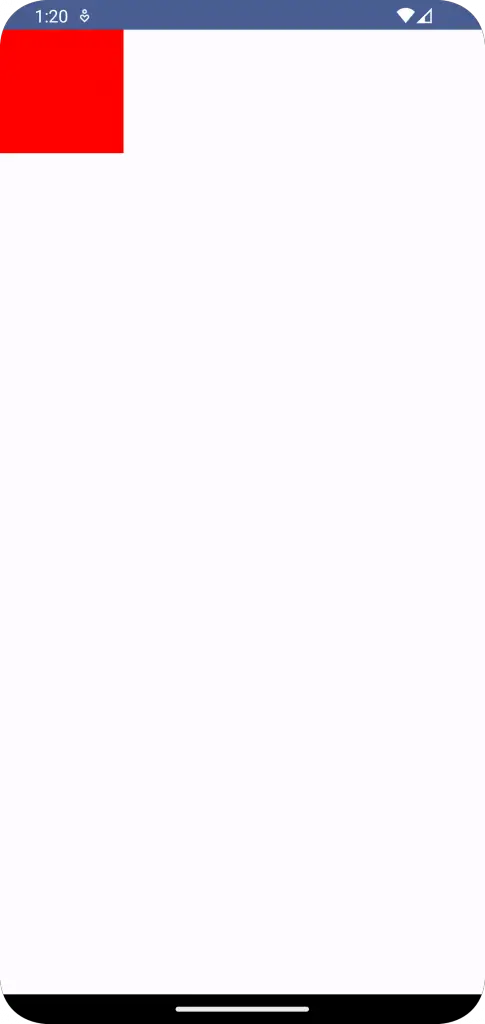
Understanding the importance of modifier order in Jetpack Compose can greatly impact the final output of your UI. Experimenting with different orders can help you achieve the desired results while avoiding any unexpected behaviors.
The key takeaway is that the order matters! Remember to consider modifier order whenever you’re building out your Jetpack Compose UI.