How to Use Alpha Modifier in Android Jetpack Compose
n Android UI development, sometimes we need to adjust the visibility or opacity of a UI component to provide a better user experience or to create a certain visual effect. This is where the alpha property comes in.
With Jetpack Compose, this can be achieved easily using the alpha modifier. In this blog post, we will delve into how the alpha modifier works in Jetpack Compose and illustrate it with examples.
The alpha modifier is used to adjust the opacity of a UI element. The value of alpha ranges from 0f to 1f. An alpha value of 0f makes the UI component completely transparent (invisible), while a value of 1f makes it completely opaque (visible).
How to Implement Alpha in Jetpack Compose
Jetpack Compose provides the alpha function as a modifier. This function takes a float value representing the desired opacity.
Let’s create a simple example where we have a Box with a color and apply the alpha modifier to it.
@Composable
fun ModifierExample() {
Column() {
Box(
modifier = Modifier
.size(100.dp)
.alpha(0.5f)
.background(Color.Red)
)
}
}
In the example above, the Box composable has a red background and an alpha of 0.5, making it semi-transparent.
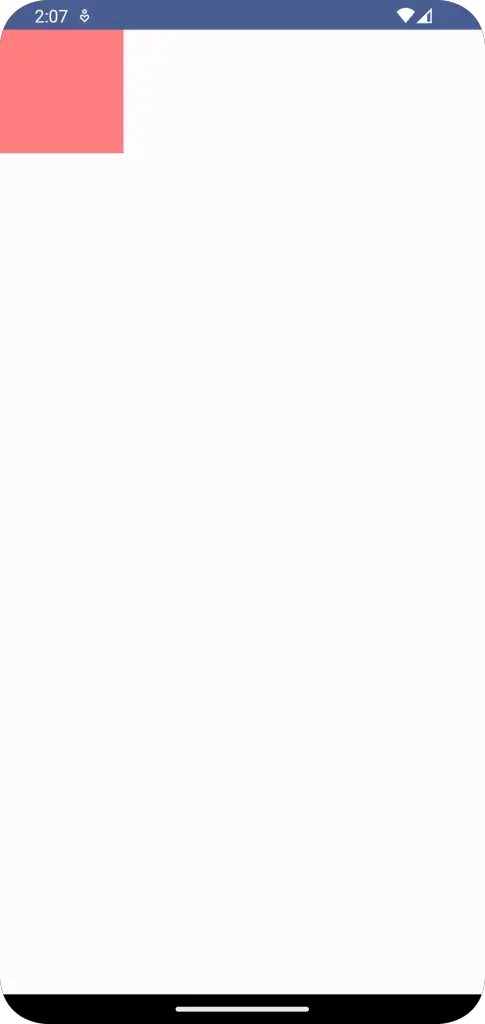
How to Add Interaction with Alpha
We can make our UI more interactive by changing the alpha in response to user interaction.
@Composable
fun ModifierExample() {
var alpha by remember { mutableStateOf(1f) }
Column() {
Slider(
value = alpha,
onValueChange = { alpha = it },
valueRange = 0f..1f
)
Box(
modifier = Modifier
.size(100.dp)
.alpha(alpha)
.background(Color.Red)
)
}
}
In this example, we have a Slider that adjusts the alpha value of the Box. As you move the slider, the Box becomes more transparent or opaque.
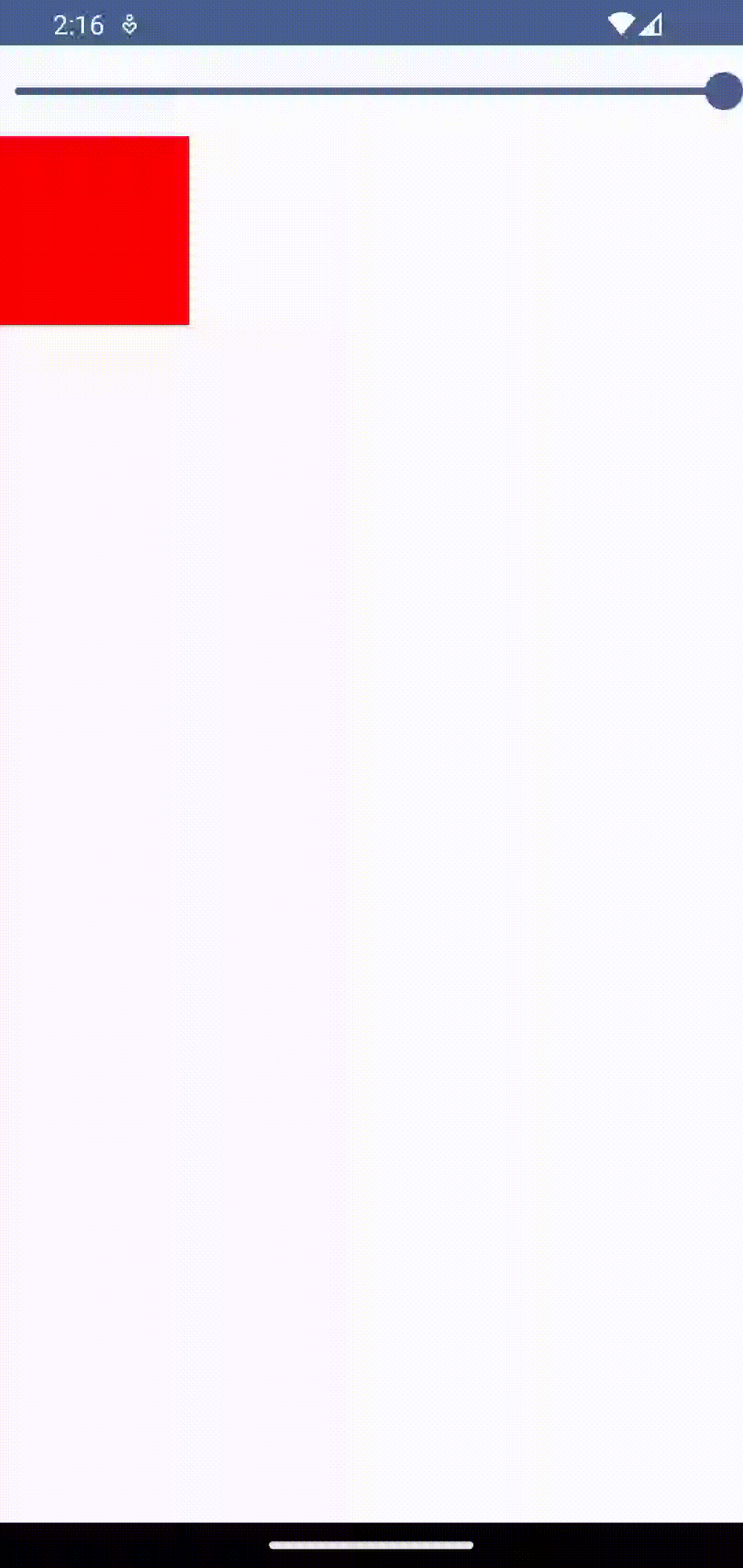
Following is the complete code for reference.
package com.example.example
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.size
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Slider
import androidx.compose.material3.Surface
import androidx.compose.runtime.Composable
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.runtime.setValue
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.alpha
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.example.ui.theme.ExampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ExampleTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
ModifierExample()
}
}
}
}
}
@Composable
fun ModifierExample() {
var alpha by remember { mutableStateOf(1f) }
Column() {
Slider(
value = alpha,
onValueChange = { alpha = it },
valueRange = 0f..1f
)
Box(
modifier = Modifier
.size(100.dp)
.alpha(alpha)
.background(Color.Red)
)
}
}
@Preview(showBackground = true)
@Composable
fun ExamplePreview() {
ExampleTheme {
ModifierExample()
}
}
The alpha modifier in Jetpack Compose allows you to adjust the opacity of a UI component, enabling you to create different visual effects and enhance the user interaction. With the simplicity of Jetpack Compose, adjusting the alpha of a composable becomes a straightforward task.